Pow() Function in Java and Python
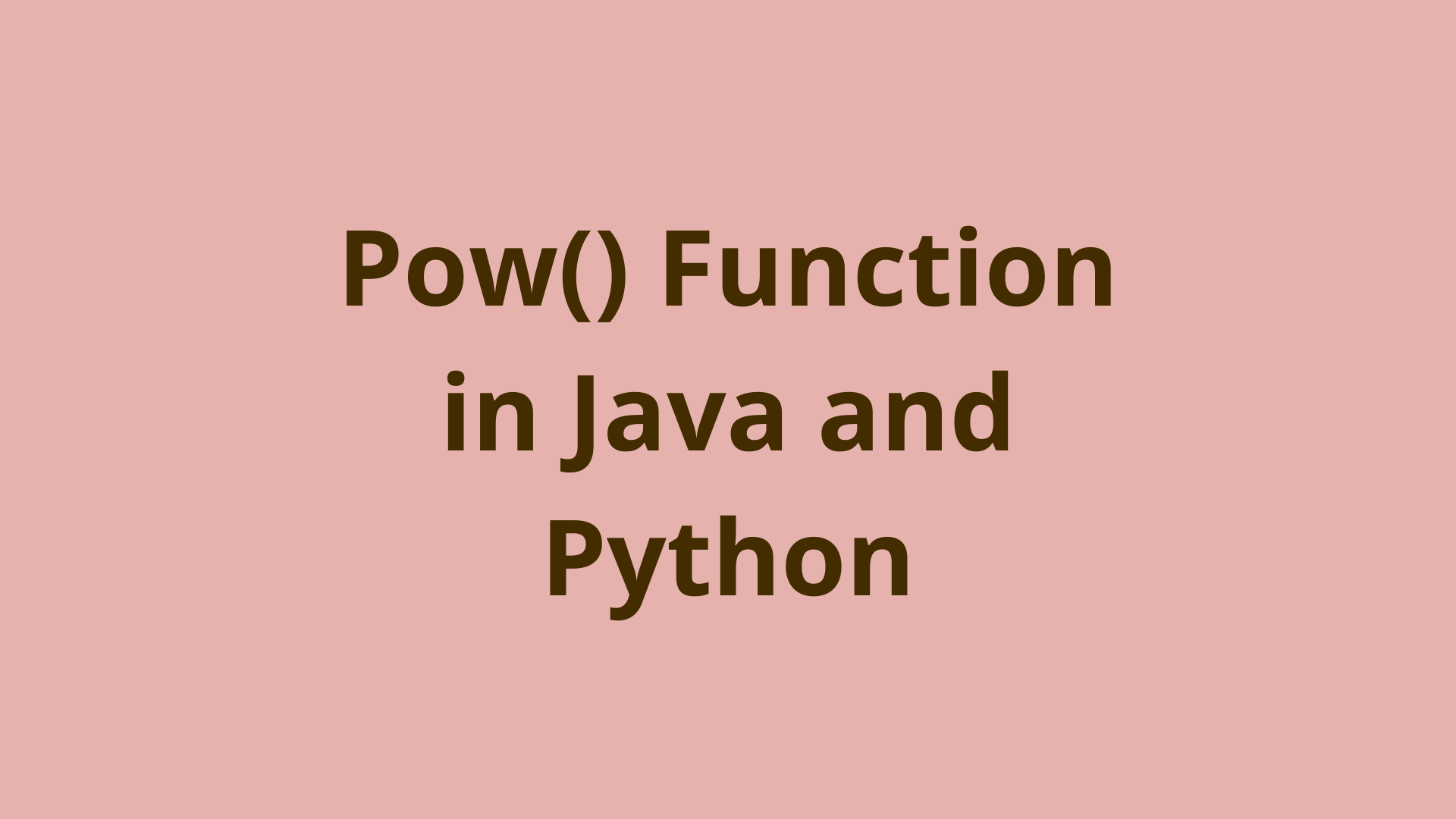
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of the Pow() Function
- How the Pow() Function Works
- Example: Pow() with a Negative Exponent
- Example: Pow() with a Negative Base
- Example: Pow() with a Fractional Base
- Summary
- Next Steps
Introduction
Java and Python both come with a library of math functions that allow users to perform a variety of mathematical operations. One useful function is pow()
which can be found in the java.lang.Math
package in Java and the math
module in Python. In this article, we'll describe how to use this function in both cases.
Overview of the Pow() Function
The pow()
function calculates the value of a base raised to the power of its exponent. This calculation is the equivalent of multiplying the base by itself the same number of times as its exponent value. For example, when using the pow() function with a base argument of 3 and an exponent argument of 4, the Function would return the value of 34, or 3*3*3*3.
How the Pow() Function Works
We'll start by explaining how to use this function in both Java and Python. In Java, the Math.pow()
function takes exactly two arguments, a base and an exponent. This function is overloaded to provide support for a variety of numerical object types, including floats, doubles, and integers. The base and the exponent can also be different numerical types.
For this first example, we'll implement some code that calculates the raised value of an integer base to an integer exponent:
import java.lang.Math;
public class PowerCalculator{
public static void main(String []args){
int base = 3;
int exponent = 4;
double raised_value = Math.pow(base, exponent);
System.out.println(raised_value);
}
}
When we run this code, we'll see that, as expected, it prints out the value of 34, 81. By implementing a for
loop instead, we can confirm that multiplying 3 by itself 4 times results in the same value:
import java.lang.Math;
public class PowerCalculator{
public static void main(String []args){
double base = 3;
double exponent = 4;
double raised_value = 1;
for (int i=0; i < exponent; i++)
{
raised_value = raised_value * base;
}
System.out.println(raised_value);
}
}
The syntax for the pow() function in Python is very similar to the Java syntax. Again, the Python function has two required arguments, a base and an exponent. It also provides optional support for a third argument, a modulus value. Because Python supports implicit typecasting, we don't need to worry about defining the variable types as we did in Java. Instead, the pow() function accepts any numerical value as an argument.
Here, we'll write a similar script in Python to calculate the value of 34:
import math
base = 3
exponent = 4
raised_value = math.pow(base, exponent)
print(raised_value)
As with the Java example, this script will print out the value of 34, 81. We can confirm again that multiplying 3 by itself 4 times results in the same value:
import math
base = 3
exponent = 4
raised_value = 1
for i in range(exponent):
raised_value = raised_value * base
print(raised_value)
Example: Pow() with a Negative Exponent
The pow() function isn't limited to working with positive values. To demonstrate this, we'll calculate the value of a base raised to a negative exponent. This is the mathematical equivalent of inversing the base so that is now the denominator of a fraction and then raising that fraction to a positive exponent. For example, 3-4 is the equivalent of 1/34.
We can implement this similarly to the previous example by just changing the exponent value to -4:
import java.lang.Math;
public class PowerCalculator{
public static void main(String []args){
int base = 3;
int exponent = -4;
double raised_value = Math.pow(base, exponent);
System.out.println(raised_value);
}
}
When we run this code, it prints 0.012345679012345678
, the correct value of 3-4. When we implement a similar example in Python, it prints the same value:
import math
base = 3
exponent = -4
raised_value = math.pow(base, exponent)
print(raised_value)
Example: Pow() with a Negative Base
Now, what would happen if we tried to work with a negative base? Since a negative value multiplied by a negative value results in a positive value, any base with an even exponent should return a positive result. Conversely, since a positive value multiplied by a negative value results in a negative value, any base with an odd exponent should return a negative exponent.
Let's demonstrate this by implementing -34 in Java. Again, we can simply change the base value to -3 and the exponent value to 4:
import java.lang.Math;
public class PowerCalculator{
public static void main(String []args){
int base = -3;
int exponent = 4;
double raised_value = Math.pow(base, exponent);
System.out.println(raised_value);
}
}
As expected, when we run this code, we'll see that it prints out a positive value, 81. Conversely, let's try an example in Python where the exponent is odd, -33:
import math
base = -3
exponent = 3
raised_value = math.pow(base, exponent)
print(raised_value)
In this case, this script will print out a negative value, specifically -27.
Example: Pow() with a Fractional Base
The pow()
function is also not limited to working with integers. In this example, we'll calculate the value of a double base raised to an integer exponent. To make things more interesting, we'll make that double a fractional value of 0.25. Mathematically, this is identical to working with an integer base, with 0.253 being equal to 0.25*0.25*0.25. Since 0.25 is less than 1, however, the resulting product will be less than the base value.
Let's implement this in Java by setting the base value to 0.25 and the exponent to 3:
import java.lang.Math;
public class PowerCalculator{
public static void main(String []args){
double base = 0.25;
int exponent = 3;
double raised_value = Math.pow(base, exponent);
System.out.println(raised_value);
}
}
When we execute this code, we'll see that it prints out the value, 0.015625
. When we execute a similar script in Python, we'll see we get the same result:
import math
base = 0.25
exponent = 3
raised_value = math.pow(base, exponent)
print(raised_value)
Example: Pow() with a Fractional Exponent
The pow() function also supports fractional exponents. In this example, we'll find the value of an integer base raised to a double exponent. Again, we'll make that double a fractional value of 0.25. Mathematically, 30.25 is the equivalent of finding the fourth root of 3. The fourth root is the value that, when multiplied by itself 4 times, results in the base value.
As with the previous example, let's implement this in Java by setting the base value to 3 and the exponent to 0.25:
import java.lang.Math;
public class PowerCalculator{
public static void main(String []args){
int base = 3;
double exponent = 0.25;
double raised_value = Math.pow(base, exponent);
System.out.println(raised_value);
}
}
When we run this code, we'll see that it prints out the value of 30.25, 1.3160740129524924
. When we execute an analogous script in Python, we get the same result:
import math
base = 3
exponent = 0.25
raised_value = math.pow(base, exponent)
print(raised_value)
Summary
In this article, we explored the Java and Python pow()
functions to understand how each one works. We discussed what parameters they take, the data types they support, and the math behind the values they return. Java's pow() function can be found in the java.lang.Math
library and Python's can be found in the math
module. In both libraries, the pow() function is the ideal way to calculate the value of base raised to a power.
Next Steps
If you're interested in learning more about the basics of Python, Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers