Pass data from Spring controller to JSP
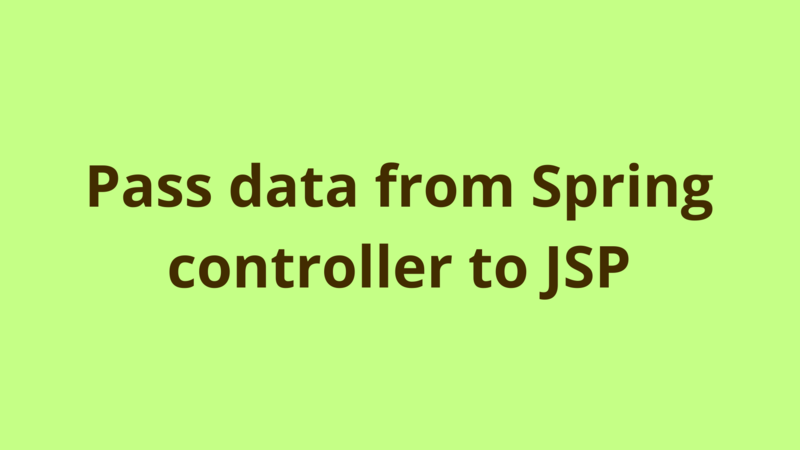
ADVERTISEMENT
Table of Contents
Introduction
This tutorial describes how to pass data from a Spring controller to a JSP view.
If you’re still using servlets, then check our “Pass data from servlet to JSP” tutorial.
Spring MVC provides a very convenient way of doing this by exposing objects like ModelMap and ModelAndView.
1- ModelMap
Spring MVC exposes a utility class called ModelMap which implicitly extends a LinkedHashMap.
In order to pass data from controller to JSP, all you have to do is add a ModelMap argument to your controller method and then populate it inside your method body using the generic addAttribute() method.
In the following example, we pass a welcome message from the controller by adding a message attribute to the model.
@RequestMapping("/")
public String welcome(ModelMap model) {
model.addAttribute("message", "Programmer Gate");
return "/home";
}
You can use addAttribute() to pass any type of data like objects, arrays, lists and maps.
When using ModelMap, you have to explicitly return the name of the JSP view at the end of your controller method.
Now, inside your JSP, you can read the message attribute in 2 ways:
<h1>Welcome to ${message} </h1>
<h1>Welcome to <= request.getAttribute("message")%> </h1>
2- ModelAndView
ModelAndView interface is used to pass data attributes and JSP view name at one shot, unlike ModelMap which is only used for passing attributes.
When using ModelAndView, your controller method should return a ModelAndView object instead of a String. Here below, we write the same above example using ModelAndView:
@RequestMapping("/")
public ModelAndView welcome() {
ModelAndView model = new ModelAndView("/home");
model.addObject("message", this.message);
return model;
}
As noticed, we pass the view name as an argument to the ModelAndView constructor, then we pass data attributes through the addObject() method.
ModelAndView interface is not defined as an argument to the controller method, rather it’s instantiated inside the method body.
Summary
This tutorial describes how to pass data from a Spring controller to a JSP view.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers