Python Getattr() Method – How it Works & Examples
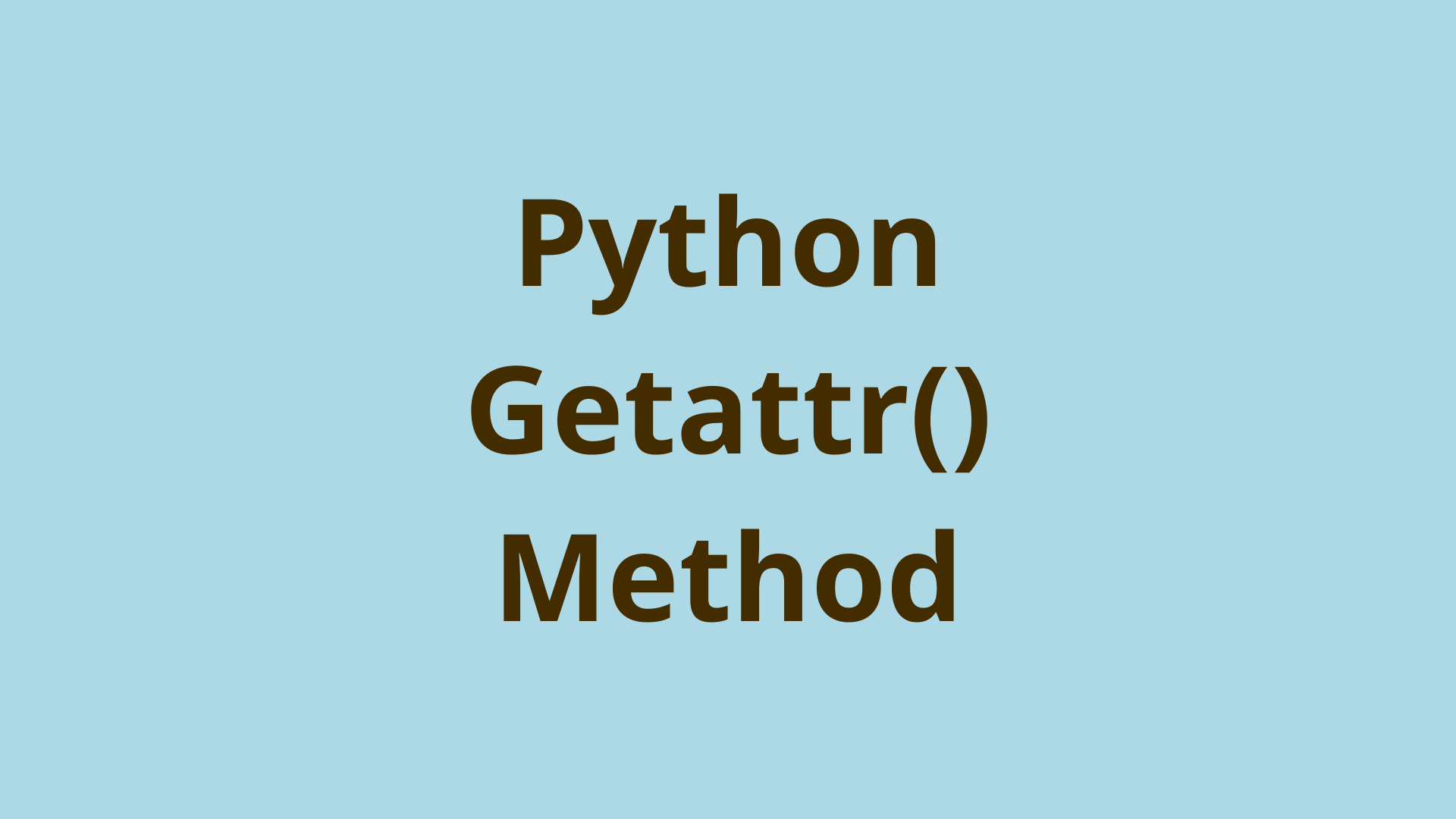
ADVERTISEMENT
Table of Contents
- Introduction
- What is a Python attribute?
- Examples of attributes
- What does Getattr () do in Python?
- Does Getattr return methods?
- What is __getattr__?
- What is the difference between __getattr__() and __getattribute__?
- How do you print all attributes of an object in Python?
- Summary
- Next Steps
- References
Introduction
Python is a powerful programming language that offers a wide range of built-in functions and methods to perform various tasks efficiently. One of these built-in functions is getattr(), which lets you get an attribute from an object. It has several features which make make it extremely useful in specific use cases.
In this article, you will learn about the getattr() function in Python, the __getattr__ and __getattribute__ special methods, and the dir() function. These concepts will take your knowledge of Python attributes to the next level.
What is a Python attribute?
An attribute in Python is used to describe a characteristic of an object, and has the syntax object.attribute
. Classes, instances, and functions can all have attributes.
Class attributes are shared across all objects and can also be accessed directly from the class variable. Instance attributes are unique to each instance of a class and are defined inside the constructor of the class, also known as the __init__ method. Function attributes are simply variable names defined inside functions with the syntax function_name.attribute
.
Examples of attributes
class Tree:
apple = 1
>>> Tree.apple
1
def func():
func.x = 2
>>> func()
>>> func.x
2
Note, that to access the function attribute outside the function, you must first call the function.
What does Getattr () do in Python?
The getattr() is a built-in Python function that allows you to get the value of the attribute of an object. Unlike the regular object.attribute
syntax used to get the value of an attribute, the getattr() function takes the attribute name as a string input. This could be just the tool you need for a specific use case.
The getattr() function has three arguments:
- object : This is the object which contains the attribute
- name : The name of the attribute in string format
- default : An optional argument, whose value is returned when the attribute name does not exist in object.
Should you use Getattr?
Here is an example of a good use case of getattr():
class House:
room = "living room"
>>> getattr(House, "room")
'living room'
>>> House.room
'living room'
Getting the value of the room returns the same value in both cases returns the same value. However, as you can see the getattr() function takes the attribute name "room" as a string.
A scenario where this might be useful is if you ask the user for the attribute name using the input() function. If you were to use the regular way, you would have to define multiple if statements for each attribute, which would make your code very lengthy for a large number of attributes. Whereas, with the getattr() function you can achieve this simply in one line:
>>> attr_name = input("Input an attribute name: ")
Input an attribute name: room
>>> getattr(House, attr_name)
'living room'
In the same scenario, the user could input an attribute name that is not an attribute in our object House. With getattr() we can use the default argument:
>>> attr_name = input("Input an attribute name: ")
Input an attribute name: abc
>>> getattr(House, attr_name, f"{attr_name} does not exist in object House")
'abc does not exist in object House'
Here you can see how getattr() can make your life easy for this specific use case.
Does Getattr return methods?
The getattr() function is not just limited to simple object types like string or int, you can also get attributes that are methods/functions in a class and then use those methods. For example:
class Tree:
def get_apple(self):
return "apple"
>>> tree = Tree()
>>> apple_method = getattr(tree, "get_apple")
>>> apple_method()
'apple'
First, you have to define a class containing a method, here we define the class Tree with the get_apple method. Then, you can get an instance of the class. Finally, you can use the getattr() function to get the get_apple method and assign it to the apple_method variable. This allows us to easily use the get_apple method with the apple_method variable.
What is __getattr__?
Although __getattr__ has a name similar to getattr(), its purpose is a bit different. __getattr__ is a special method in Python that is exclusively called when an attribute is accessed that does not exist in the instance. For example:
class Tree:
apple = 5
leaves = 100
def __getattr__(self, name):
return f"Sorry, \"{name}\" does not exist!"
>>> t = Tree()
>>> t.apple
5
>>> t.app
'Sorry, "app" does not exist!'
>>> getattr(t, "app", "not found!")
'Sorry, "app" does not exist!'
>>>
We have a Tree class with variables apples and leaves, and we define the __getattr__ method to return a string message if an attribute is not found. Note that the name in __getattr__ is the name of the attribute.
Next, we get an instance of Tree, t. Getting the attribute apple from t normally returns the value of apple. However, trying to get the value of the non-existent attribute app invokes the __getattr__ method and returns our custom message instead of an exception.
Ultimately, I try getting the "app" attribute with the getattr() function with a default value. You can see that the __getattr__ method overrides the default value in the getattr() function.
What is the difference between __getattr__() and __getattribute__?
There is another special method called __getattribute__, which could cause confusion, so we will discuss how they are different.
The __getattribute__ method is invoked every time you get an attribute from an instance. Even if this object exists or doesn't exist. Let's see an example to make this clear:
class Tree:
apple = 5
leaves = 100
def __getattribute__(self, name):
print("In __getattribute__")
value = object.__getattribute__(self, name)
return f"The value of {name} is: {value}"
def __getattr__(self, name):
return f"Sorry, \"{name}\" does not exist!"
>>> t = Tree()
>>> t.apple
In __getattribute__
'The value of apple is: 5'
>>> t.app
In __getattribute__
'Sorry, "app" does not exist!'
We have the same class Tree again, but this time we have a __getattribute__ method with a print statement inside. The value = object.__getattribute__(self, name)
line prevents an infinite loop and lets us simply get the raw value of the attribute.
When we get the apple attribute, it invokes the __getattribute__ method and returns the customized output. Even when we try to get the app attribute, the __getattribute__ still invokes first (as evident from the print statement) and then the __getattr__ invokes.
How do you print all attributes of an object in Python?
A really handy function to know in Python is the dir() built-in function. The dir() function lists all the attributes an object has. For example:
>>> import math
>>> dir(math)
['__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'comb', 'copysign', 'cos', 'cosh', 'degrees', 'dist', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'isqrt', 'lcm', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'nextafter', 'perm', 'pi', 'pow', 'prod', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc', 'ulp']
>>>
You can see that the dir() function gives you a list of all the various functions supported by the math module.
Of course, this can be very useful when working with the getattr() function. But many times using the dir() function with a new module can give you very useful insight into what the module can do before you even go and look at the documentation.
Summary
In this article, you learned about the getattr() function and special methods which can allow you to modify how attributes are accessed in your class.
First, you briefly went over what attributes are in Python and how they work. Next, you learned what the getattr() does, its syntax, and a scenario where it can be useful. Then you further explored how the getattr() function can even return class methods. You followed that by understanding what the __getattr__ and __getattribute__ special methods do, to make sure you don't confuse them with the getattr() function. Eventually, you saw what the dir() function is and how it can be very useful.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Python Offical Documentation - getattr() - https://docs.python.org/3/library/functions.html#getattr
- Python reference - getattr - https://python-reference.readthedocs.io/en/latest/docs/functions/getattr.html
Final Notes
Recommended product: Coding Essentials Guidebook for Developers