Servlet 2.5 – Hello World Tutorial
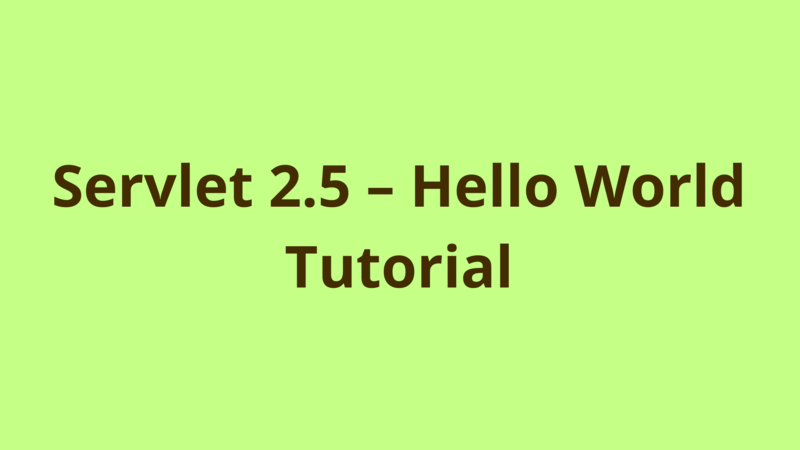
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Create dynamic web project
- 2. Create Hello World servlet
- 3. Deploy the applicaton
- 4. Access the application
- Summary
- Next Steps
Introduction
In this tutorial, we provide a step-by-step guide for creating a hello world web application using Servlet 2.x technology.
Prerequisites:
- Eclipse IDE (Mars release)
- Java 1.7
- Apache tomcat 7
1. Create dynamic web project
Open eclipse, then select File -> New -> Dynamic Web Project.
In the next screen, fill the mandatory fields as above, in case you didn’t previously link apache tomcat to eclipse, then just click on “New Runtime” button and link your tomcat, in this tutorial i use Apache tomcat 7.
Click “Next”.
Click “Next”.
in the final screen, make sure to check ‘Generate web.xml deployment descriptor’ checkbox, so that eclipse generates automatically the web.xml file under WEB-INF.
Here we go, the structure of the generated project looks like the following:
2. Create Hello World servlet
Right click on the src folder, then select New -> Servlet
In the next screen, select the name and the package of the servlet then click “Next”.
In this screen, you can define the url mapping of the new servlet, by default the mapping is set to the servlet name. Just keep it as is, you can always change it later on inside web.xml.
Here you define the inherited methods to be generated inside the servlet, by default each servlet should implement doGet and doPost methods, so just keep it as is and click “Finish”.
After clicking finish, eclipse automatically creates a servlet class named HelloWorld.java under com.programmer.gate package as the following:
/**
* Servlet implementation class HelloWorld
*/
public class HelloWorld extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public HelloWorld() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
response.getWriter().append("Served at: ").append(request.getContextPath());
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
it also writes the definition of the servlet under web.xml as the following:
<servlet>
<description></description>
<display-name>HelloWorld</display-name>
<servlet-name>HelloWorld</servlet-name>
<servlet-class>com.programmer.gate.HelloWorld</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloWorld</servlet-name>
<url-pattern>/HelloWorld</url-pattern>
</servlet-mapping>
Our new servlet can be accessed by:
In order to return a hello world html message from our servlet we modify the doGet() method to be as the following:
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
response.getWriter().println("<h1>Hello World!</h1>");
}
3. Deploy the applicaton
Follow this guide in order to setup Tomcat 7 in eclipse.
Then deploy your application to Tomcat 7, just drag and drop the application to the tomcat instance under servers view.
Now that the application is deployed successfully under Tomcat 7, in order to start tomcat, right click on tomcat instance -> Start.
4. Access the application
After starting up tomcat, you can access the “Hello World” servlet using the following url:
http://localhost:8085/Servlet-2-Hello-World/HelloWorld
In order to find the port number of the application, double click on the tomcat instance in servers view:
These port attributes are editable and you can change them anytime.
/Servlet-2-Hello-World: denotes the deployed application name.
/HelloWorld: denotes the name of the requested servlet, it is the value of
Finally, the output of the url would look like:
Summary
In this tutorial, we provide a step-by-step guide for creating a hello world web application using Servlet 2.x technology.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers