Python SyntaxError: can't assign to function call
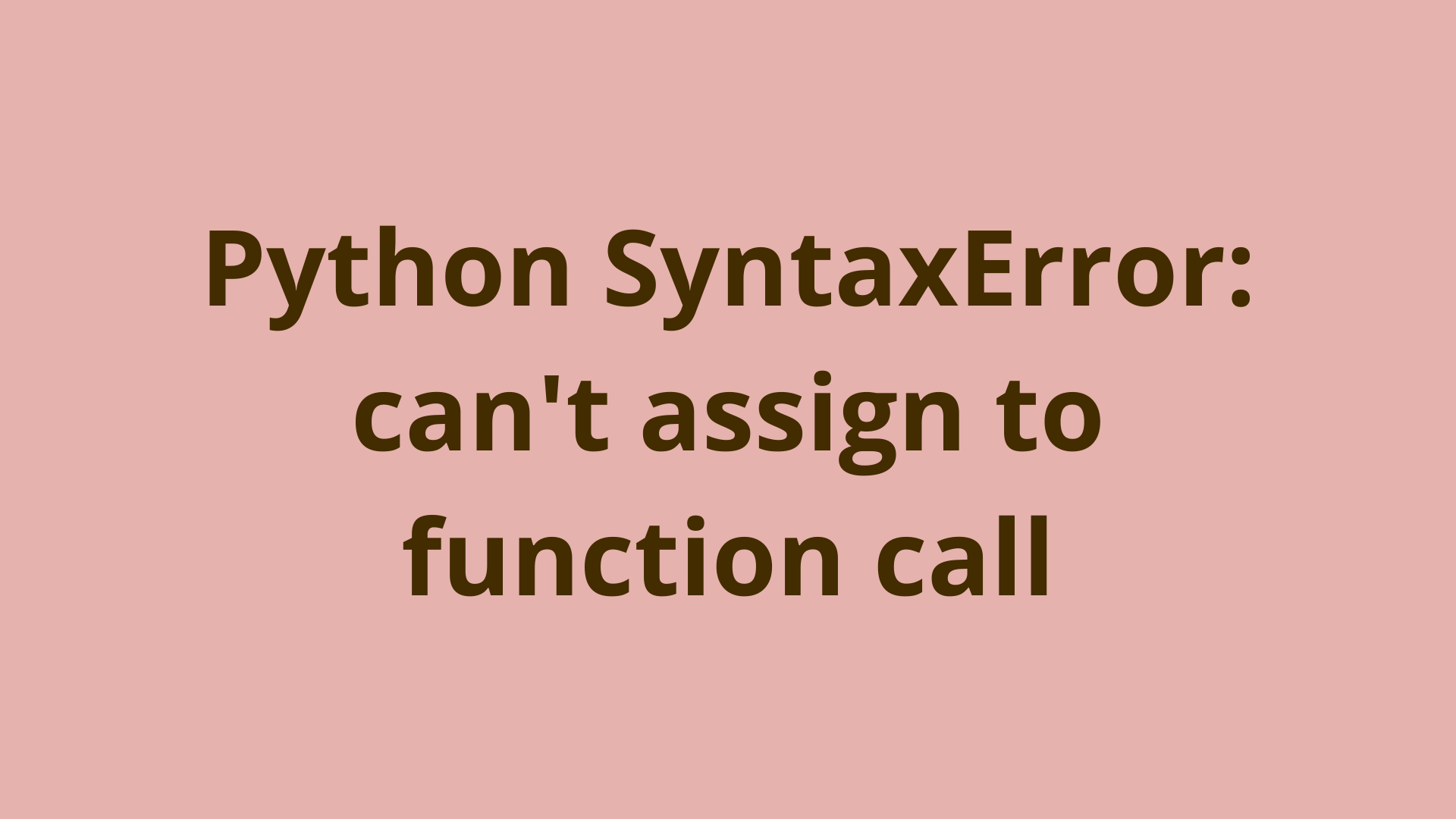
ADVERTISEMENT
Table of Contents
- Introduction
- When Will You Encounter this Error?
- What is a SyntaxError?
- Understanding Variable Assignment
- What is a Function Call?
- SyntaxError Resulting from Confusion Between Comparison Operator (==) and Assignment (=)
- Proper Way to Assign a Function to a Variable
- Summary
- Next Steps
Introduction
Function calls and variable assignments are powerful tools in Python. They allow us to make our code more efficient and organized. Function calls allow us to easily reuse blocks of code and variable assignments help us keep our code organized. However, if used incorrectly we can run into a SyntaxError
, which is a specific type of Python exception. Other types include AttributeError, and TypeError, but today we'll stick to this specific SyntaxError.
In this article, we will explore variable assignment, function calls, and syntax errors. We will then use our understanding of these concepts to fix or avoid the "SyntaxError: can't assign to function call" error in Python.
When Will You Encounter this Error?
This error occurs when putting a function call on the left-hand side of the equal sign in a variable assignment statement. An example of this error is shown below:
>>> def test_func(x):
... return x + 1
...
>>> test_func(1) = 'test_str'
File "<stdin>", line 1
SyntaxError: cannot assign to function call
This example uses a simple function named test_func
that takes an argument x
and returns x
plus 1 as its output. Then the function is called on the left-hand side of the equal sign with an attempt to assign the string 'test_str'
to it on the right-hand side of the equals sign.
Below is another example, where the built-in function list(...)
is called on the left-hand side of the equal sign and the integer 1 is assigned to it on the right-hand side of the equals sign. This code is in a module named assign_func_call.py
:
# assign_func_call.py
list() = 1
Then I run the script on the command line to produce the same error:
$ python3 assign_func_call.py
File "assign_func_call.py", line 3
list() = 1
^
SyntaxError: cannot assign to function call
Through these examples, we see that the specific function that is called on the left side of the equation doesn't matter - the error happens with both user-defined functions and built-in functions. Similarly, the specific value on the right side doesn't either, whether it is a string, integer, or anything else.
The error is caused by the incorrect arrangement of the function call and object (string, integer, list, etc) in the assignment statement, hence it being a SyntaxError
.
What is a SyntaxError?
A SyntaxError is a Python error that occurs due to writing code that doesn't obey the predefined rules of the language. It is a very common type of error due to human error occurring in the process of typing and using computers. The Python interpreter gives us helpful information when this type of error occurs, so fixing most syntax errors is pretty simple.
>>> def func():
... retun 1
File "<stdin>", line 2
retun 1
^
SyntaxError: invalid syntax
Let’s consider the SyntaxError
above, where the error occurs because the return
statement is clearly misspelled.
The first line tells us the line number where the error occurs, which is usually all you need to fix a SyntaxError
, since once you know what line it's on, it is sometimes pretty obvious what the problem is. If the error persists, the last line of the SyntaxError
gives more detailed information.
When looking for syntax errors, the Python interpreter scans the code from top to bottom. Furthermore, the Python interpreter specifically looks for syntax errors before any other type of error. For example, let's start by looking at this simple script that throws a different type of error:
# test_error.py
x = y
Now let's run the code on the command-line:
$ python3 test_error.py
Traceback (most recent call last):
File "test_error.py", line 3, in <module>
x = y
NameError: name 'y' is not defined
In this Python script, x
is assigned to a value not defined - y
, and running the script produces a NameError
(this is not a syntax error). Now let’s see another example:
# test_error.py
x = y
list() = 1
And run it:
$ python3 test_error.py
File "test_error.py", line 4
list() = 1
^
SyntaxError: cannot assign to function call
Here the list() = 1
line is after the x = y
line, yet we still get a SyntaxError
because Python checks for SyntaxError
before any other error.
The official python documentation gives more information on syntax errors Python Docs.
Understanding Variable Assignment
Variable assignment in Python is used temporarily give values to references that we can use in the code. We assign a variable to a value with the =
sign as follows:
>>> x = 1
>>> print(x)
1
In this example, we assign the integer value 1 to the variable x
and then print it to the console.
Python variable names are restricted to containing letters, digits, or underscores, and must only start with letters or underscores. The order of operations is important too. The variable must always go before the equal sign. The value being assigned must always go on the right side of the equals sign. A variable will always refer to the value it is assigned until you assign it a different value.
What is a Function Call?
Functions are an essential part of Python. They allow blocks of code to be reused multiple times. Functions can also make use of arguments to make them dynamic, so that we can call the same function but change specific values resulting in a different output or flow of instructions. For example:
>>> def func(x, y):
... return x + y
...
>>> func(1, 2)
3
>>> func(y=5, x=4)
9
>>> func(5, 5)
10
Here, the function func
is defined and returns the sum of the two arguments x
and y
. To call the function, we first write the function name followed by the arguments in parentheses. In this example, the function call prints the output only because the code is executing in interactive mode. The function call returns the value supplied in the return
statement, or null
if no return statement exists.
Check out Learn Functions in Python 3 to get a deeper understanding of functions.
SyntaxError Resulting from Confusion Between Comparison Operator (==) and Assignment (=)
One reason you could run into the SyntaxError: can't assign to function call
error is that you confused or mistyped the comparison operator ==
(double equals sign) instead of the =
(single equals sign) used for variable assignment.
The comparison operator ==
is used to check if two objects are equal, and will yield a boolean, either True or False. For example:
>>> list() == 1
False
>>> list() == []
True
The list()
function without arguments returns an empty list, so it's equal to the empty list but not equal to integer 1. However, if we were to use a single equals sign by mistake =
, Python thinks we are trying to assign the integer to the function call, and we get the SyntaxError
:
>>> list() = 1
File "<stdin>", line 1
SyntaxError: cannot assign to function call
Proper Way to Assign a Function to a Variable
There are two methods to assign a function to a variable. One method is to assign the result of a function call to a variable - like this:
>>> def func():
... return 1
...
>>>
>>> x = func()
>>> print(x)
1
Here, function func
returns 1. Then we assign the result of this function call to the variable x
, the function call being on the right side of the equal sign. Next, we print x
to show that it now holds the value returned by the function call. This method is used a lot when programming, to avoid having to call the same function multiple times, since its result is now stored in our variable.
The second method is assigning the function itself to a variable:
>>> def func():
... return 1
...
>>> print(func())
1
>>> x = func
>>> print(x())
1
>>> func = 2
>>> print(func)
2
In this example, calling function func
and printing the result displays 1. However, then we assign func
(without (), so it’s not a function call) to the variable x
. Next, we call x
to show that it returns 1 because it refers to the same function func
. Finally, we assign the 2 to func
(again no () so not a function call), and printing func
prints 2. From this example, we learn that function names are just like variable names, and we can refer to the same function with a different name. We can also reassign the function name to another object, and it will then refer to that object.
Summary
In this article, we discussed the Python issue SyntaxError: can't assign to function call
. We explained why the error occurs and how to fix it.
We looked into what SyntaxError
is and how to use the information provided by the Python interpreter to debug such an error. We also explored how variable assignment works and the proper syntax to use for an assignment statement. We then looked at the basic usage of a function call. Finally, we looked at proper ways to assign a function and its result to a variable.
Next Steps
Understanding function calls and variable assignments will not only allow you to avoid this type of syntax error, but will also allow you to write more organized and efficient code.
However, this is only one of such errors you will encounter when programming in Python. Our post Return Outside Function Error in Python explores another such Python error.
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers