How to use Enums in java
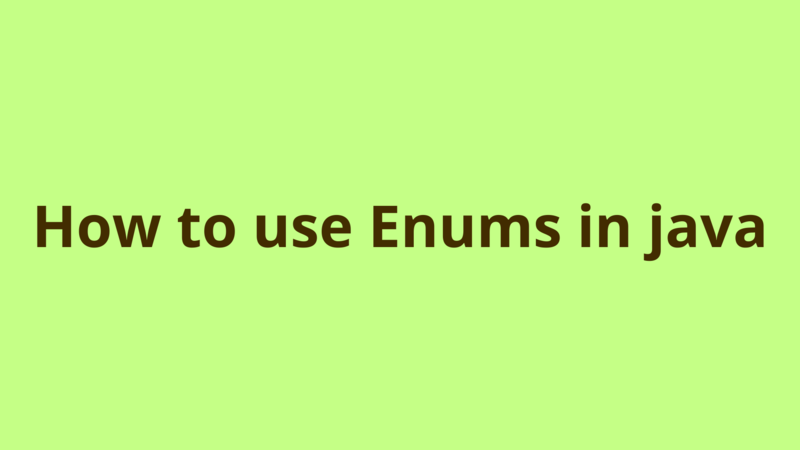
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Overview of Enum
- 2. A typical Enum
- 3. Common operations of Enum
- 3.2 Convert String to Enum
- 3.5 Retrieve Enum by value
- Summary
- Next Steps
Introduction
In this article, we provide several examples on the usage of Enums in java.
1. Overview of Enum
Enum is a java type/class which holds a fixed set of related constants, it is the replacement of the traditional definition of multiple static final variables in java. It is mostly recommended when you define a method which accepts a constant argument, this way you force the method callers to commit to the predefined constants and prevent them to pass random constant values.
2. A typical Enum
public enum ErrorCodes {
BUSINESS_ERROR(100), SERVER_ERROR(500), NETWORK_ERROR(1000);
private int errorCode;
private ErrorCodes(int errorCode) {
this.errorCode = errorCode;
}
public int getErrorCode() {
return errorCode;
}
}
The above enum can be represented traditionally as:
public static final int BUSINESS_ERROR = 100;
public static final int SERVER_ERROR = 500;
public static final int NETWORK_ERROR = 1000;
some points to consider when defining an enum:
- Enum implicitly extends java.lang.Enum, so it can’t extend other class.
- Enum constructors can never be invoked in the code, they are always called automatically when an enum is initialized.
- You can’t create instance of Enum using new operator, it should have a private constructor and is normally initialized as: ErrorCodes error = ErrorCodes.BUSSINESS_ERROR
- Each constant in the enum has only one reference, which is created when it is first called or referenced in the code.
3. Common operations of Enum
Following are common operations of Enum:
3.1 Instantiate Enum
private static void instantiateEnum() {
ErrorCodes businessError = ErrorCodes.BUSINESS_ERROR;
System.out.println(businessError);
}
Output:
BUSINESS_ERROR
3.2 Convert String to Enum
private static void convertStringToEnum() {
ErrorCodes businessError = ErrorCodes.valueOf("BUSINESS_ERROR");
System.out.println(businessError);
System.out.println(businessError.getErrorCode());
}
Output:
BUSINESS_ERROR
100
3.3 Compare enums
To compare enums, you can use: ==, equals() or switch() block.
private static void checkEnumForEquality() {
ErrorCodes businessError = ErrorCodes.BUSINESS_ERROR;
if(businessError == ErrorCodes.BUSINESS_ERROR)
{
System.out.println("You can check enum for equality using == operator.");
}
if(businessError.equals(ErrorCodes.BUSINESS_ERROR))
{
System.out.println("You can check enum for equality using equals() method.");
}
switch(businessError)
{
case BUSINESS_ERROR:
{
System.out.println("You can check enum for equality using switch block.");
break;
}
default: System.out.println("Non-business error.");
}
}
Output:
You can check enum for equality using == operator.
You can check enum for equality using equals() method.
You can check enum for equality using switch block.
3.4 Iterate over Enum values
private static void iterateEnumValues() {
System.out.println("Iterating over ErrorCodes enum");
for(ErrorCodes errorCode : ErrorCodes.values())
{
System.out.println("Enum key = " + errorCode);
System.out.println("Enum value = " + errorCode.getErrorCode());
}
}
Output:
Iterating over ErrorCodes enum
Enum key = BUSINESS_ERROR
Enum value = 100
Enum key = SERVER_ERROR
Enum value = 500
Enum key = NETWORK_ERROR
Enum value = 1000
3.5 Retrieve Enum by value
By default, java doesn’t provide any way to retrieve an Enum instance by its value. To do so, update ErrorCodes enum in order to expose a hashmap which maps each value to its corresponding Enum instance, the hashmap is filled and exposed as the following:
public enum ErrorCodes {
BUSINESS_ERROR(100), SERVER_ERROR(500), NETWORK_ERROR(1000);
private int errorCode;
private static Map<Integer, ErrorCodes> errorCodeByErrorNumber = new HashMap<Integer, ErrorCodes>();
static {
for (ErrorCodes errorCode : ErrorCodes.values()) {
errorCodeByErrorNumber.put(errorCode.getErrorCode(), errorCode);
}
}
private ErrorCodes(int errorCode) {
this.errorCode = errorCode;
}
public int getErrorCode() {
return errorCode;
}
public static ErrorCodes getErrorCodeByNumber(Integer errorNumber) {
return errorCodeByErrorNumber.get(errorNumber);
}
}
Now, you can retrieve an enum instance of a numeric value as the following:
private static void retrieveEnumByValue() {
ErrorCodes businessError = ErrorCodes.getErrorCodeByNumber(100);
System.out.println(businessError);
}
Output:
BUSINESS_ERROR
That’s it !!
Summary
In this article, we provide several examples on the usage of Enums in java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers