Git Branches | Git List Branches & Other Branch Commands
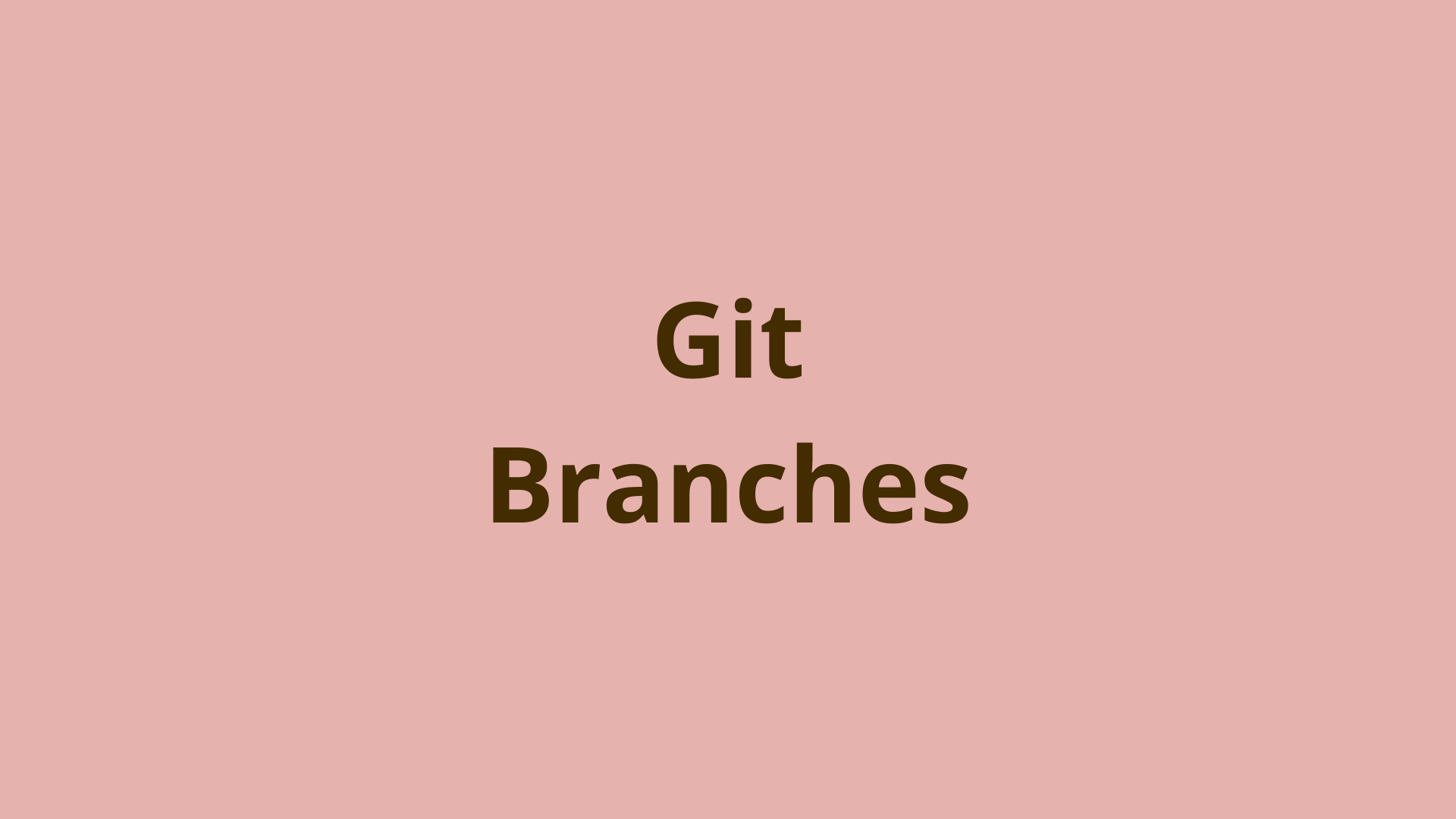
ADVERTISEMENT
Table of Contents
- Introduction
- What are Branches in Git?
- Why are Branches Used in Git?
- Create a New Branch
- How Many Branches are there on Git?
- What is a Remote Branch in Git?
- How Does Git Remote Branch Work?
- Git List Branches
- How Do I List a Remote Branch?
- Git List All Branches
- How Do I View Branches in GitHub?
- Git Delete Branch
- How Do I Know Which Branch I Branched From?
- Common Git Branching Workflows
- Git Flow
- Next Steps
- References
Introduction
A large part of working with git involves collaborating with other developers. To work effectively an understanding of git branches is required. In this article, we'll explain what a branch is in Git, how to create and delete branches, and different strategies for working with Git branches.
What are Branches in Git?
In Git, a branch is a label that identifies a series of commits that are connected to each other. Each of the commits in a branch references its parent commit, except the initial commit, which has no parent commit.
A Git branch is really more of a concept than an actual data structure. A branch is a type of "ref" in Git. It is really just a label that points to a specific commit.
As you make new commits, Git repoints the branch name to the new commit, so a branch is basically a ref (label) that always points to the tip of the chain of commits you're working on.
All Git repositories in which at least one commit has been made have at least one branch, which is named the master
branch by default. As we'll see later, it is commonly renamed to main
these days.
When you create your first commit, Git sets a reference (ref) point called HEAD, which always points to the commit ID of the commit currently checked out in your working directory. As you make additional commits, HEAD is reassigned so that it always points to the latest commit. Order is maintained because each commit also references the commit that came before it (commonly referred to as its parent).
Why are Branches Used in Git?
Developers these days tend to work in teams. This means that multiple developers often work on the same codebase at the exact same time. Branches allow developers to isolate their changes in various ways, which can be important for the developers and organizational workflows:
- Different environments, such as Development, Testing, and Production are often isolated to different branches.
- In some workflows, a new branch is created for each new feature, these are referred to as "feature branches".
- Individual developers can easily create their own local branches to make changes without worrying about interference from other devs.
Create a New Branch
As previously mentioned, Git has a lightweight branching model. This simply means that a Git branch is really just a label that points to a specific commit - there is no new data structure in Git that represents a branch. This encourages frequent branch creation in many development workflows.
You can use the git branch command to create new branch in your Git repository:
$ git branch <new-branch-name>
Before you can use the newly created branch you need to switch to it. Switching to a branch is referred to as checking out a branch:
git checkout <branch-name>
Lastly, since creating a branch and checking it out is such a common task, Git provides a useful shortcut to create a branch and check it out in a single command:
$ git checkout -b <new-branch-name>
How Many Branches are there on Git?
When you first initialize a repository a create your first commit, you will be on a branch called master
by default. This means at minimum you will always be working with one branch. A common modern day trend is to use a branch name of main
rather than master as the primary branch.
You can make Git rename your local master
branch to main
as follows:
$ git branch -m master main
If you need to sync that up with your remote repository, you can use the following commands:
$ git push -u origin main
$ git push origin --delete master
If you get an error, you may need to log into GitHub and change the default branch to main
before you delete the master branch. This should allow you to run the previous commands and delete the master branch in your remote repository.
What is a Remote Branch in Git?
For most local branches that you work on, you'll want to sync up those changes with a copy of the branch in a remote repository. The remote repository is usually hosted on a platform like GitHub or BitBucket. Branches are synced between your local repo and the remote repo using the git pull and git push commands.
When synced with a remote, each of your local branches will have a corresponding remote tracking branch. You can think of a remote tracking branch as a copy of your local branch that is used to determine if you're in sync or out of sync with the remote of the branch.
Git handles most interactions with the remote tracking branches automatically, so you'll never have to merge or interact with one directly.
How Does Git Remote Branch Work?
When working with a tool like GitHub you will be working with branches that are hosted on a remote web server. One method to create branches is to do so directly from the GitHub interface. Then you can use git fetch
in your local repo to download all the branches associated with that remote repository, create remote tracking branches in your local repo for all those branches, and sync them up to local copies of those branches.
Alternatively, you can create a new branch in your local Git repo and push it to the remote using the command:
$ git push -u origin <branch-name>`
In this command, the -u
(or --set-upstream
) flag tells Git to sync your local branch to a remote copy. The origin
represents the default remote repository you're communicating with.
Git List Branches
It is very common for Git repositories to have many branches. This is especially true for large projects with large development teams that have been around for a long time. Therefore, it is important to keep track of branches and make sure you are not working on the wrong branch. Git provides a number of useful commands to help you list branches and keep track of branches.
To view the current branch you are working on you can simply use the git status
command. This command shows a lot of useful information about your current changes including what branch you are on:
$ git status
On branch main
Your branch is up to date with 'origin/main'.
The command git branch
when run with no flags will list all local branches:
$ git branch
*main
develop
test
feature1
Note the asterisk in the git branch output identifies the currently checked out branch in your local Git repository.
How Do I List a Remote Branch?
You can use the git branch -r
to make Git list remote branches that have been synced to your local repository:
$ git branch -r
origin/main
origin/develop
origin/test
origin/feature1
Note that since these are remote tracking branches, these branches are listed in the format origin/branchname
. These remote tracking branch refs can actually be found in your filesystem within the hidden .git
directory at the path .git/refs/remotes/origin
:
$ ls .git/refs/remotes/origin
main
develop
test
feature1
Git List All Branches
You can make Git list local branches and remote branches in your Git repository using the command git branch -a
:
$ git branch -a
*main
develop
test
feature1
origin/main
origin/develop
origin/test
origin/feature1
How Do I View Branches in GitHub?
You can view all your remote branches in the GitHub interface if you want to directly check what is set up there. Simply navigate to a Git repository and by default you should be on the master (or main) branch. You should see a fairly conspicuous dropdown menu that you can click on to list all branches in GitHub.
Git Delete Branch
It's often good practice to have Git delete local branches if they are no longer in use or if they've already been merged into other branches you're still using.
If you have already merged your branch you can use the -d
flag to delete the Git branch:
$ git branch -d <branch-name>
An warning will be displayed if you try to delete a branch that isnβt merged, or if you try to delete the branch that is currently checked out in the working directory.
If you see the Git error message Cannot delete branch 'Branch name' checked out at '[directory]'.
just check out a different branch and try to delete the previous branch again.
If you see the Git error message The branch 'Branch name' is not fully merged. If you are sure you want to delete it...
that simply means you haven't merged the changes in that branch yet, so you could lose data if the branch is deleted.
If you want to delete the unmerged branch anyway and are OK with losing any data in the process, you can use the -D
flag:
$ git branch -D <branch-name>
To propagate the branch deletion to a remote repo or delete a branch in the remote, you can use the command:
$ git push origin --delete <branch-name>
How Do I Know Which Branch I Branched From?
In general it is not possible to determine the branch or commit that a particular new branch was created from. The reason is that a branch is just a label that references a specific commit based on its commit ID.
This label moves forward each time Git records a new commit on the branch. But there is no original trace of the branch label on each commit that the label was applied to in the past. Once Git moves the branch ref to a new commit, there isn't a consistent and reliable way to determine which commits had the branch ref applied in the past.
Common Git Branching Workflows
A development team's branching strategy determines the number of branches, naming convention, and storage policy for the branches in Git repository. Two commonly used Git branching models include Git Flow and Trunk Based Development.
Git Flow
In its simplest form, Git Flow makes use of two long running branches called master
and develop
, which initially are exact replicas of each other. Feature branches and Bugfix branches are then made from the develop
branch before being merged back into develop
.
A release branch is then made from the develop branch that eventually gets merged into master
when a new release of the software is ready for release to production.
A successful Git branching model has an excellent illustration of this process. It also includes hotfix branches, a type of branch that is designed to correct major bugs that made it into production.
Trunk Based Development
A more modern approach to software development focuses on more continuous deployment. For small teams trunk based development allows all developers to work on the master branch and commit directly to master
. In this model, builds are consistently occurring and required to pass a set of tests before the changes are deployed to the production environment.
Scaled Trunk Based Development
Larger teams who want to use trunk based development use a scaled variation to help manage their code base. The only difference here is that developers will branch off of master
before merging back in. This helps prevents the large number of commits from directly interfering with each other on a regular basis.
Next Steps
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- GitHub - https://github.com
- Git SCM, Git Branching - Remote Branches - https://git-scm.com/book/en/v2/Git-Branching-Remote-Branches
- Vincent Driessen, A Successful Git Branching Model - https://nvie.com/posts/a-successful-git-branching-model/
Final Notes
Recommended product: Decoding Git Guidebook for Developers