Spring Boot – How to solve OAuth2 ERR_TOO_MANY_REDIRECTS
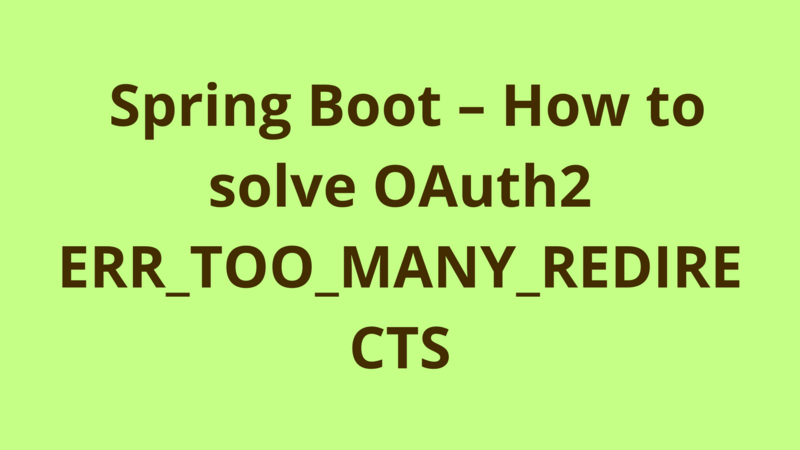
ADVERTISEMENT
Table of Contents
Introduction
When redirecting back to your application after a successful OAuth2 authentication, the following error occurs:
Solution
This error occurs when the redirect URL set under the authorization service(Google, Facebook … etc) is not defined as a permitted URL inside your application.
The permitted URL is the one which can be accessed without authentication.
When the authorization service redirects to a non-permitted URL, the application will redirect back to the authorization service for further authentication and the process enters in a loop which doesn’t end causing ERR_TOO_MANY_REDIRECTS error to occur.
In order to permit the access to the callback URL with Spring Boot, you need to extend WebSecurityConfigurerAdapter and override the security configuration as the following:
@Configuration
@EnableOAuth2Sso
public class ApplicationSecurity extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.antMatcher("/**")
.authorizeRequests()
.antMatchers("/", "/login**","/callback/", "/webjars/**", "/error**")
.permitAll()
.anyRequest()
.authenticated();
}
}
In the above block, we consider /callback as our redirect URL, so we permit the access to it using permitAll() while we still secure the access for other URLs.
Summary
When the authorization service redirects to a non-permitted URL, the application will redirect back to the authorization service for further authentication and the process enters in a loop which doesn’t end causing ERR_TOO_MANY_REDIRECTS error to occur.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers