Python Reserved Keywords (Full List) | Initial Commit
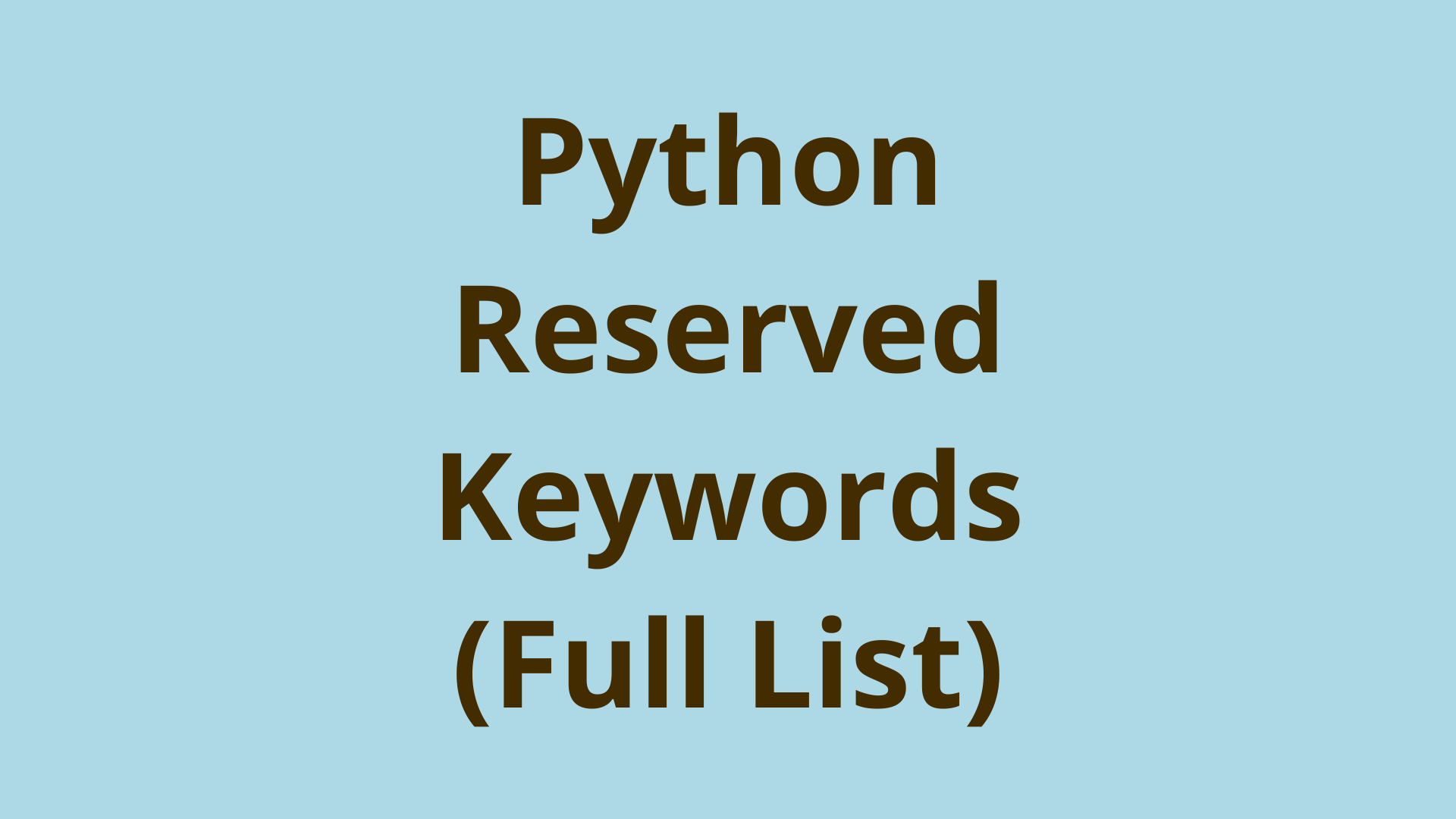
ADVERTISEMENT
Table of Contents
- Introduction
- Reserved Keywords in Python
- What do Keywords do in Python?
- assert
- break
- def
- else
- for
- Is List a reserved word in Python?
- Is TODO a reserved word in Python?
- Is While a reserved word in Python?
- Is concat a reserved word in Python?
- What are the 33 reserved words in Python?
- Summary
- Next steps
- References
Introduction
Python, like all other programming languages, has a base set of rules. These rules are known as the syntax of any given language. Python has multiple rules and structures, ranging from text spacing to reserved keywords. Here, we'll look at the words and see how they function in relation to the rest of Python's syntax.
Reserved Keywords in Python
Below is a table of the 35 reserved keywords in Python. According to the documentation, "The following identifiers are used as reserved words, or keywords of the language, and cannot be used as ordinary identifiers." This also means that these words cannot be spelled incorrectly.
and | def | global | or | False |
as | del | if | pass | True |
assert | elif | import | raise | None |
async | else | in | return | |
await | except | is | try | |
break | finally | lambda | while | |
class | for | nonlocal | with | |
continue | from | not | yield |
For further information on a specific keyword, click the word and you will be directed to the corresponding part of the article.
What do Keywords do in Python?
As mentioned before, keywords are just one part of Python's syntax. They serve to perform a static and defined purpose as created by the developers of Python. In this way, keywords provide programmers with simple but effective tools that can be used for simple or complex design patterns. This section will delve into the details of each keyword more thoroughly.
and
<arg1> and <arg2>
The and
keyword is an operator that determines whether two arguments are logically equal. The output, should both sides be [logically] unequal, will be <arg1>
. Should the opposite occur, <arg1>
will be the output.
as
import InnitialCommit as init_commit
When importing modules or libraries, the as
keyword serves to apply an alias to these imports. Rather than having to deal with a library or module's default name, one can assign an alternate name with the as
keyword.
assert
assert <expectation>
For debugging purposes, the assert
keyword is quite useful. It allows a programmer to set a certain expectation for a program and test output against that expectation.
async
async def tick():
# Code for tick function
The async
keyword is paired up with the def
keyword. This is because async
acts as a modifier to the function's definition. Any function defined with async
will thusly become asynchronous.
await
int time_passed = await tick()
This keyword, await
works in conjunction with asynchronous functions. When used, await
allows the event loop to take control of the mentioned function and await its results.
break
while True:
print("Initial Commit")
break
From dictionary mapping to statements to loops, the break
keyword serves a vital purpose. This keyword allows a programmer to break out of the current scope of code the program is running in. Observing the example above, the loop is not infinite since after "Initial Commit" is printed, the break statement brings the program outside the loop's scope.
class
class ExampleClass():
example_x = 0
example_y = 0
The class
keyword allows a programmer to create their own class object.
continue
for commits in commit_array:
if <expression>:
continue
Iterating through loops can be made more efficient with the continue
keyword. Should this keyword be used, the loop will cease operating on the current iteration and continue on to the next one.
def
def my_function(num):
return num + 1
The def
keyword gives a function or class its programmer-made definition. It can be used alongside async
in order to create asynchronous functions.
del
dict = {1: "Bob", 2: "Annie", 3: "Mark"}
del dict[1]
The del
character can be used to delete items from a list or dictionary. It also can unset variables but is commonly used for the purpose stated above. In the example, the use of del
will remove number 1, "Bob", from the dictionary of names.
elif
if <expression1>:
# Do something
elif <expression2>:
# Do something different
The elif
keyword must be used after an if is used. The elif
serves to outline a secondary course of action should the first if-statement not be triggered. The elif
is Python's version of the else if
keyword in languages like Javascript.
else
if <expression1>:
# Execute here
elif<expression2>:
# Execute here
else:
# Execute here
An else
keyword defines a block of code that is executed after all expressions in the above if
and elif
statements are false. The elif
needs no expressions or conditionals.
except
try:
my_file = open("InnitialCommit.txt", r)
except:
print("File was unable to be read")
The except
keyword works as the latter half of a two-part procedure. The combination of try
and except
allow programmers to handle exceptions in a graceful manner. Similar to elif
, a programmer may use the except
keyword as many times as needed in relation to the try
expression.
finally
try:
my_file = open("InnitialCommit.txt", r)
except:
print("File was unable to be read")
finally:
print("This happens no matter what.")
Opposite to the philosophy of the else
statement, the finally
keyword will carry out its code no matter the evaluation of the try
and except
blocks that came before it.
for
for item in list:
print(item)
The for
keyword serves to instantiate a for-loop. It can be combined with other keywords like in
and range
to customize the parameters of the loop.
from
# from <module> import <specifics>
from InnitialModule import InnitialCommit
The from
keyword works in conjunction with the import
keyword to allow users to bring in specific tools and libraries found outside the current project. The use of from
gets a general library or toolset while import
specifies which particular tool or class is needed.
global
def example_function():
global PI = 3.14
The global
keyword allows a variable to exist within the scope of the entire program rather than just existing within a function or class. In the example above, the variable PI is viewable outside the scope of "example_function".
if
if <expression>:
# Do something
The if
keyword is one of the most used across Python and other languages as well. It allows the programmer to create a conditional statement that executes code depending on the expression's outcome.
import
import <module>
Python's import
keyword brings the specified module or library into the program, thus allowing for further functionality and optimization.
is
x = True
y = True
print(x is y)
# Will print True, since X and Y have are the same object in memory
The is
keyword checks the types of two arguments. This operator returns True if the two arguments are of the same type in memory and False otherwise.
lamda
x = lambda y: y + 1
print(x(1))
# Will print 2
The lambda
keyword specifies an anonymous function with one statement, otherwise known as a lambda function.
nonlocal
nonlocal <variable>
Similar to the global
keyword, nonlocal
specifies a scope for a variable. In this case, the variable being addressed belongs to the parent scope.
not
my_bool = True
my_other_boo = not my_bool
The not
keyword gets the opposite boolean value of the argument passed to it. In the example above, "my_other_bool" is equal to the opposite of "my_bool", or False.
or
<expression1> or <expresion2>
In Python, or
will return the first expression if True
and returns the second expression if False
. Where and
determines if both expressions are True, or
determines if at least one expression is true.
pass
def example():
pass
A pass
is a no-op. It tells the Python interpreter that no operations are taking place in a given block of code. Similar to commenting out code, instead of ignoring the block outright, that block is still interpreted but never given any tasks to execute.
raise
raise <exception>
If you wish to raise an exception in Python, use the raise
keyword.
return
def my_function():
return "Initial Commit"
The return
keyword is simple. Given a function defined with def
, return
tells Python to stop executing code in the function block, and instead return back to the main event loop with the function's results.
try
try:
my_file = open("InnitialCommit.txt", r)
except:
print("File was unable to be read")
The try
keyword is the first part of the try/except block. The try
keyword indicates that the following code may result in an exception. The except
keyword actually handles that exception, if it comes up.
while
while <expression>:
# Do something
The while
keyword is used to instantiate while loops in Python.
with
with <context manager> as <variable>:
# Code to be executed
In Python, the management of resources is handled by Context managers. The with
keyword allows a programmer to specify which context manager is needed for a procedure.
yield
def iterable_function():
yield 1
yield 2
yield 3
The yield
keyword acts similarly to the return
keyword. However, where return
produces a function's output once, yield
can output a function's output many times via a generator. In the example above, each call to "iterable_function()" using next()
will result in each yield value being returned until the function can return nothing else. Though yield
and return
may seem synonymous, there are further differences between the two.
False
x = False
A boolean value of False
True
x = True
A boolean value of True
None
x = None
Like null or undefined in other programming languages, Python's None
keyword represents no value.
Is List a reserved word in Python?
Constructs such as list and dict are better known as, "Data Structures" in Python and many other languages. As the name implies, data structures are used for the storage and maintenance of data. Given their defined responsibility to store data, words like list
and dict
are not keywords in Python.
Is TODO a reserved word in Python?
It can be confusing sometimes to look at comments in code and see that a word is highlighted. This can often be the case with the TODO
comments. These may seem to be keywords, but the fact is that many IDEs (Integrated Developing Environments) are just pulling a clever trick.
When working on code, one might find themselves wanting to integrate something but not having the time for it. In this case, many IDEs actually highlight TODO
comments so that the programmer will be more aware of unimplemented features. In short, TODO
is not a keyword, but a flashy reminder.
Is While a reserved word in Python?
The while
keyword is one of the most frequently used in many programming languages, including Python. It allows for incredibly simple or complex loops while being easy to understand in the process.
Is concat a reserved word in Python?
The use of concat
may sometimes be confused for a Python keyword. In actuality, concat
is actually a function of the pandas library; a third-party library focused on data structures and data analysis. It is not associated with any of Python's reserved keywords.
What are the 33 reserved words in Python?
With the release of Python 3.5 in 2015, async
and await
were added to Python's keywords library, bringing the total amount of keywords up from 33 to 35. As of this article's writing, current releases of Python still contain 35 keywords.
Summary
We have looked at the reserved keywords in Python and their use syntactically speaking. Though there are 35 keywords, some like with
, nonlocal
, and lambda
are situational and may rarely be used depending on the programmer. Then there are reserved keywords like if
, try
, and for
that see extensive use across all Python programmers. In totality, the keywords of Python give the programming language a lot of built-in functionality that some people might ignore beyond the basics, and this should be taken advantage of. There's no need to reinvent the wheel, if there's a problem in your code that could be solved with the use of a keyword, try using it.
Next steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Python Keyword Documentation - https://docs.python.org/3/reference/lexical_analysis.html#keywords\
- Asynchronous Functions - https://realpython.com/async-io-python/
- Yield vs Return - https://initialcommit.com/blog/python-yield-vs-return
- Context Managers - https://www.geeksforgeeks.org/context-manager-in-python/
- pandas - https://pandas.pydata.org
Final Notes
Recommended product: Coding Essentials Guidebook for Developers