Spring – Read files from classpath
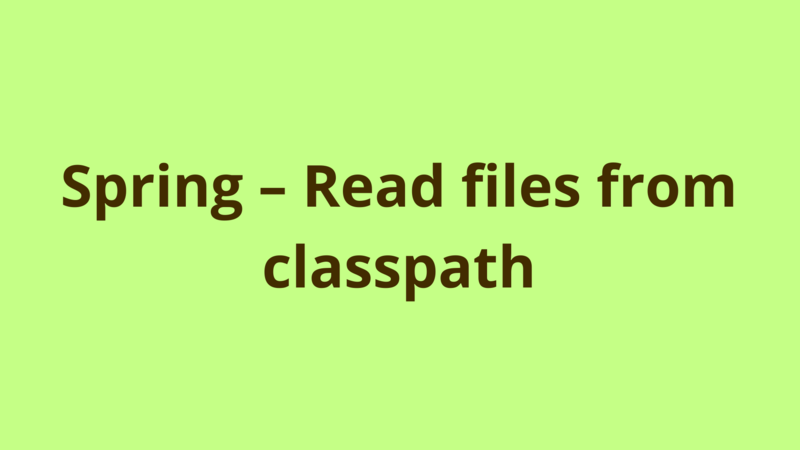
ADVERTISEMENT
Table of Contents
Introduction
In this tutorial, we discuss several ways of reading a resource file from classpath in a Spring MVC application.
1- ClassPathResource
Using ClassPathResource class provided by Spring Core, you can read a resource file using both absolute and relative paths.
To read a resource file through an absolute path, simply use:
Resource resource = new ClassPathResource("test.png");
To read it through a relative path, pass your current class as a second parameter:
Resource resource = new ClassPathResource("test.png", BaseController.class);
After that, you can use getFile() or getInputStream() to parse the content of the resource.
2- @Value
If your resource file is in a fixed location and you already know its path, you can define your resource as a class field and annotate it with @Value, so that it’s loaded at the startup of the application.
@Value("classpath:test.png")
Resource resourceFile;
After that, you’re free to use resourceFile field wherever you want inside your class.
3- ResourceLoader
Another way of reading a resource file in a Spring MVC application is through ResourceLoader.
You can simply define the ResourceLoader as a class field:
@Autowired
ResourceLoader resourceLoader;
And then use it in your method as the following:
Resource resource = resourceLoader.getResource("classpath:test.png");
After that, you can use getFile() or getInputStream() to parse the content of the resource.
4- ResourceUtils
Using ResourceUtils is not recommended by the Spring team as it’s only intended to be used internally in the Spring Core code.
However, it’s still an option and can do the job:
File file = ResourceUtils.getFile("classpath:test.png");
Summary
In this tutorial, we discuss several ways of reading a resource file from classpath in a Spring MVC application.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers