Python RuntimeError (Examples & Use Cases) | Initial Commit
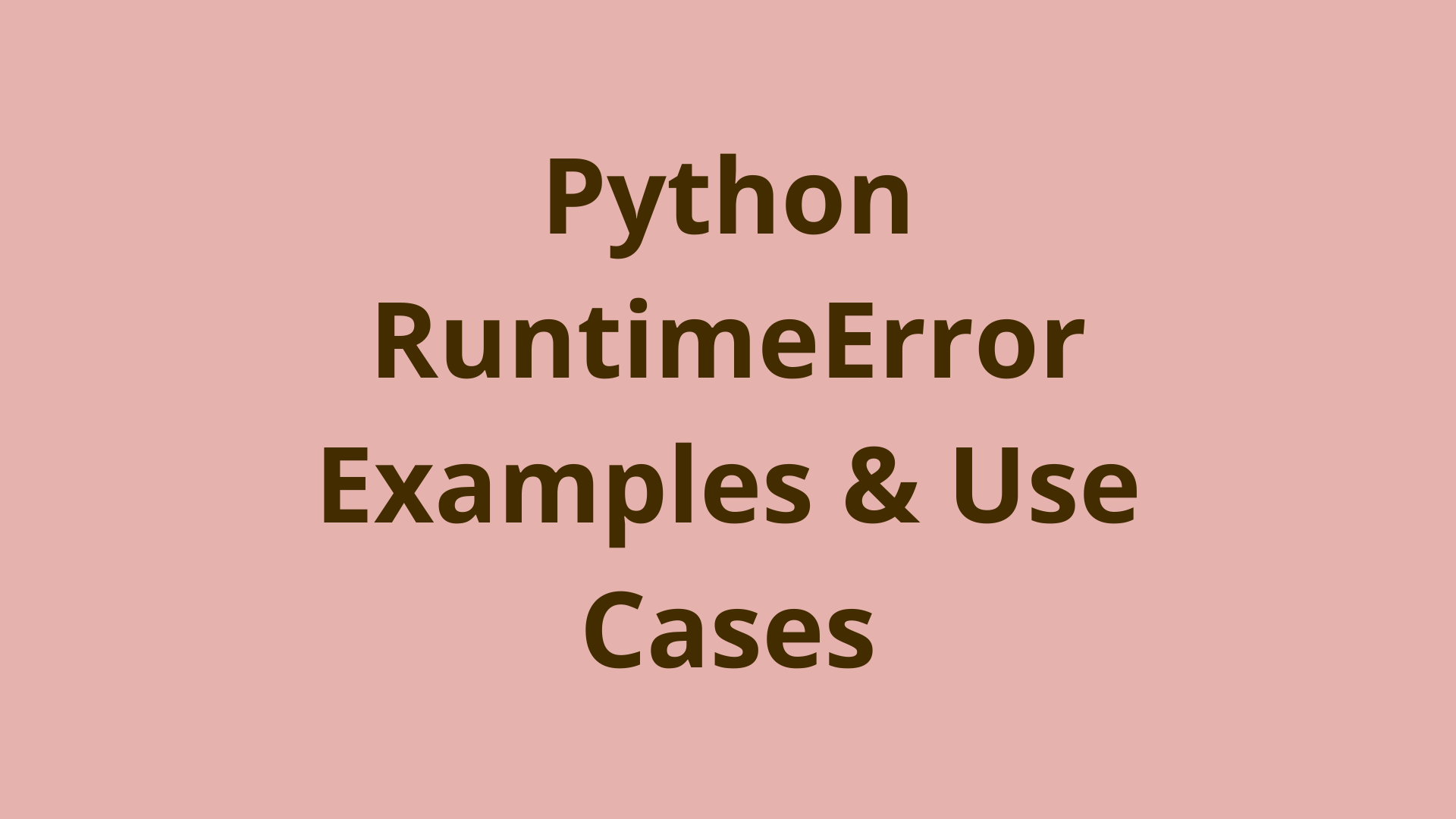
ADVERTISEMENT
Table of Contents
- Introduction
- What is a Runtimeerror error?
- What causes run time error message in Python?
- How do you handle a runtime error?
- What is runtime error message example?
- Zero Division Error raised
- Index Error
- How do I fix a built-in exception value error in Python?
- What causes the built-in exception overflow error?
- Summary
- Next steps
- References
Introduction
There are several types of errors in Python that help you debug your program. When you run your program, Python first checks for any syntax errors, and then runs the program. Errors thrown during syntax checking are called syntax errors. Errors that are thrown once the code is run are under the class of runtime errors. These errors will always include an error message printed to the interpreter. In this tutorial, you will learn several different types of runtime errors in python, what their error messages are, and how to fix them.
What is a Runtimeerror error?
A python run-time error is a class of python built-in exceptions that are thrown at run-time. There are many different types of run-time errors, we will cover them in this article. Some examples include type errors and name errors. run-time attribute errors fall under a larger group of concrete built-in exceptions in Python.
What causes run time error message in Python?
There are many things that can raise a runtime errors in python depending on the type of error that is thrown. Some questions you can ask yourself to debug including, are you:
- dividing by zero?
- using incorrect types in operations?
- indexing past the length of a list?
- accessing a key in dictionary that doesn't exist?
- using variables that haven't been defined yet?
- trying to import or access a file that does not exist?
If you checked for all of these things and you are still getting an error, then it may be caused by something else. We will cover some examples of causes and fixes of certain errors in the following sections.
How do you handle a runtime error?
How you handle a run-time error in python depends on the specific error that was thrown. Once way to generally handle run-time errors is by using a try/except block. You include the code that may cause a runtime error in the try clause. You can then specify how you want to handle the code in the case of an error in the except clause. Here is an example:
>>> y = 12
>>> x = 0
>>> try:
... z = y / x
>>> except:
...print("An error occurred")
...z = 0
This way you are alerted of the error without causing a raised exception and stopping your program.
What is runtime error message example?
Every run-time error in python prints a message to the interpreter indicating what caused raised error and what line it was on. Let's take a look at some examples of common run-time errors and how to fix them.
Zero Division Error raised
A ZeroDivisionError is thrown when you try to divide or modulo by zero in your code, for example:
>>> x = 0
>>> y = 2
>>> if y % x == 0:
... print(y, :is divisible by", x)
Traceback (most recent call last):
File "<stdin>", line 3, in <module>
ZeroDivisionError: integer division or modulo by zero
Note that the error message idicates the error is happening on line 3. We can avoid this by adding a check to make sure the number we're modding by isn't 0 before we perform the operation:
>>> x = 0
>>> y = 2
>>> if (x != 0) and (y % x == 0):
... print(y, "is divisible by", x)
Type Error
A TypeError is thrown when you try to perform an operation with incorrect types. For example, if you tried to concatenate an int and a string, you get a type error:
>>> x = "1"
>>> y = 2
>>> z = x + y
Traceback (most recent call last):
File "<stdin>", line 3, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'str'
The error will also specify which operation is throwing the error and what types you're trying to use. In this case we can resolve this error by casting all the variables to integers to make sure we are only adding integers:
>>> x = "1"
>>> y = 2
>>> z = int(x) + int(y)
>>> print(x+y)
3
Another common cause for type errors is attempting to loop through an incorrect type. For example, attempting to iterate through an integer:
>>> for i in 10:
... print(i)
Traceback (most recent call last):
File "<stdin>", line 3, in <module>
TypeError: 'int' object is not iterable
In this case the error tells us that we are attempting to iterate through an integer on line 3, which is not a type we can iterate through. Instead we want to use a for-range loop:
>>> for i in range(10):
... print(i)
0
1
One example of a library function that can throw a type error is the pandas.head()
method from the Pandas library. The head function throws a type error if you provide an argument that is not an integer:
>>>df.head(2.5)
TypeError: cannot do positional indexing on RangeIndex with these indexers [2.5] of type float
You can learn more about the pandas head function here.
Key Error
A KeyError is thrown when you are trying to access a key in a dictionary that doesn't exist. For example:
>>> d = {1: ["a"], 2: ["b"]}
>>> print(d[3])
Traceback (most recent call last):
File "<stdin>", line 2, in <module>
KeyError: 3
The error will specify which key you are trying to access that does not exist. You can fix this by making sure to check if the key is in the dictionary before trying to access that specific key. You can check if a key exists in a dictionary by using the in function, which returns a boolean:
>>> d = {1: ["a"], 2: ["b"]}
>>> if 3 in d:
... print(d[3])
Index Error
An index error is caused by indexing into a list with an out of bounds index:
>>> L = [1,2,3]
>>> for i in range(10):
... print(L[i])
We can fix this by either adjusting the for loop to only loop over the range of the list, or adding a check before we index into the list to make sure the index is in range:
>>> L = [1,2,3]
>>> for i in range(len(L)):
... print(L[i])
or
>>> L = [1,2,3]
>>> for i in range(10):
... if i < len(L):
... print(L[i])
Name Error
A NameError is thrown when you try to use a variable that is not defined yet in the file. For example:
>>>print(x)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'x' is not defined
You can fix this by defining the variable on a line before the line it is called on:
>>>x = 5
>>>print(x)
5
Name errors can also be thrown if you try to use a variable that is already defined but out of scope. For example, if you try to use a local variable defined inside of a function globally:
>>>def foo(x,y):
print(x + y)
>>>
>>>print(x)
Traceback (most recent call last):
File "<string>", line 4, in <module>
NameError: name 'x' is not defined
To fix this, make sure you are only trying to access variables that are within scope.
File not found error
A FileNotFoundError is thrown when you try to access a file that does not exist in the directory you specified:
>>> open("file", "r")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
FileNotFoundError: [Errno 2] No such file or directory: 'file'
There are a couple ways to resolve the error. First you should check to make sure that the file you are trying to access is in your current directory. If not, make sure to specify the full filepath when trying to access that file, for example:
>>> open("/C:/folder1/folder2/file", "r")
How do I fix a built-in exception value error in Python?
A value error is caused by giving a function an input that is of the correct type, but an incorrect value. For example:
>>>import math
>>>math.sqrt(-100)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: math domain error
Here we get an error because the math.sqrt function only works with positive integers or zero as input. We can prevent this error by making sure that anything passed into the function is greater than or equal to zero.
What causes the built-in exception overflow error?
An overflow error is caused by an arithmetic operation that is too large for Python to represent.
For example:
>>>math.exp(5000)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
OverflowError: math range error
You can prevent overflow errors by making sure that the numbers you pass into functions are not too large.
Summary
In this tutorial, you learned that run-time errors are, when they occur in your program, and several ways to fix them. First, you learned that run-time errors are a type of built-in exceptions thrown at run-time. Next, you learned some common causes of run-time errors like dividing by zero and using incorrect types in operations. Then, you learned how to generally handle run-time errors using a try-except block. Last, you looked at several examples of things that can raise run-time errors and how to resolve them, including some less common errors like overflow errors and value errors.
Next steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
Final Notes
Recommended product: Coding Essentials Guidebook for Developers