What is Spring Boot?
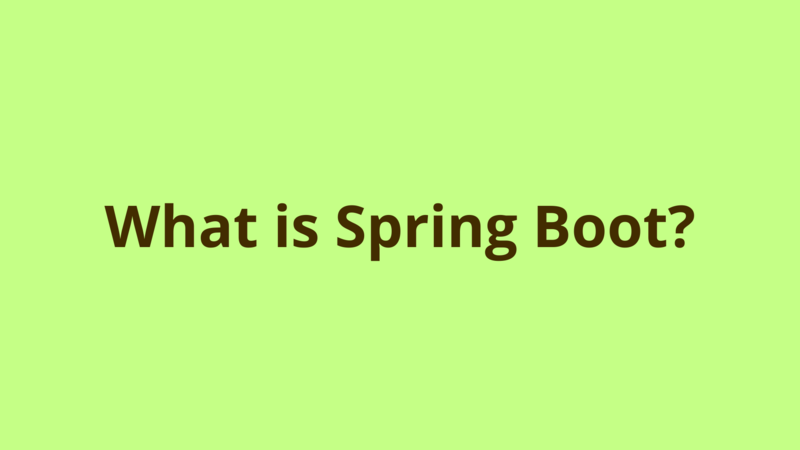
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Introducing Spring Boot
- 2. Spring Boot Features
- 2.3 Embedded Servlet Container Support
- 3. Spring Boot Requirements
- 4. Creating our first Spring Boot application
- 4.1 Create Maven web project
- 4.4 Create Application class
- 5. Deploy Spring Boot application
- Summary
- Next Steps
Introduction
Setting up an enterprise spring-based application has become a tedious and error-prone task due to the required bulky configuration along with the complicated dependency management especially if we’re talking about applications which make use of several third party libraries.
Each time you build an enterprise spring-based application, you have to repeat the same configuration steps:
- Import the required spring modules based on the type of application you’re creating i.e. Spring MVC, Spring JDBC, Spring ORM.
- Import the web container library (case of web applications).
- Import the required third-party libraries (i.e. hibernate, jackson), you have to hunt for the compatible versions with the specified Spring version.
- Configure DAO beans such as: data source, transaction management … etc.
- Configure web layer beans such as: resource manager, view resolver.
- Define a starter class which loads all the required configuration.
The above is a routine configuration procedure which should be done each time you create an enterprise java application based on Spring framework.
1. Introducing Spring Boot
Spring team decides to provide the developers with some utility which relatively automates the configuration procedure and speeds up the process of building and deploying Spring applications, so they invented Spring Boot.
Spring Boot is a utility project which aims to make it easy to build spring-based, production-ready applications and services with minimum fuss. It provides the shortest way to have a Spring web application up and running with smallest line of code/configuration out-of-the-box.
2. Spring Boot Features
There are a bunch of features specific to Spring Boot, but three of my favourite are dependency management, auto configuration and embedded servlet containers.
2.1 Easy dependency Management
In order to speed up the dependency management process, Spring Boot implicitly packages the required compatible third-party dependencies for each type of Spring application and exposes them to the developer using starters.
Starters are a set of convenient dependency descriptors that you can include in your application. You get a one-stop-shop for all the Spring and related technology that you need, without having to hunt through sample code and copy paste loads of dependency descriptors.
For example, if you want to get started using Spring and JPA for database access, just include the spring-boot-starter-data-jpa dependency in your project, and you are good to go. (no need to hunt for compatible database drivers and hibernate libraries).
Also if you want to create a spring web application, just add spring-boot-starter-web dependency, by default it pulls all the commonly used libraries while developing Spring MVC applications such as spring-webmvc, jackson-json, validation-api and tomcat.
In other words, Spring Boot gathers all the common dependencies and define them in one place and allow the developer to use them instead of reinventing the wheel each time he creates a new common application type.
Therefore, pom.xml becomes much smaller than the one used with traditional Spring applications.
2.2 Auto Configuration
The second awesome feature of Spring Boot is the auto-configuration.
After you select the appropriate starter, Spring Boot attempts to automatically configure your Spring application based on the jar dependencies that you have added.
For example, if you add spring-boot-starter-web, Spring Boot automatically configures the commonly registered beans like: DispatcherServlet, ResourceHandlers, MessageSource.
Also, if you’re using spring-boot-starter-jdbc, Spring boot automatically registers DataSource, EntityManagerFactory and TransactionManager beans and reads the connection details from application.properties file.
In case you don’t intend to use a database and you don’t provide any manual connection details, Spring Boot will auto-configure an in-memory database without any further configuration on your part whenever it finds H2 or HSQL library on the build path.
This is totally configurable and can be overridden anytime by custom configuration.
2.3 Embedded Servlet Container Support
Each Spring Boot web application includes an embedded web server by default, check this for the list of embedded servlet containers supported out of the box.
Developers don’t need to worry about setting up a servlet container and deploying the application on it. The application can be run by itself as a runnable jar file using its embedded server.
If you need to use a separate HTTP server, you just need to exclude the default dependencies, Spring Boot provides separate starters for HTTP servers to help make this process as easy as possible.
Creating standalone web applications with embedded servers is not only convenient for development, but also a legitimate solution for enterprise-level applications, this is increasingly useful in the microservices world. Being able to wrap an entire service (for example, user authentication) in a standalone and fully-deployable artifact that exposes an API makes distribution and deployment much quicker and easier to manage.
3. Spring Boot Requirements
Setting up and running Spring Boot applications require the following:
- Java 8+
- Spring Framework 5.0.1.RELEASE or above
- Servlet 3.0+ container (in case you don’t make use of the embedded servers).
4. Creating our first Spring Boot application
Now let’s get into practical part, we’re going to implement a very basic payment API similar to the one we used in the previous Spring tutorial.
We aim to highlight the facilities provided by Spring Boot which makes creating REST api way simpler than doing it using the traditional way.
4.1 Create Maven web project
Create a maven web project using this tutorial and name your project as SpringBootRestService.
Make sure to use Java 8+ since Spring Boot doesn’t work with earlier versions.
4.2 Configure pom.xml
The second step is to configure Spring Boot in pom.xml.
All Spring Boot applications extend from spring-boot-starter-parent, so before defining your dependencies, define the parent starter as the following:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.8.RELEASE</version>
</parent>
Now since we’re creating a REST api, we’re going to use spring-boot-starter-web as a dependency which would implicitly define all the required dependencies like: spring-core, spring-web, spring-webmvc, servlet api and jackson-databind library, so just add the following as a dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
The following jars are automatically imported to your project under Maven Dependencies:
The next step is to add the Spring Boot plugin as the following:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
Then define the Spring repositories:
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</pluginRepository>
</pluginRepositories>
The final step is to set the packaging property as jar, so that maven generates a runnable jar file on build.
<packaging>jar</packaging>
Here is the full working pom.xml :
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.programmer.gate</groupId>
<artifactId>SpringBootRestService</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.8.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</pluginRepository>
</pluginRepositories>
</project>
As you notice, using one dependency we’re able to create a fully functional web application.
4.3. Create REST resources
Now, we’re going to create our payment controller along with the request and response POJO classes, exactly as we did in the previous tutorial.
Following is the payment request class which should be submitted by clients on each payment request:
package com.programmer.gate;
public class PaymentRequest {
private int userId;
private String itemId;
private double discount;
public String getItemId() {
return itemId;
}
public void setItemId(String itemId) {
this.itemId = itemId;
}
public double getDiscount() {
return discount;
}
public void setDiscount(double discount) {
this.discount = discount;
}
public int getUserId() {
return userId;
}
public void setUserId(int userId) {
this.userId = userId;
}
}
And this is the base response returned back from our service:
package com.programmer.gate;
public class BaseResponse {
private String status;
private Integer code;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
}
And this is our controller:
package com.programmer.gate;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/payment")
public class PaymentController {
private final String sharedKey = "SHARED_KEY";
private static final String SUCCESS_STATUS = "success";
private static final String ERROR_STATUS = "error";
private static final int CODE_SUCCESS = 100;
private static final int AUTH_FAILURE = 102;
@RequestMapping(value = "/pay", method = RequestMethod.POST)
public BaseResponse pay(@RequestParam(value = "key") String key, @RequestBody PaymentRequest request) {
BaseResponse response = new BaseResponse();
if(sharedKey.equalsIgnoreCase(key))
{
int userId = request.getUserId();
String itemId = request.getItemId();
double discount = request.getDiscount();
// Process the request
// ....
// Return success response to the client.
response.setStatus(SUCCESS_STATUS);
response.setCode(CODE_SUCCESS);
}
else
{
response.setStatus(ERROR_STATUS);
response.setCode(AUTH_FAILURE);
}
return response;
}
}
4.4 Create Application class
This final step is to create the configuration and starter class, Spring Boot supports a new annotation @SpringBootApplication which is equivalent to using @Configuration, @EnableAutoConfiguration and @ComponentScan with their default attributes.
So you just have to create a class annotated with @SpringBootApplication and Spring Boot would enable the auto configuration and would scan for your resources in the current package:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
5. Deploy Spring Boot application
Now let’s make use of the third awesome feature of Spring Boot which is the embedded server, all we need to do is to just generate a runnable jar file using maven and run it as a normal standalone application.
- Right click pom.xml -> run-as -> Maven install
- Maven generates a runnable jar file called SpringBootRestService-1.0.jar inside target folder
- Open cmd, then run the jar using: java -jar SpringBootRestService-1.0.jar
Here we go, our REST api is up and ready to serve requests at port 8080 by default.
In this tutorial we introduced Spring Boot features and created a fully working example using the Spring Boot embedded server.
Hope you like it, for clarifications please leave your thoughts in the comments section below.
Summary
In this tutorial we introduced Spring Boot features and created a fully working example using the Spring Boot embedded server.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers