Python ValueError Exception – How to Identify & Handle
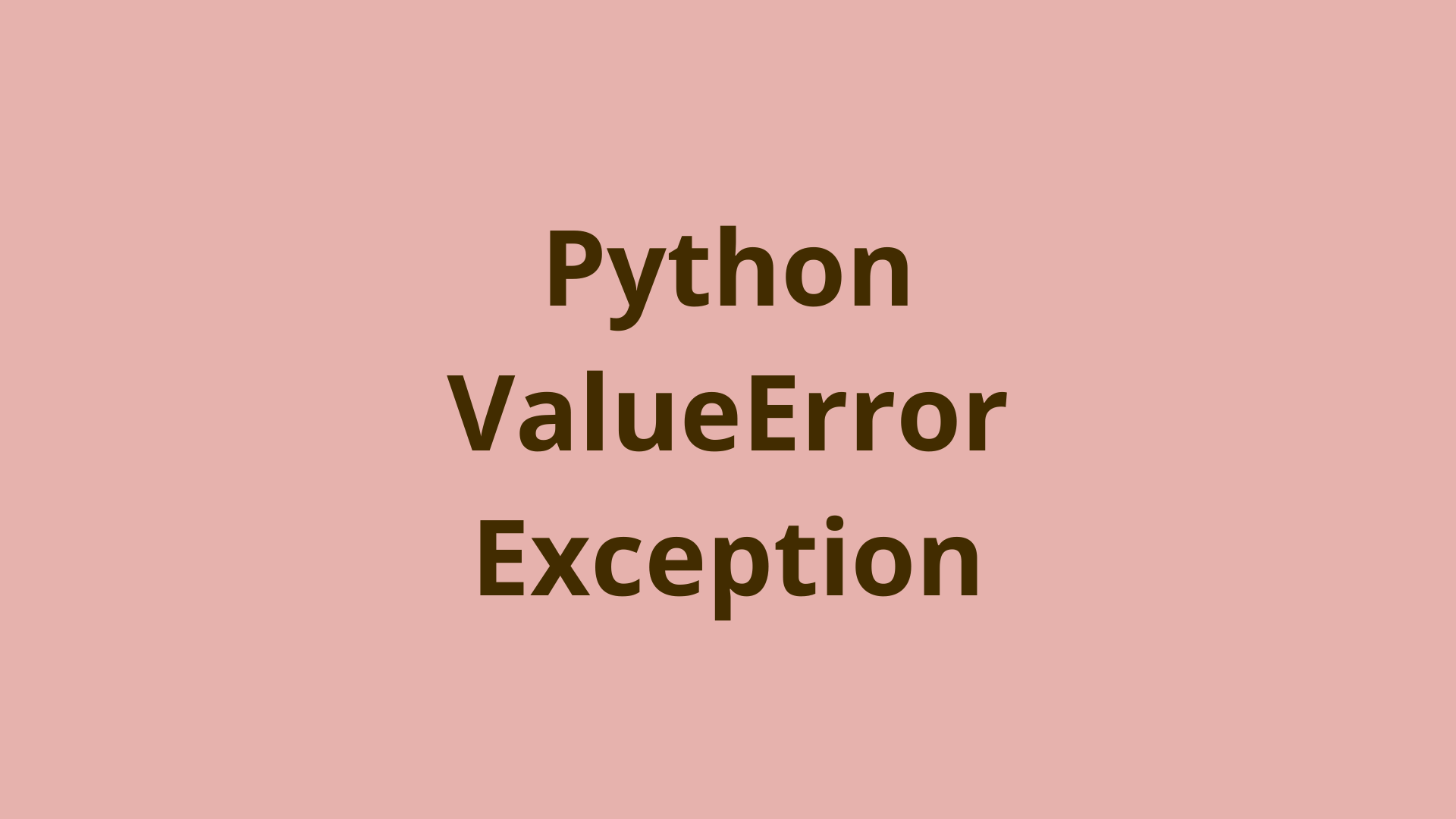
ADVERTISEMENT
Table of Contents
- Introduction
- What are Python built-in exceptions?
- What are the 3 types of errors in Python?
- What is python valueerror?
- How do you fix a value error in Python?
- math.sqrt() with a Negative Number
- Removing Value from a List or Set
- How do you raise a value error exception in Python?
- Handling ValueError using try and except clause
- Summary
- Next Steps
- References
Introduction
While programming you are bound to run into errors or exceptions when writing Python scripts. The name of each exception can tell you what type of error you've encountered, and the message provides more detail, this is what makes debugging so much easier in Python. However, the ValueError
exception is a little bit different. Although it refers to a specific type of error, it can be caused by a variety of reasons. This can make it difficult to identify its cause.
In this article, you will learn about the various ways ValueError occurs, and how you can resolve and avoid them in the future.
What are Python built-in exceptions?
Exceptions in Python occur during the execution of the program when the program encounters an error. Exceptions can be built-in/default and can even be raised in code using the raise
keyword. There are many different built-in exceptions in Python, and they give you useful error messages when raised. You can use these error messages to fix the error accordingly.
What are the 3 types of errors in Python?
Some of the common exceptions include:
SyntaxError
- occurs when there is incorrect syntax in the code.TypeError
- occurs when an operation is performed on the incorrect type of object.ImportError
- occurs when a module imported does not exist, or is not found.
What is python valueerror?
The official Python documentation states:
exception ValueError: Raised when an operation or function receives an argument that has the right type but an inappropriate value, and the situation is not described by a more precise exception such as IndexError.
The ValueError occurs when you input an incorrect value into a function, but the type of the value is correct. For example, if a function needs an int value, but you input a string instead, this would cause the TypeError, not ValueError. However, if you input a negative int value into a function that takes a positive int value only, this will raise the ValueError. Of course, the prime example of this is when you try to square root a negative number:
>>> import math
>>> math.sqrt('abc')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: must be real number, not str
>>> math.sqrt(-1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: math domain error
>>>
Here, TypeError is raised when you input a string for math.sqrt and a ValueError when you input a negative int.
How do you fix a value error in Python?
There are many different causes for the ValueError, so in this section, we will discuss the common causes, and how you can fix them. Note that fundamentally this exception occurs (as the name implies) when the argument's type in a function call is correct, but specifically the value is incorrect.
math.sqrt() with a Negative Number
You can fix the ValueError encountered with math.sqrt in the previous section, by simply making sure the input is positive.
>>> math.sqrt(100)
10.0
You could also use try/except to catch this raised exception and handle the exception with your own code.
Using int function with an Invalid Data Value
As you might already know, the built-in int()
function takes a string, float, or any representation of a number, and returns an integer. However, if you input a string with non-numeric characters, or an unconventional representation of a float, the int() function can return a ValueError. Here are some examples:
Non-numeric Character
>>> int('a')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: 'a'
Here we are trying to input a non-numeric character, which results in a value error. You can fix it by making sure to input a string representation of an integer (a string containing only digits):
>>> int('1')
1
Float
>>> int('0.1')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '.1'
Here, int cannot convert a string representing a float and returns the ValueError. To convert the string representing a float, you first use the float() function to convert the string into a float, and then convert the output into an int, since the int() function does accept a float:
>>> int(float('0.1'))
0
Binary String
>>> int(b'102', 2)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 2: b'102'
>>>
Here we input an invalid binary string b'102'. Note that the second argument in int() is the base of the number, which is by default 10. We can fix this issue by making sure to specify the base as 2 and making sure the binary string only contains 1s and 0s:
>>> int(b'101', 2)
5
Unpacking Values from a List/Tuple
Another common reason for the occurrence of the ValueError is when unpacking items in a tuple or list into variables. In Python, you can store each item in a list or tuple into specific variables with syntax like this:
>>> a, b, c = [1, 2, 3]
>>> print(a, b, c)
This can be very useful when you want to assign multiple variables in the same line, improving readability. Note that the number of variables must be exactly equal to the number of items in the list/tuple. If it's not, you will encounter the ValueError. For example:
>>> a, b = [1, 2, 3]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: too many values to unpack (expected 2)
>>> a, b, c = [1, 2]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: not enough values to unpack (expected 3, got 2)
The only way to fix this error is to make sure both the number of variables and items are the same.
Removing Value from a List or Set
You can also encounter the ValueError when using the built-in method remove() on a list. This method is used to remove an item of a specific value from a list or set. However, you will encounter the ValueError when you try to remove an item from the list which does not exist. Note, that this error only occurs with lists, not sets, which would cause the KeyError
.
>>> l = [4, 1, 2, 5, 3]
>>> l.remove(100)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: list.remove(x): x not in list
>>>
How do you raise a value error exception in Python?
Like any other exception, you can raise the ValueError in Python using a custom message. For example:
>>> raise ValueError("There's an Error with the value!")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: There's an Error with the value!
This could be useful by itself when you want to raise the ValueError for a situation where there could be an error because of the value of the input. But, this is especially useful with the try and except syntax.
Handling ValueError using try and except clause
The try and except syntax is used to catch exceptions, and handle them appropriately.
As discussed previously, you can handle the math.sqrt ValueError with negative numbers using try and except in conjunction with raise. Let's see how you can do this using an example:
>>> num = -1
>>> try:
... sqrtNum = math.sqrt(num)
... except ValueError:
... raise ValueError("Number is negative. num must be 0 or positive")
...
Traceback (most recent call last):
File "<stdin>", line 2, in <module>
ValueError: math domain error
During handling of the above exception, another exception was raised:
Traceback (most recent call last):
File "<stdin>", line 4, in <module>
ValueError: Number is negative. num must be 0 or positive
>>>
With try/except you can catch the ValueError, and then raise the ValueError again with a more meaningful message.
Summary
In this article, you learned about ValueErrors, how they are different from other built-in exceptions, and various ways to handle them.
You started by briefly looking at what Exceptions are in Python and then specifically the ValueError, and why it's different than other Exceptions. Then, you explored various ways the ValueError could occur and how to fix them. Next, you learned what the raise keyword is in Python and how you can use it. Finally, you looked at how you can use the raise with try/except to handle ValueErrors.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Exceptions - Official Python documentation - https://docs.python.org/3/library/exceptions.html#ValueError
- Exceptions in Python - https://www.edureka.co/blog/exceptions-in-python/
- Python ValueError - https://www.educba.com/python-valueerror/
Final Notes
Recommended product: Coding Essentials Guidebook for Developers