Python zfill Method
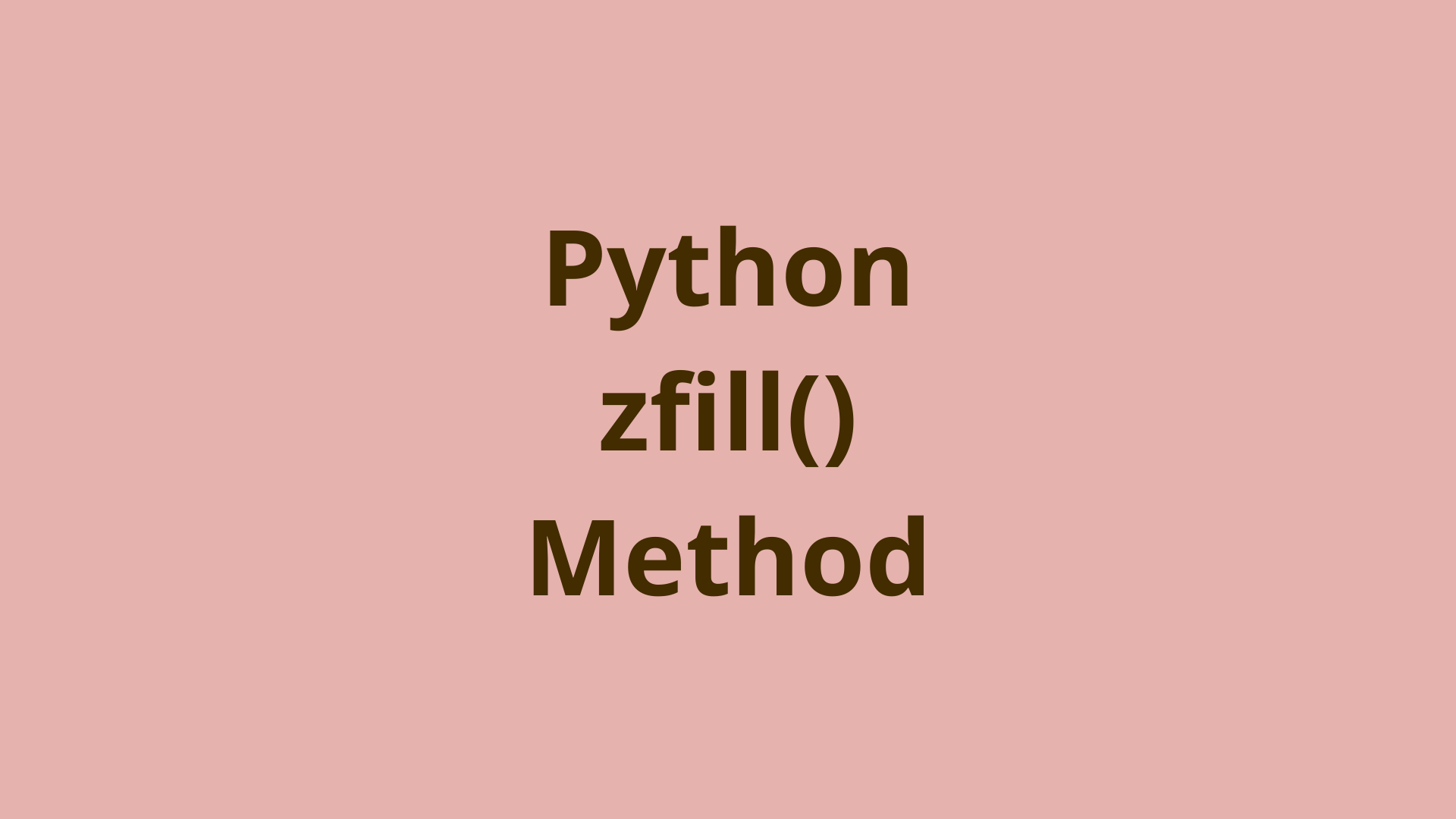
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of the zfill method
- Positive and Negative Sign with zfill
- Replicating zfill with string format method
- Replicating zfill with rjust method
- Summary
- Next Steps
Introduction
Python has a vast array of built-in methods that help us quickly implement an algorithm, that would otherwise take much more time to create ourselves. The accessibility of these methods is part of what makes Python such a powerful programming language.
In this article, we explore Python's built-in method zfill()
. We discuss how it interacts with different inputs and also suggest some alternatives depending on the situation.
Overview of the zfill method
zfill()
is a built-in string method in Python that is used for padding zeros to the left side of the string. The syntax for the zfill() method is:
some_string.zfill(integer_value)
We apply the zfill() method to a string value while passing a single argument, which must be an integer. It returns the zero-padded version of the original string. The argument it takes is the length of the string that should be returned after padding is performed on it. For example:
>>> orig = "hello"
>>> len(orig)
5
>>> padded = orig.zfill(10)
>>> padded
'00000hello'
>>> len(padded)
10
Here, we have the string orig
with the length of 5. When we use the zfill() method on string orig
with argument 10, the resulting value (stored in the variable padded
) has zeros before it. The length of padded is 10, which is the argument we gave the zfill() method. From this example, we can understand that zfill makes the string have a length we want it to have. To obtain this new length it adds the required number of zeros at the beginning of the string.
But then what will happen if the argument given is an integer less than the original string's length? In this case, zfill() will return the original string because the main purpose of zfill() is to fill in the number of slots in a string to make it the desired length.
>>> orig = "hello"
>>> orig.zfill(3)
'hello'
>>>
It doesn't matter how much smaller the argument value is compared to the original string length. It could even be a negative integer, and the zfill() method would still return the original string.
>>> orig.zfill(-3)
'hello'
>>> orig.zfill(-10)
'hello'
>>> orig.zfill(-100)
'hello'
We can also use a string that contains numbers with the zfill() method:
>>> "512".zfill(5)
'00512'
>>> "64".zfill(5)
'00064'
This can be useful when formatting binary numbers as text since they are often represented in 32-bit or 64-bit formats. Therefore they often have a lot of leading zeros. So, for example, you could use the zfill() method to add the leading zeros required for a 32-bit representation of the binary number 6:
'110'.zfill(32)
'00000000000000000000000000000110'
Positive and Negative Sign with zfill
The zfill() method has an interesting behavior regarding leading negative and positive signs at the beginning of the string. When there is a positive or negative sign as the first character in the string and the zfill() method is used on that string, the zeros used for padding are added after that sign. For example:
>>> orig = "-hello"
>>> orig.zfill(8)
'-00hello'
>>> orig = "+hello"
>>> orig.zfill(8)
'+00hello'
>>>
Here, we see the string orig with a positive and negative as its first character, and the zeros are padded after the signs with the zfill() method. Of course, this feature is advantageous when dealing with numbers:
>>> num = "-10"
>>> num.zfill(5)
'-0010'
>>> num = "+10"
>>> num.zfill(5)
'+0010'
In this scenario, there is one notable point that is easy to miss. Even though the zeros are padded after the sign, the sign still counts as a character. We can see in the previous example that the integer 5 was used for the argument, and the length of the resulting string including the positive or negative sign, matches that.
Replicating zfill with string format method
The zfill() method does have features that make it useful in certain situations. However, you can use other methods to replicate this functionality. One such way is by using the string format()
method.
This method is a powerful tool for formatting strings and integers. Of course it can also pad zeros to a string like so:
>>> formatted = '{:>010d}'.format(50)
>>> formatted
'0000000050'
>>> len(formatted)
10
Here we used the format() method with the integer 50, and it returns a padded string. The syntax inside the curly braces might seem confusing at first, but it's quite simple.
The syntax for padding goes after the semicolon :
. Before the semicolon goes the index specifying the argument to use, since format() can have multiple strings or integers as arguments and format them into one string.
After the semicolon is the greater than symbol >
which signifies that the padded zeros need to go before the specified index. If we use the less-than symbol (<), the zeros will go after the index:
>>> '{:<010d}'.format(50)
'5000000000'
After the greater than symbol is the number 0 that represents that we are using the character 0 for padding. We can also do padding with the space character by simply removing the zero.
>>> '{:>010d}'.format(50)
'0000000050'
>>> '{:>10d}'.format(50)
' 50'
>>>
Next, we have the number 10 which is like the argument for zfill() specifying the length of the resulting string, and we can change it to any integer:
>>> '{:>05d}'.format(50)
'00050'
>>> '{:>015d}'.format(50)
'000000000000050'
Finally, have the character 'd' that signifies that we are using an integer for padding. But if we use the character 's' instead, strings can be used as well:
>>> '{:>010s}'.format('hello')
'00000hello'
We can see that the format() method is a much more powerful tool than zfill() and has more functionality. However, the Pythonic way of coding is simplicity. So oftentimes it's better to use a simpler method that does the specific task than overcomplicate things with unnecessary functionality. This will make the code easier to read and debug later on.
There is one thing that the zfill() method does that format() method doesn't. The format() method doesn't pad zeros after the positive or negative sign:
>>> '{:>010d}'.format(-50)
'0000000-50'
>>> '{:>010s}'.format('-50')
'0000000-50'
So in certain situations, the zfill() method will be the best or only way to perform a task.
Replicating zfill with rjust method
Another method that can replicate the function of the zfill() method is the rjust()
string method. For example:
'00000hello'
>>> new_str = 'hello'.rjust(10, '0')
>>> new_str
'00000hello'
>>> len(new_str)
10
The rjust() method takes two arguments. The first argument is the same as the argument for the zfill() method, and the second argument allows us to choose what character to use for padding. Unlike the format() and zfill() methods, you can add padding with any character. If you don't specify the second argument rjust() just does padding with the space character. For example:
>>> 'hello'.rjust(10)
' hello'
>>> 'hello'.rjust(10, 'a')
'aaaaahello'
There is another string method ljust()
that has all the same functions as the rjust() method but does padding after the string. The r in rjust() represents that the string moves to the right, and the l in ljust represents that it moves to the left.
Here are some examples of the ljust() method:
>>> 'hello'.ljust(10, '0')
'hello00000'
>>> 'hello'.ljust(10, 'a')
'helloaaaaa'
>>> 'hello'.ljust(10)
'hello '
Similar to the format() method, the rjust() method doesn't work the same way as the zfill() method with negative and positive signs:
>>> '-50'.rjust(10, '0')
'0000000-50'
Summary
In this article, we took a look at the zfill() built-in method in Python. We examined how to use the zfill() method in various ways. We saw how the zfill() method interacts with positive and negative sign characters. Finally, we illustrated some alternative methods to do what the zfill() method does and noted when each might be the best option.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers