Serve Static Resources with Spring Boot
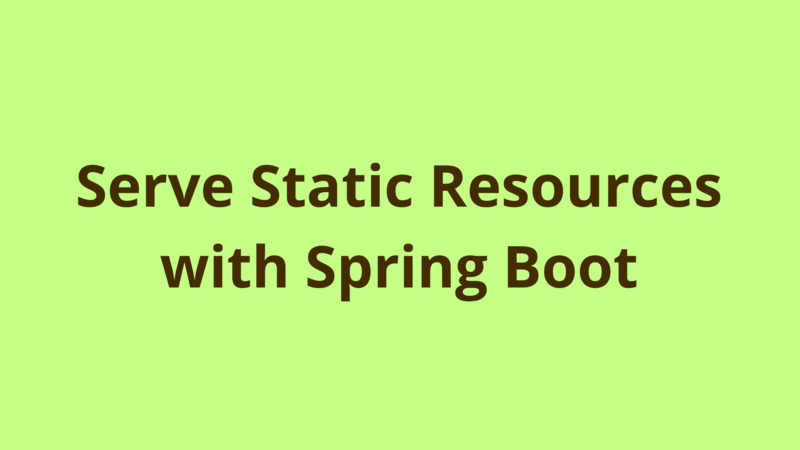
ADVERTISEMENT
Table of Contents
- Introduction
- 1- Default path for static resources
- 2- Welcome page
- 3- Serve HTML files from Controller
- Summary
- Next Steps
Introduction
In this tutorial we show how Spring Boot serves static resources like (html, js, css) in a web application.
1- Default path for static resources
By default Spring Boot serves static resources defined under the following paths:
- /META-INF/resources/
- /resources/
- /static/
- /public/
Except for index.html which is served as the root resource when accessing the root URL of a Spring Boot application, this page need not exist under the above paths.
In order to change the default paths of static resources, you can define “spring.resources.static-locations” attribute under application.properties as the following:
spring.resources.static-locations=/html/,/js/,/css/
2- Welcome page
By default, Spring boot serves index.html as the root resource when accessing the root URL of a web application.
In order to define your own root resource, you can map the root path ‘/’ inside your controller and return your own html as the following:
@Controller
public class HomeController {
@RequestMapping("/")
public String welcome(Map<String, Object> model) {
return "home.html";
}
}
home.html should exist under any of these paths:
- src/main/resources/META-INF/resources/home.html
- src/main/resources/resources/home.html
- src/main/resources/static/home.html
- src/main/resources/public/home.html
3- Serve HTML files from Controller
In order to return an HTML view from a controller, you need to return its full name plus the extension knowing that it’s located under any of the paths defined in step-1.
Here below we map /home URL to load home.html view:
@RequestMapping("/home")
public String home(Map<String, Object> model) {
return "home.html";
}
Summary
In this tutorial we show how Spring Boot serves static resources like (html, js, css) in a web application.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers