Pass data from html to servlet
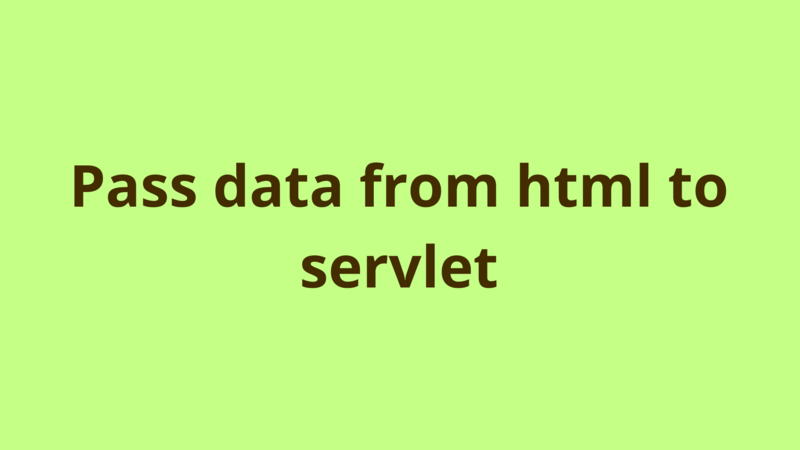
ADVERTISEMENT
Table of Contents
Introduction
In this tutorial we explain the common way of passing data from html to java servlets, we mostly focus on passing form data entered by end-users through pages like: login, sign-up, contact-us ..
- Pass form fields to servlet Consider the following login.html:
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Login Page</title>
</head>
<body>
<form name="loginForm" method="GET" action="LoginProcessor">
Username: <input type="text" name="username"/> <br/>
Password: <input type="password" name="password"/> <br/>
<input type="submit" value="Login" />
</form>
</body>
</html>
Users interact with web applications through forms, the “form” element is the common way for asking end-users to add their input. Upon submission, the user’s input is traversed to the server side for processing through 2 important attributes method & action supported by the form element:
- action: this attribute specifies the relative url of the servlet which handles the form submission, in our example, we should have defined a servlet mapped by “/LoginProcessor” in our application.
- method: this attribute defines the submit method, there are 2 ways of passing form data from client side to server side:
- GET method: using this method, the form fields are passed through URL (as QueryString), they are visible to the end-user and are limited in terms of length: maximum 2083 characters, this method is only used when submitting ASCII characters, it is not efficient when submitting binary data like: files.
- POST method: using this method, the form fields are passed through the body of the http request, they aren’t visible to the end-user (i.e. more secured), the amount of transmitted data is unlimited and can support binary data type. This method is mostly recommended over the GET method.
When submitting a form, the application container reads the method & action attributes, calls the appropriate handler, and passes all the form fields using the specified method as: name=value format.
In our example, since we’re using GET method, the form fields are passed to server side through the URL as the following:
http://localhost:8085/PassHtmlServlet/LoginProcessor?username=Hussein&password=Terek
However, if we were using POST method, the browser URL would look like the following after submission:
http://localhost:8085/PassHtmlServlet/LoginProcessor
and the form fields would have passed inside the body of the http request.
In both methods, the form fields will reach the server side in: name=value format.
2. Read form fields inside servlet
In order to process the login request submitted by login.html, we define the following servlet:
/**
* Servlet implementation class Processor
*/
@WebServlet("/LoginProcessor")
public class LoginProcessor extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
}
}
Notice that the value of @WebServlet(“/LoginProcessor”) is similar to the value of the action attribute defined in login.html.
At the server side, requests are handled through 2 methods: doPost(), doGet().
- doPost(): this method handles requests submitted through POST method.
- doGet(): this method handles requests submitted through GET method.
In our example, when user submits login.html, the request is handled in doGet() as we defined the method as GET.
In order to read the submitted form data, HttpServletRequest supports the following methods which can be used in both doGet() & doPost():
- String getParameter(String name): this method retrieves the value of a specific field through the field name, it should be used with single-value form fields like: textbox, password, textarea, radio button, combobox …
- In our example, in order to read the submitted username and password, we can use the following inside doGet() method:
String username = request.getParameter("username");
String password = request.getParameter("password");
- String[] getParameterValues(String name): this method retrieves the multiple values of a specific field through the field name, it should be used with multi-value form fields like: checkbox i.e. retrieve all the selected values of a checkbox group.
- For example, suppose we want to read all the selected languages of a “language” checkbox, we would write the following at the server side:
String languages[] = request.getParameterValues("language");
if (languages != null) {
System.out.println("Languages are: ");
for (String lang : languages) {
System.out.println("\t" + lang);
}
}
- Enumeration
getParamterNames() : this method retrieves the names of the complete list of all parameters in the current request, it is mostly used when you have many fields in the request and you don’t know the name of all fields.- For example, we would read the values of all form fields without knowing any field name through the following:
Enumeration paramNames = request.getParameterNames();
while(paramNames.hasMoreElements()) {
String paramName = (String)paramNames.nextElement();
String[] paramValues = request.getParameterValues(paramName);
// Read single valued data
if (paramValues.length == 1) {
String paramValue = paramValues[0];
} else {
// Read multiple valued data
for(int i = 0; i < paramValues.length; i++) {
}
}
}
Summary
In this tutorial we explain the common way of passing data from html to java servlets, we mostly focus on passing form data entered by end-users through pages like: login, sign-up, contact-us ..
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers