git clone | How to Clone a Repo in Git
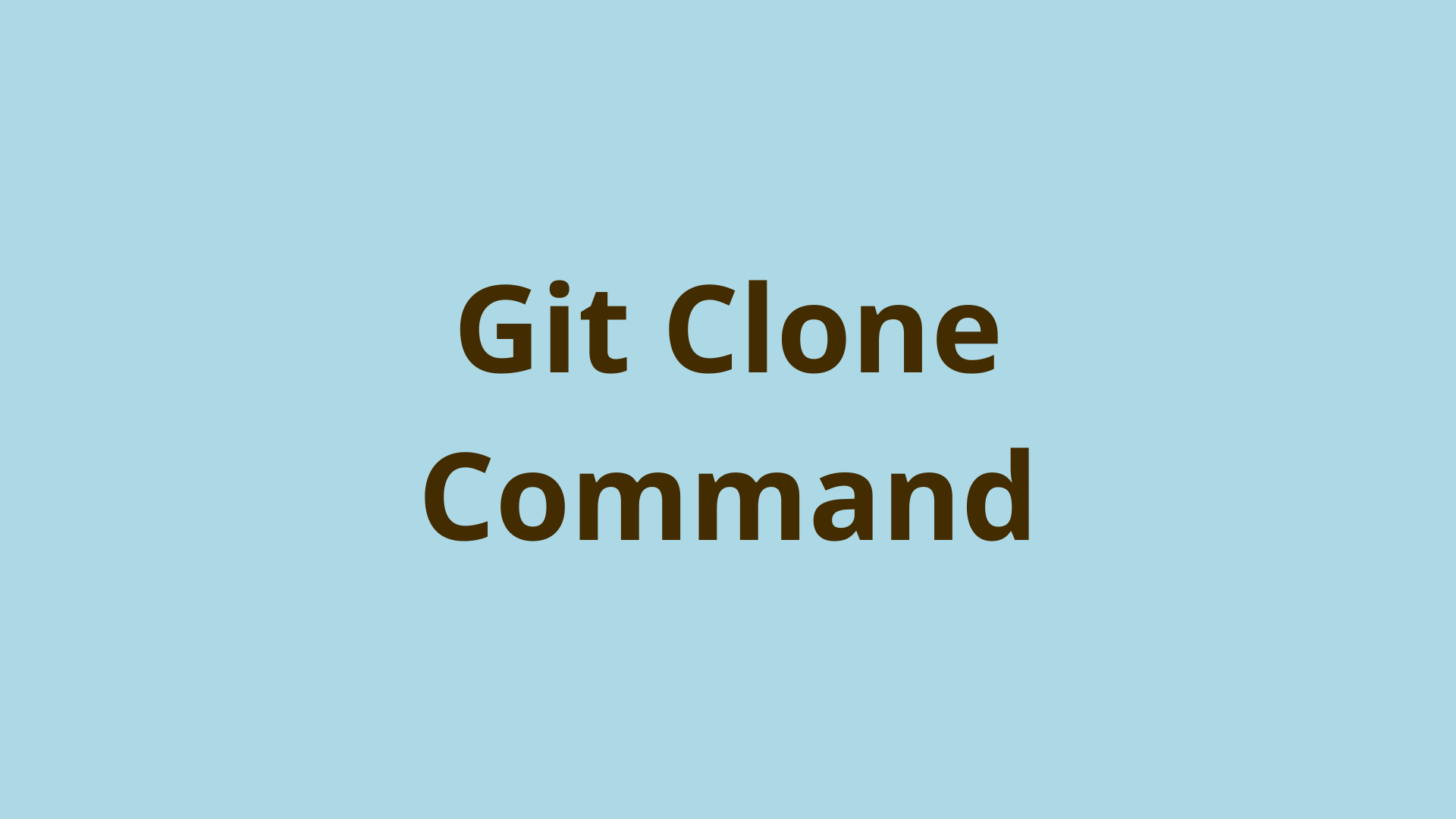
ADVERTISEMENT
Table of Contents
- Introduction
- Why Clone A Repository?
- How Do I Clone A Git Repository?
- Can I Clone A Local Repository?
- Does Git Clone Get All Branches?
- Adding Remotes to Your Git Clone
- Interacting With Remotes From Within Your Cloned Repository
- Git Clone SSH
- Git Clone HTTPS
- Git Clone Common Flags and Options
- Clone without tags
- Should I Clone Or Fork A Git Repository?
- Summary
- Next Steps
- References
Introduction
Git is a popular version control system (VCS) often used by programmers to collaborate on coding projects. Git is helpful in maintaining an accurate history of your project because it stores your projects and code in a repository or "repo" for short. Git repos track all changes and build up a revision history, making it easy to get a top-down view of all the changes made throughout the life of your project.
If you are starting from scratch, you will need to create a new Git repository. However, there will undoubtedly be times when you want to copy an existing repository. This is where Git clone comes in. Git clone is a helpful command for any developer to learn if you want to improve your Git workflow and become a more collaborative developer.
Continue reading to learn about the core features of git clone
and how it works, as well as some helpful commands and options.
Why Clone A Repository?
Using Git as your VCS has to start somewhere; in many cases, that starting point is an existing Git repository. When a developer wants to use Git to collaborate on coding projects, they make their own local copy of the Git repository using the git clone
command.
The main purpose of Git clone is to copy an existing repository to a new directory or location.
Once the repo has been cloned, the developer now has a working copy where they can work independently of the remote repository. The option exists to push your local changes into the remote repo, but you are free to add and commit changes locally without affecting the remote code base until the chosen time.
How Do I Clone A Git Repository?
Getting started with the basics of Git clone is very straightforward. A simple example of using git clone in the terminal looks like this:
$ git clone https://github.com/user/some-repo
$ cd some-repo
The first line clones the repo into a directory called some-repo, and the second line changes the directory into the newly cloned repo.
As an alternative, we could clone this repo into a specific directory of our choosing by adding a directory name as a parameter after the repo, like so:
$ git clone https://github.com/user/some-repo my-directory
$ cd my-directory
Can I Clone A Local Repository?
Usually, repositories are cloned from a cloud hosting service such as Github. However, it's also possible to make a clone of your local repository to another location locally.
Most of the time, you will find yourself cloning a remote repository, but there may be instances where you will need to clone a local repo as well. For example, let's say we have a repo located in c:\projects\cool-repo
that we'd like to clone into a directory called c:\beta
. We could do so with the following command:
$ git clone c:\projects\cool-repo c:\beta\cool-repo-beta
As you can see, cloning a repository is fairly straightforward, whether locally or from a remote origin. However, there are some details to be aware of when using the git clone command.
Does Git Clone Get All Branches?
Generally speaking, a branch is a label that references a specific series of commits. You may be wondering if git clone downloads every branch in a repository. The short answer is yes, Git clone does download all branches. However, this comes with an important caveat:
Every downloaded branch is designated as a remote tracking branch. However, only the master branch is checked out locally, which tracks a remote tracking branch called origin/master. Any of the other remote tracking branches can be checked out locally as needed, which will create the actual local copy of the branch.
To get a visual of this concept, we can use our previously cloned repo and view the branches found within. Assume this repo has three branches called master, v1.0, and v2.0:
$ git branch
* master
As you can see, we're only showing the master branch available locally. As previously stated, that's because master is checked out by default during the cloning process and therefore the local branch itself is created.
However, if you need to view a list of every remote tracking branch available for potential checkout, you would use the -a
option with git branch:
$ git branch -a
* master
remotes/origin/HEAD
remotes/origin/master
remotes/origin/v2.0
remotes/origin/v1.0
Git doesn't create a local branch for each remote tracking branch, but it does give us the ability to view them all and check one out if needed. Although there are ways to clone a repo and make a local branch for each remote branch, it's usually not recommended aside from a few specific scenarios.
A clone that creates a local branch for every remote branch can quickly result in stale tracking branches that are multiple commits behind the remote branch, which is why it's considered best practice to only checkout remote branches as needed.
For instance, if we needed to checkout the v2.0 remote branch to work on, we could accomplish this like so:
$ git checkout v2.0
Branch v2.0 set up to track remote branch v2.0 from origin.
Switched to a new branch 'v2.0'
Notice we didn't need to give the fully qualified name origin/v2.0
. As long as there's only one remote, Git will automatically find the correct remote branch and setup the local copy. If you're tracking more than one remote, you'll need to use the fully qualified name.
Adding Remotes to Your Git Clone
When working with teams, you may need to collaborate with individual team members on specific tasks. In this scenario, you may want to setup a remote to your teammate's repository. To do so, we can simply use the git remote
command with a few options and parameters, like so:
$ git remote add joe https://dev.team.com/joe.git
We can now collaborate outside the origin repository, which can really come in handy especially when working on large projects.
Interacting With Remotes From Within Your Cloned Repository
Once we've added a remote to our cloned repo, it's important to understand how to interact with it. For example, let's say we wanted to run a Git fetch command from Joe's repo that we added in the previous section:
$ git fetch joe
For fetch, along with pull and push, it's a simple matter of adding the remote name after the desired action.
Git Clone SSH
SSH (secure shell) is one of the URL protocols that Git uses to allow connections to remote repos. SSH is a highly secure authentication protocol, which means the user will need to provide credentials in order to connect to the host.
An example of a git clone using ssh:
$ git clone ssh://username@host.xz/absolute/path/to/repo.git/
Git Clone HTTPS
HTTPS (hypertext transfer protocol secure) is another URL protocol that can be used to connect to Git repos. In our previous examples, we used this method. Although SSH is considered more secure than HTTPS, Git currently recommends using HTTPS due to its ease of use. Additionally, HTTPS provides a more streamlined experience since you don't need to keep track of multiple SSH keys for each of your devices.
The only perceived disadvantage to git HTTPS is that you are required to enter your Git password whenever you push. Although this can be a slight inconvenience, the positives outweigh the negatives.
Git Clone Common Flags and Options
Git clone includes useful options and flags for directing your workflow. Let's have a look at some of the most common ones.
Cloning a specific branch
Git allows us to override the default behavior of checking out the master branch, and instead checkout a branch of our choosing:
$ git clone --branch <branch-name> <repo-url>
It's important to note that the --branch
option will still download every branch and designate them as remote branches.
If you would like to clone and download only the specified branch, simply add the --single-branch
flag like so:
$ git clone --branch --single-branch <branch-name> <repo-url>
As a quick note, -b
serves as a shorthand alias for --branch
.
Clone without tags
The git tag command is used to reference points in the repository's history that are then linked to specific versions or features. If you want to exclude these tags when using Git clone, you can use the --no-tags
flag, as follows:
$ git clone <repo-url> --no-tags
Clone specific number of commits
The --depth
flag allows us to limit the number of commits that are cloned to a specified amount:
$ git clone --depth=10 <repo-url>
This is useful for truncating exceptionally large repositories with an extensive commit history.
These are just a few of the many options available for git clone. For a complete list, check out the git clone docs.
Should I Clone Or Fork A Git Repository?
The main difference between git clone and fork is that a fork creates your own unique copy of a Git repository. Unlike a fork, a Git clone creates a linked copy that is always synchronized with the original.
So far, we have seen that git clone creates a linked copy of a Git repository. Once cloned, developers are still linked to the target repo via remote tracking branches, and able to remain synchronized by pulling, fetching, and merging changes. Using Git clone is ideal when you'd like to contribute to a shared code base with other developers while keeping track of and collaborating on the changes made by everyone involved.
In contrast, a fork creates an unlinked, or standalone copy of the target repo that doesn't include remote tracking to the target repo. Git fork is a better choice if you'd like to make an isolated copy of a code base to make it into something different. Of course, your forked repo could then be cloned by others and collaborated on as a group or team.
Summary
If you are trying to expand your developer skillset, then a mastery of Git is essential. The git clone
command is a helpful tool that allows you to make a local copy of a remote repo. The advantage of a cloned repository is that it remains synced to the target repo via remote tracking, allowing developers to stay up to date with changes made by other developers working on the same code base.
A clone is basically an exact replica of the target repo. As shown above, by default git clone
checks out and tracks the master branch from the target repo, but all other branches can be checked out as needed. The default behavior can be overridden by using available options with clone such as --branch
and --depth
.
Overall, git clone
is an essential tool to add to any developer's workflow. If you want to put what you have learned into practice, try cloning an interesting repository from Github and playing around with some of the commands we have discussed in this article.
Next Steps
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this, we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- Git SCM Docs, git clone - https://git-scm.com/docs/git-clone
Final Notes
Recommended product: Decoding Git Guidebook for Developers