Using raw_input() in Python 3
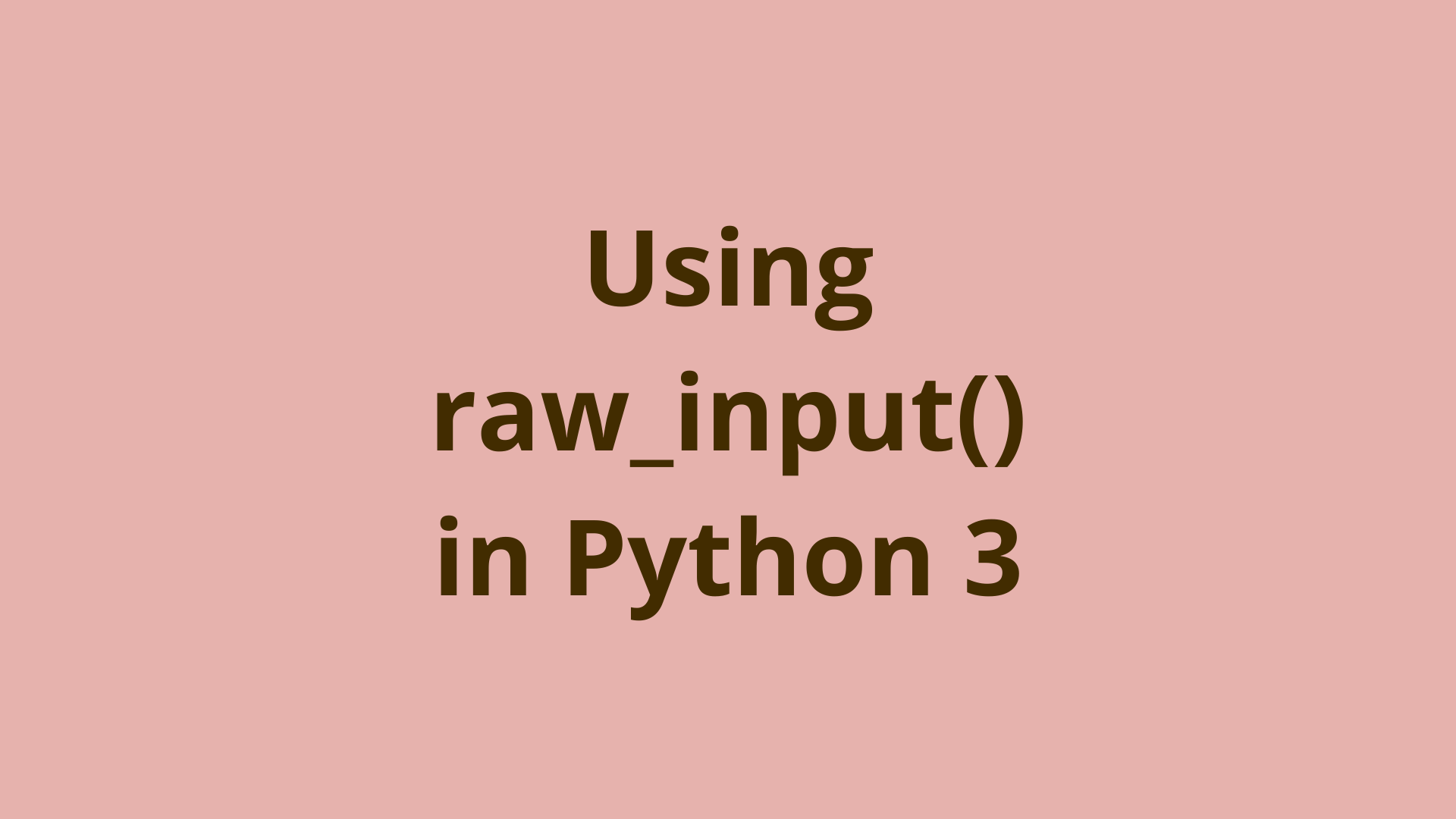
ADVERTISEMENT
Table of Contents
- Introduction
- raw_input() function in Python 2
- input() function in Python 2
- Why was the original input() removed from Python?
- input() function in Python 3
- Multi-Line user input
- Summary
- Next Steps
Introduction
User interaction is an essential part of many programs. The websites and apps that we use every day offer many ways to interact with them, often allowing users to supply their own input in various ways.
For programs that run on the command-line, programming languages typically offer a way to prompt the user to supply some typed input. This user-supplied input can then be stored in a variable in the code and used in the program's flow. Python has included this functionality for many years.
However, when Python 2 was superseded by Python 3, major changes were made. These included changes to user input functions. This can make it confusing for developers to track down the correct method to use for user input in Python command-line programs.
In this article, we will review how to accept user input in Python 2 and Python 3. We will look at what changed in Python 3 and discuss why these changes were made.
raw_input() function in Python 2
Python 2 included the built-in function raw_input()
, to get to prompt command-line users for a user input string. This function prompts the user to type in some text at the command line and returns that text as a string.
The most common way to use this function is to assign its result to a variable, and then perform some actions with that data:
>>> # Python 2
>>> fruit = raw_input()
apples
>>> print(fruit)
apples
>>>
In the third line of the code above, the raw_input() function prompts the user to type a value and stores that value as a string in the variable fruit
. The key thing to remember is that raw_input() always returns a string, even if the user types in other types of values, such as a number:
>>> # Python 2
>>> num = raw_input()
12
>>> num
'12'
>>> type(num)
<type 'str'>
If the user is not instructed that the program is waiting for them to type in an input, they may think the program is hanging or that it crashed.
The print
statement can be used to inform the user that they have to input some value:
>>> # Python 2
>>> from __future__ import print_function # Access Python 3 print function in Python 2
>>>
>>> def getInput():
... print("Enter name of a fruit: ", end="")
... fruit = raw_input()
... return fruit
...
>>> fruit = getInput()
Enter name of a fruit: apple
>>> fruit
'apple'
In the above example, we did this in Python 2 without adding an '\n' (end line character) in the printed message, by using the print
function from Python 3. This was done by accessing future enhancements via the __future__
library. This writes your message to the user in the print function before the raw_input() function, as shown above.
However, this is actually unnecessary because you can perform this task much more easily with an argument in the raw_input() function in Python 2. This argument is a string that is displayed to the user to prompt them for user input, so they are aware they need to input some data. For example:
>>> # Python 2
>>> fruit = raw_input("Enter name of a fruit: ")
Enter name of a fruit: apples
>>> print(fruit)
apples
>>>
However, if you have strict formatting requirements for multiple lines of prompting, it can be helpful to use both print statements and the raw_input() argument.
input() function in Python 2
Python 2 has another built-in function to get user input, the input()
function. The main difference between raw_input() and input() is datatype of the functions' return value. The raw_input() function specifically returns the user's input as a string, while the input() function can accept Python literals and return a variety of Python datatypes. It can even return the result of a Python code expression (which is one of the reasons it was removed from Python 3 for security reasons).
This might seem confusing at first. But, it pretty much that the input() function can receive different data types, such as integers, lists, dictionaries, etc depending on the user's input. Let's look at an example to better understand it:
>>> # Python 2
>>> a = input("> ")
> 12
>>> type(a)
<type 'int'>
>>> b = input("> ")
> [1, 2, "abc", "xyz"]
>>> b
[1, 2, 'abc', 'xyz']
>>> type(b)
<type 'list'>
When the input() function prompts for an input, if the user types the integer 12, the value 12 is stored as an integer in variable a
. This would have been converted to a string when using the raw_input() function, as we saw previously. Similarly, a list is stored in variable b
if the user inputs it in its literal Pythonic form, including the square brackets. This list can be used like a normal Python list.
>>> # Python 2
>>> b
[1, 2, 'abc', 'xyz']
>>> b[2]
'abc'
>>>
Allowing users to input arbitrary data structures may seem useful at first glance, but it has major issues and has been removed in Python 3. Let's see why...
Why was the original input() removed from Python?
First, we should clarify that the input() function in Python 2 is completely different from the input() function in Python 3. As we mentioned before, Python 2 included both raw_input() (which always converted the user's input to string datatype) and input() which accepted the datatype provided by the user.
Python 3, input()
is the only option that exists, but somewhat confusingly, it behaves like the raw_input() from Python 2 - that is - it converts all user input to the string datatype.
The main reason why the original functionality of the input() function was removed in Python 3 is for security. The input() function from Python 2 essentially enabled the user to execute raw code, giving the user the power to manipulate code and the inner workings of a program.
For example, in Python 2, a user could execute a function from the input() prompt:
>>> # Python 2
>>> def func(x):
... return x + 1
...
>>> a = input("> ")
> func(1)
>>> print(a)
2
>>>
Giving the user so much power can be dangerous, and it could be used with malicious intent, and has therefore been removed in Python 3.
input() function in Python 3
The input()
function in Python 3 is exactly the same as raw_input() in Python 2, just with a different name. You can use the input() function in Python 3 in the same way as raw_input() from Python 2:
>>> # Python 3
>>> a = input("Enter a fruit: ")
Enter a fruit: orange
>>> a
'orange'
>>> num = input("Enter a number: ")
Enter a number: 15
>>> num
'15'
>>>
Here you can see that input() always returns a string in Python 3 and accepts a string (prompt) argument in the same way as raw_input().
Multi-Line user input
Python 3's input() function (and Python 2's raw_input()) can store only one line of user input at a time. Using the \n
(endline) character in the input prompt does not work either:
>>> # Python 3
>>> twoLines = input("> ")
> line one\n line two
>>> print(twoLines)
line one\n line two
>>> twoLines
'line one\\n line two'
>>>
And it is also wrong to assume that the user knows what the \n
character is. But with a simple while loop, we can create an intuitive multi-line user prompt:
>>> # Python 3
>>> allLines = []
>>> print("Type some multi-line input (type END when done):\n")
>>> while True:
... line = input("> ")
... if line == "END":
... break
... else:
... allLines.append(line)
...
Type some multi-line input (type END when done):
> This is my first line
> This is my second line
> This is my third line
> This is my last line
> END
>>>
Here, you first create a list allLines
to store all the user lines. Then, you have to setup an infinite while loop, which prompts the user for input again and again and stores the input in the allLines
list. The loop will end when the user inputs the text "END". It's also a good idea to inform the user how to end the user prompt with a print message.
Next, you need to format the user input correctly:
>>> # Python 3
>>> allLines
['This is my first line', 'This is my second line', 'This is my third line', 'This is my last line']
>>> userInput = "\n".join(allLines)
>>> print(userInput)
This is my first line
This is my second line
This is my third line
This is my last line
>>>
You can then join the lines in the allLines
list with the .join
string method as shown above. This will allow you to store the multi-line user input in one variable.
Summary
In this article, we discussed the various functions you can use in Python to get user input from the command-line.
First, you saw how to use the user input functions in Python 2: raw_input() and input(). Then you saw why the functionality for the input() function in Python 2 was removed in Python 3. Next, you saw how to use the input() function in Python 3, which is the same as raw_input() from Python 2. Finally, you saw how you can accept and format multi-line user input with a while loop.
Next Steps
If you're interested in learning more about the basics of coding, programming, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers