IOError in Python | How to Solve with Examples
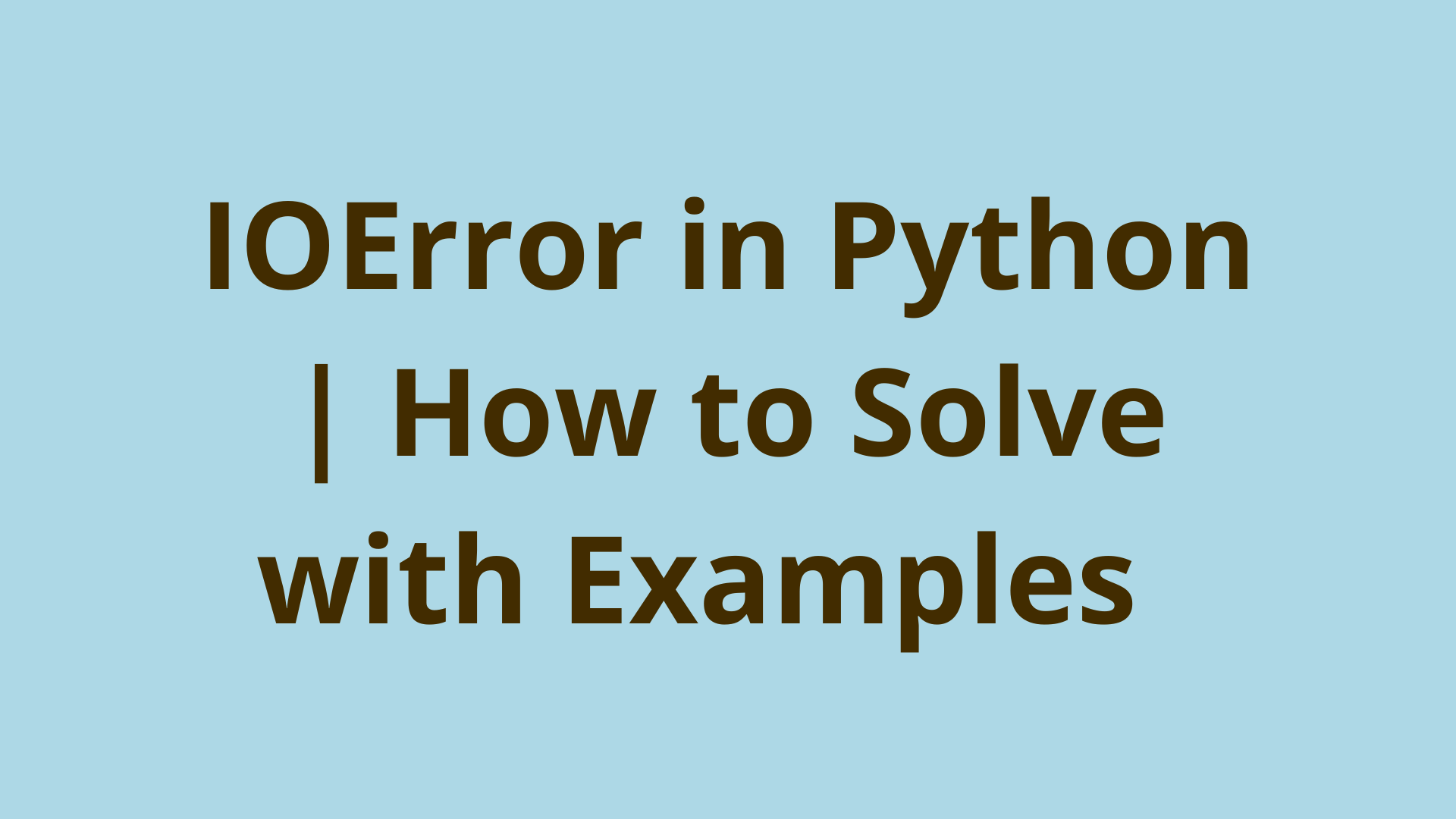
ADVERTISEMENT
Table of Contents
- Introduction
- What is IOError in Python?
- How do you make an IOError in Python?
- How does Python handle IO built-in exceptions?
- How do I fix Python error No such file or directory?
- Resolving Python IOErrors
- Avoiding Python IOError Exceptions
- Summary
- Next Steps
- References
Introduction
The IOError is a built-in exception associated with the failure of an input or output task. The purpose of this article is to outline the functions of the io error as well as solutions to common problems. The io error has more to it than the exception it throws, and it's important to understand that going forward.
An io error is raised during the failure of an input/output system task.
In Python, errors don't appear randomly but rather show up in a specific context and situation. In order to make it easier to determine such contexts, Python exceptions are organized into classes and subclasses, and io error is no exception. From here, io error just gets more interesting.
What is IOError in Python?
The io error is an exception specific to input and/or output functions. In a system-related function such as open()
or read()
, any obstacles that prevent communication between your code and your computer system will be exhibited as a raised io error or similar exception. Similar exceptions might stem from io error itself, given that it has subclasses such as the FileNotFoundError
.
How do you make an IOError in Python?
The most common way to run across this error in Python is by using open()
and similar functions. These include the read()
, write()
, and close()
methods. These methods work in conjunction to help programmers manipulate both program files and files on the system. Therefore, any errors pertaining to file-system interaction are handled by IOError and its subclasses. Here are some common examples of IOErrors:
No such file or directory
>>>new_file = open('InnitialCommit.txt', 'r')
Traceback (most recent call last):
File "main.py", line 1, in <module>
new_file = open('InnitialCommit.txt', 'r')
FileNotFoundError: [Errno 2] No such file or directory: 'InnitialCommit.txt'
In the code above, a file is opened in read mode, thus the use of 'r'
in the open()
function's parameters. Since the string value file being opened has not been defined or written to, an error is raised for attempting to read its nonexistent contents. This is an error that's easily made when working with file systems in Python; however, there are ways to avoid it that are covered further on.
I/O operation on closed file
>>>some_file = open('somefile.txt', 'w')
>>>some_file.close()
>>>some_file.read()
Traceback (most recent call last):
File "main.py", line 3, in <module>
some_file.read()
ValueError: I/O operation on closed file.
We can see from above, that the error raised is a ValueError
exception. It states that an I/O operation was attempted on a closed file. While an IOError was the expected result, a `ValueError was given instead. That was because all I/O operations had already stopped trying to read the file. Thus, the IOError is not thrown since no I/O commands were carried out in the first place.
How does Python handle IO built-in exceptions?
The IOError is part of a larger group of built-in exceptions. This group of built-in exceptions makes up the OSError exception class and includes exceptions relating to socket errors and other I/O issues.
The OSError class is part of Python's OS Module and is also a built-in exception. As previously mentioned, a programmer can manipulate computer functions with code; so the OSError class serves to handle any errors given by the system, AKA the "OS". That's not all to OSError though, IOError is one of the subclasses of OSError, alongside socket.error
, EnvironmentError
, and other subclasses. Within these subclass exceptions. It is the job of IOError to pay specific attention to the opening, closing, and alteration of files.
How do I fix Python error No such file or directory?
Example 1: In this example, the file 'ini.txt' does not exist in the system.
>>>file = open('ini.txt', 'r')
Traceback (most recent call last):
File "main.py", line 1, in <module>
file = open('ini.txt', 'r')
FileNotFoundError: [Errno 2] No such file or directory: 'ini.txt'
Though the error raised is a FileNotFoundError, which is a subclass of the familiar IOError, it still serves to show that any failed alteration during an input or output function will result in an error.
Example 2: This example creates a file, writes to it, closes it, deletes it, then attempts to read the deleted file.
>>>import os ## Using the OS module to delete files directly from the IDE
>>>
>>>new_file = open('InnitialCommit.txt', 'w')
<div id='waldo-tag-14053'></div>
## Creating new file and setting it to write "w" privileges.
>>>new_file.write("Hello World!") ## Write content to file
>>>new_file.close()
>>>
>>>os.remove("InnitialCommit.txt") ## Deleting file
>>>
>>>print(open('InnitialCommit.txt', 'r')) # Attempting to print contents of InnitialCommit.txt
Traceback (most recent call last):
File "main.py", line 9, in <module>
print(open('InnitialCommit.txt', 'r'))
FileNotFoundError: [Errno 2] No such file or directory: 'InnitialCommit.txt'
This error is yet another FileNotFoundError, but we know from the information above that this error is simply a subclass of IOError. Since the file was created but deleted, any system calls referencing it would raise some sort of io error.
Resolving Python IOErrors
OS errors, and by extension, IOErrors can be avoided by the use of a try/except block. This piece of coding knowledge is useful for raised exception handling in general but the focus, for now, is on IOErrors.
The try/catch is composed of two functions, each contained within its own try
block and catch
block. The try block will attempt to run the code within its scope; if successful, the code continues executing while skipping over the catch block. If the code in the try block fails, then the catch block intercepts any errors thrown. The catch block will then execute any alternative programming contained within itself, thus allowing the program to continue without stopping for an error.
Example:
>>>try:
... temp_file = open('nonexistant.txt', 'w') ## Attempt to open file
... except:
... print("An error occured while opening the file.")
An error occurred while opening the file.
The try/except block above takes in the given command and intercepts any errors thrown if there are any. It then presents a custom message or performs a separate function, depending on what the programmer needs it to do.
If you want to do even more with handling exceptions, there is also the finally keyword which can be appended onto try/except blocks for further actions.
Avoiding Python IOError Exceptions
When working with files in Python, it's generally a good idea to always work with try/catch blocks in order to avoid headaches. Also, keeping track of which files you alter and when will help to avoid altering a file at an inopportune time.
Summary
This article went over what the io error is and how it handles input and output. It is a subclass under OSError class and works alongside several other subclasses to cover a variety of OS-related errors. The io error in particular watches for the alteration of files and thusly has several of its own subclass errors to throw for specificity's sake. Errors like FileNotFoundException
are one such subclass of io error.
In order to avoid IOErrors and other exceptions, it's useful to have a try/catch block. The try/catch can not only catch the raised exception being thrown but allow the code to continue undisturbed.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- OSError - https://docs.python.org/3/library/exceptions.html#inheriting-from-built-in-exceptions).
- Finally with try/catch - https://www.w3schools.com/python/ref_keyword_finally.asp.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers