Python Ternary Operator
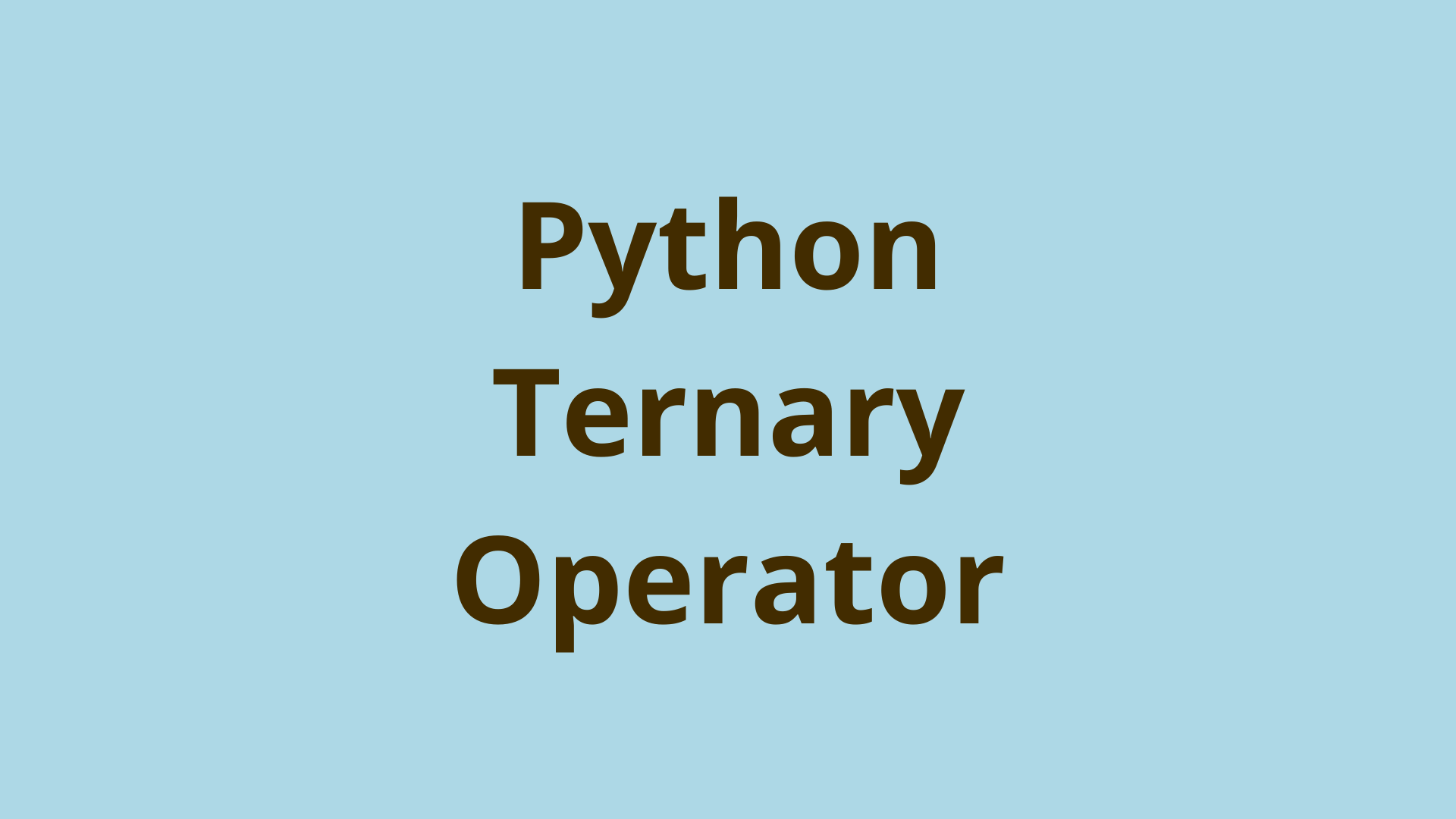
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of Common Ternary Operator
- Ternary in Python
- Ternary Operator in Tuple Format
- Shorthand form of Ternary Operator
- Ternary Operator inside other statements
- Summary
- Next Steps
Introduction
Computer programming has many tools that allow programmers to implement various forms of logic. The ternary operator is one such tool and it is used to implement basic conditional expressions. It is part of many programming languages, including Python.
In this article, we will look at how we can use ternary operators in various ways with Python.
Overview of Common Ternary Operator
Ternary in the English language means: to be in three parts. This definition reflects how ternary operators are implemented in programming.
Ternary operators are used to make if
statements more concise by writing them in one line, which is why they are often referred to as the "conditional operator", "inline if" (iif), or "ternary if".
Ternary operations generally involve three parts:
- A condition that evaluates to either a boolean True or False value
- The value to return when the condition is true
- The value to return when the condition is false
In many programming languages, the ternary operator is implemented as a question mark and colon syntax such as condition ? value_if_true : value_if_false
.
As you can see, this expression is made up of three parts, which is why it's called the ternary operator. However, as we will see, Python does things a bit differently.
Ternary in Python
In Python, there is no question mark and colon syntax for ternary operation. So in that sense you can say there is not one single "ternary operator". However, the functionality can be achieved in a few ways.
These ternary operations were not implemented in Python when it was created. They were added later on in Python version 2.4. There are several ways to implement this behavior, so lets start with the most basic one.
First, let us look at a simple if
statement:
>>> likes_apples = True
>>>
>>> if likes_apples:
... apples = "likes apples"
... else:
... apples = "doesn't like apples"
...
>>>
>>> print(apples)
likes apples
Here, if the likes_apples
variable is boolean True we assign "likes apples" to the apples
variable, otherwise we assign "doesn't like apples". This takes a total of 4 lines and we have two lines dedicated to assignments to the apples
variable. The ternary syntax simplifies this operation. With Python's ternary operator conditional expressions, we can do the same operation like this:
>>> likes_apples = True
>>> apples = "likes apples" if likes_apples else "doesn't like apples"
>>> print(apples)
likes apples
Now we get the same results, but with only one line and the code reads like normal English language and we only assign apples
once. This simplicity and readability are what make ternary operators nice in Python.
Note that the order of the 3 elements differs from the standard question mark and colon format we mentioned above.
Ternary Operator in Tuple Format
Another format you can write a ternary operator in is the Tuple format. For example:
>>> likes_apples = True
>>> apples = ("doesn't like apples", "likes apples")[likes_apples]
>>> print(apples)
likes apples
To understand this syntax, we have to know that this format simply uses a tuple and indexing to get the value. In Python, boolean False and True can evaluate to the integers 0 and 1, respectively. So, when using True for the index is the same as using the integer 1:
>>> ("first", "second")[True]
'second'
>>> ("first", "second")[False]
'first'
>>>
So in the first example, in the tuple, the first value (index 0) needs to be the value you want when likes_apples is False and the second value (index 1) needs to be the value you want when likes_apple is True. You can also do this format with lists since they are so similar to tuples:
>>> likes_apples = False
>>> apples = ["doesn't like apples", "likes apples"][likes_apples]
>>> print(apples)
doesn't like apples
Shorthand form of Ternary Operator
There is also a shorthand form of the ternary operator, which is essentially a boolean OR operator. For example:
>>> likes_apples = "likes apples"
>>> apples = likes_apples or "doesn't like apples"
>>> print(apples)
likes apples
>>>
>>> likes_apples = False
>>> apples = likes_apples or "doesn't like apples"
>>> print(apples)
doesn't like apples
Here, we see that to make this example work with the shorthand form of the ternary operator, when likes_apples
is True, the value on the right side of the or
operator needs to be the string that you want to assign. To understand why we can just use a string in-place of boolean True, you can check out the section "What are True and False values in Python?" in our post Python All() Function.
Next, it is very important that the variable likes_apples
is before the or
. In Python, the or
operator checks values from left to right. So, starting from the left if any value is boolean True, that value is returned. We can see this in the second example, where we assign likes_apples
to False, and consequently the next value evaluated by or
is the string "doesn't like apples" which is boolean True and therefore it is returned.
Ternary Operator inside other statements
In all the previous examples we assigned the output of the ternary operators to a variable. However, we can place the ternary operator anywhere a regular expression can be used in code. This includes inside statements, function arguments, return values, etc. For example:
>>> likes_apples = True
>>>
>>> print("likes apples" if likes_apples else "doesn't like apples")
likes apples
>>>
>>> print(("doesn't like apples", "likes apples")[likes_apples])
likes apples
>>> print(["doesn't like apples", "likes apples"][likes_apples])
likes apples
>>>
>>> likes_apples = "likes apples"
>>> print(likes_apples or "doesn't like apples")
likes apples
>>>
Here, we see placing the same ternary operators we assigned to a variable with the basic format, tuple format and the shorthand form in the previous sections inside a print statement works perfectly and prints the output.
Summary
In this article, we took a look at ternary operators in Python. These provide an easy and concise way to implement if
statements in one line. We described the basic syntax for a ternary operator equivalents in Python.
We also looked at various other ways to implement the functionality of a ternary operator, including tuple format and shorthand form. Finally, we looked at how the ternary operator can be used inside other statements for even simpler code.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers