Static keyword in java
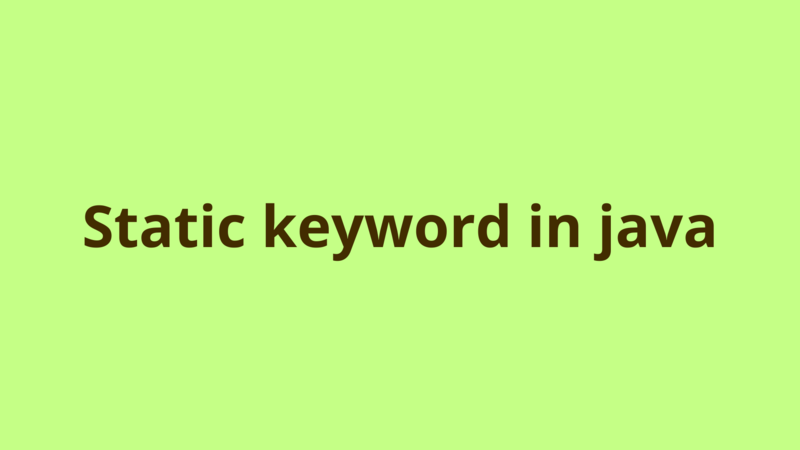
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Static variable
- 2. Static method
- 3. Static block
- 4. Some points to consider when using static keyword
- 5. Disadvantage of static keyword
- Summary
- Next Steps
Introduction
Every instance of a class has its own state and behavior, whenever the state of a particular instance is modified, other instances are not affected.
In java, a static keyword is normally applied to class members or methods in order to make them shared between all instances, the static keyword forces the variable or the method to only have one copy in the memory regardless how many instances of the class are created.
1. Static variable
A static variable is a variable which belongs to the class and has one value shared between all instances of the class. Any modification done on the variable is reflected on all the instances.
The typical usage of a static variable is when you define programatically an incremental ID of a class, in the following example, we define a Student class which holds id and name , the id is assigned programatically and is incremented upon each object creation.
public class Student {
private int id;
private String name;
private static int studentNumber = 1;
public Student(String name) {
this.name = name;
id = studentNumber++;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getStudentNumber() {
return studentNumber;
}
}
In the above class, we define ID as an instance variable since its value should be unique and different between all instances, at the same time we define a static variable called studentNumber which is incremented at each object creation. Each time a new instance of the class is created, the ID variable is assigned to the latest value of studentNumber.
In order to understand how static variables work, we create the following test method:
public static void main(String[] args) {
Student student1 = new Student("Alex");
Student student2 = new Student("Hussein");
System.out.println("Alex ID = " + student1.getId());
System.out.println("StudentNumber static variable = " + student1.getStudentNumber());
System.out.println("Hussein ID = " + student2.getId());
System.out.println("StudentNumber static variable = " + student2.getStudentNumber());
}
Output:
Alex ID = 1
StudentNumber static variable = 3
Hussein ID = 2
StudentNumber static variable = 3
we notice here that the value of studentNumber is shared between student1 and student2, while at the same time we are able to set a unique ID for each student.
2. Static method
A static method is a method which is shared between all instances of the class, developers normally use static keyword with utility methods which do some common functionality that is regularly used anywhere in the application, utility methods are invoked by the class name without the need to create an object before. e.g.
Some rules apply when defining a static method:
- Static methods can’t use instance variables, they usually take input from the parameters , perform some business then return some result.
- Static methods can’t invoke a non-static method.
- Static methods can’t be overridden.
- You can’t use this keyword inside a static method.
A typical example of a static method is an email validator , which takes as input an email then checks whether its valid or not.
public static boolean isValidEmailAddress(String email) {
boolean result = true;
try {
InternetAddress emailAddr = new InternetAddress(email);
emailAddr.validate();
} catch (AddressException ex) {
result = false;
}
return result;
}
3. Static block
A static block is a block of statements inside a java class which is executed when a class is first loaded into the JVM e.g. it is initialized the first time the class is referenced in the code.
Normally, static blocks are used for initializing static class variables, and they are different from constructors in that static blocks execute only once at the time of class loading and initialization by JVM while constructor is called every time a new instance of the class is created.
P.S: you can’t use instance variables or invoke instance methods inside the static block.
A typical example of using a static block is when you support retrieving an Enum instance by its value, to do that you define a hashmap as a static variable which maps each value to its corresponding Enum instance, the map is initialized and filled inside a static block before the Enum is ever used in the application.
public enum ErrorCodes {
BUSINESS_ERROR(100), SERVER_ERROR(500), NETWORK_ERROR(1000);
private int errorCode;
// This field maps each error code numeric value to a corresponding Enum instance.
private static Map<Integer, ErrorCodes> errorCodeByErrorNumber = new HashMap<Integer, ErrorCodes>();
static {
for (ErrorCodes errorCode : ErrorCodes.values()) {
errorCodeByErrorNumber.put(errorCode.getErrorCode(), errorCode);
}
}
private ErrorCodes(int errorCode) {
this.errorCode = errorCode;
}
public int getErrorCode() {
return errorCode;
}
public static ErrorCodes getErrorCodeByNumber(Integer dayNumber) {
return errorCodeByErrorNumber.get(dayNumber);
}
}
4. Some points to consider when using static keyword
- Static variables and methods are accessed through the class name:
. or . - Static keyword can be applied to variable, method, or inner class, it can’t be applied on top level class.
- Static fields are initialized at the time of class loading opposite to instance variables which are initialized at the instance creation.
- If a static block throws an exception, then you may get java.lang.NoClassDefFounfError when you try to access the class which failed to load.
5. Disadvantage of static keyword
Using the static keyword inside your application would lead to some serious problems:
Static variables are shared between all class instances, this causes major problems in multi-threaded environments where multiple threads can access and modify the variable at the same time. So developers should take attention when accessing the static variables inside the application, and they should surround each access with Synchronized block. Static methods can’t be mocked, mocking is a mechanism used in unit testing in order to fake the business of some methods, when a method is defined as static there is no way to mock its business.
Summary
Every instance of a class has its own state and behavior, whenever the state of a particular instance is modified, other instances are not affected.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers