Python all() Function
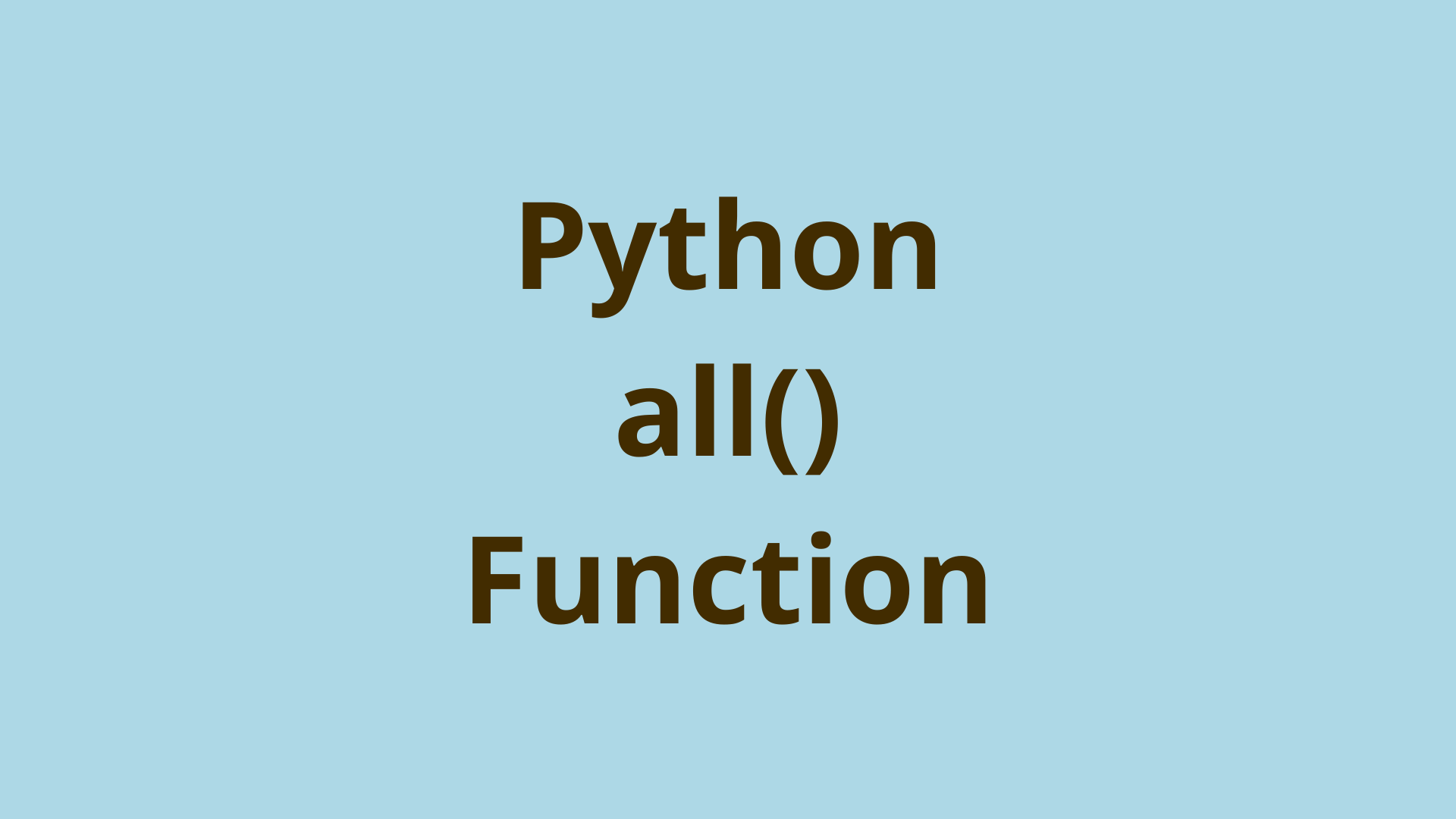
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of the All function in Python
- What are True and False values in Python?
- All() function with Tuple and Set
- All function with Dictionary
- All function with individual Strings and Integers
- all() vs any()
- Understanding All function with for loop
- Summary
- Next Steps
Introduction
Python has a vast array of built-in functions that help us quickly implement an algorithm, that would otherwise take much more time to create ourselves. The accessibility of these functions is part of what makes Python such a powerful programming language.
In this article, we will explore the all()
Python function and look at how it handles various data structures and data types.
Overview of the All function in Python
The all() built-in function in Python takes one iterable as an argument. It returns boolean True
if all values in the iterable are True
and False
if even one value iterable is boolean False
. You can think of an iterable as an object that contains other objects, which you can iterate through.
Here are a few examples that illustrate how the all() function is used:
>>> all([True, True])
True
>>> all([True, False])
False
>>> all([False, False])
False
>>> all([])
True
In the first example, we see that all values in the list are True, so the output is True. In the next two examples, the lists have at least one False value, so the all() function returns False. The last example is slightly confusing - it returns True with an empty list since there are no values in the list that are False.
What are True and False values in Python?
In the previous section, we only used the boolean values True
and False
. But the iterable can contain any data types, such as a string, integer, float, or even other data structures. So what classifies a data type as True or False?
Let's use Python's bool()
built-in function to determine which boolean value is given to certain common objects.
>>> bool(True)
True
>>> bool(False)
False
A string is boolean True if it contains any characters and False if it is an empty string:
>>> bool('')
False
>>> bool('abc')
True
A float or integer is boolean True if it has any non-zero positive or negative value, and False if it is 0:
>>> bool(5)
True
>>> bool(-5.1)
True
>>> bool(0)
False
>>> bool(0.0)
False
Finding boolean values of lists and tuples is similar to that of strings. An empty list or tuple is boolean False, while a list or tuple with at least one value is boolean True.
>>> bool([])
False
>>> bool(())
False
>>> bool([1, 2, 'abc'])
True
Another value in Python that also evaluates to boolean False
, is the None
value:
>>> bool(None)
False
So, you can use the all()
function with values such as strings, integers, or data structures as shown below:
>>> all([1, 2, 'abc'])
True
>>> all([1, 2, 'abc', (), None])
False
>>> all([1, 2, 'abc', 0])
False
To learn more about the bool()
function check out the Python Documentation.
All() function with Tuple and Set
So, far we have only seen the all() function with a list supplied as an argument (aside from empty tuples). However, you can use any iterable such as a tuple, dictionary, or a set.
Using the all() function with a tuple or set behaves the same as a list. For example:
>>> all((1, 2, 'abc'))
True
>>> all((0, 1.1, '@'))
False
>>> all(())
True
>>> all(('1', '50%'))
True
Here, there are various data types inside tuples for the argument for the all() function. Sets also behave the same way. With the same values as the tuples in the previous example but within a set, we get the same outputs:
>>> all({1, 2, 'abc'})
True
>>> all({0, 1.1, '@'})
False
>>> all({})
True
>>> all({'1', '50%'})
True
All function with Dictionary
A dictionary is a data structure, also known as a mapping, that associates keys with values. So when using the all() function with a dictionary, the function uses only the keys to determine the output. For example:
>>> all({'a': '1', 'b': [2]})
True
>>> all({'a': '1', 0 : [2]})
False
>>> all({'a': '1', '' : [2]})
False
>>> all({'a': '1', 'b' : []})
True
First, we used an example where all keys and values in the dictionary are boolean True. Changing the key to 0 or an empty string, the function returns False. Changing a value to boolean False an empty list, we still get True as the output because all the keys are boolean True. This proves that the all() function only uses the keys.
If we want to use the values in a dictionary with the all() function, we have to use the dict.values()
built-in method. This method returns a list of all the values in a dictionary.
>>> test_dict = {'a': '1', 'b' : []}
>>> print(test_dict.values())
dict_values(['1', []])
>>> all(test_dict.values())
False
In the above example, test_dict
is the same dictionary used in the previous example. We see that using that dictionary with the dict.values()
built-in method causes the all() function to return False.
All function with individual Strings and Integers
Strings are iterables because they are a collection of individual characters. Passing a string to the all() function behaves the similarly to a list. For example:
>>> all('abc')
True
>>> all(' ')
True
>>> all('')
True
>>> all('this that')
True
Note that passing a string to the all() function always returns True. This is because there's no way for there to be a character in the string that is empty, and an empty string behaves the same as an empty list, which we know returns True.
However, integers and floats are not iterables and will output a TypeError
:
>>> all(1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
>>> all(0)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
>>> all(1.1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'float' object is not iterable
all() vs any()
If the all() function uses the boolean and
operator across all the items in an iterable, then Python's built-in function any()
uses the boolean or
operator. The any() function returns True if any value in the iterable is boolean True, and returns False only if all the values in the iterable are boolean False. For example:
>>> any([1, 2, 'a'])
True
>>> all([1, 2, 'a'])
True
>>> any([5, '', 0])
True
>>> all([5, '', 0])
False
>>> any([])
False
>>> all([])
True
>>> any([(), '', 0])
False
>>> all([(), '', 0])
False
Here, we see various lists applied to both the any() and all() functions. With the first list, both functions return True because all values are boolean True. But in the next list, we have two values that are boolean False. The any() still returns True because we have at least one boolean True value: 5. But, since not all the values are boolean True the all() function returns False.
Next, we see that unlike the all() function an empty iterable returns False for the any() function because we need at least one value in the iterable with boolean True.
Finally, we see that both all() and any() return False when there are no values in the iterable that are boolean True.
Check out Python's Official Documentation to learn more about the any() function.
Understanding All function with for loop
To better understand the all() function let's try to make our own versions of the all() and any() functions:
>>> def my_all(vals):
... output = True
... for i in vals:
... if not i:
... output = False
... break
... return output
...
>>> def my_any(vals):
... output = False
... for i in vals:
... if i:
... output = True
... break
... return output
...
Above, both functions my_all()
and my_any()
are very similar. The key difference is that for one the variable output
is initially set to True, and for the other, it's set to False. The my_all() function loops through the iterable, until it finds a value that is not True and sets output
to False. The my_any() on the other hand breaks out of the for loop when the value is True and sets the output
to True. And of course, they both return the same values as their respective original functions:
>>> print(my_all([]), all([]))
True True
>>> print(my_any([]), any([]))
False False
>>> print(my_all([123, 'abc']), all([123, 'abc']))
True True
>>> print(my_any([123, 'abc']), any([123, 'abc']))
True True
>>> print(my_all([123, 'abc', 0]), all([123, 'abc', 0]))
False False
>>> print(my_any([123, 'abc', 0]), any([123, 'abc', 0]))
True True
Summary
In this article, we first looked at the basic usage of the all()
function in Python. Then, we looked at the boolean representation of different data types and data structures. We then saw examples of using the all() function with various sequences as arguments. We saw how the all() function handles strings and integers. We compared the all() function to the any()
function. Finally, we used a for loop to create new functions that behave similarly to the all() and any() function to better understand how they work.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers