Assignment Operators in Python
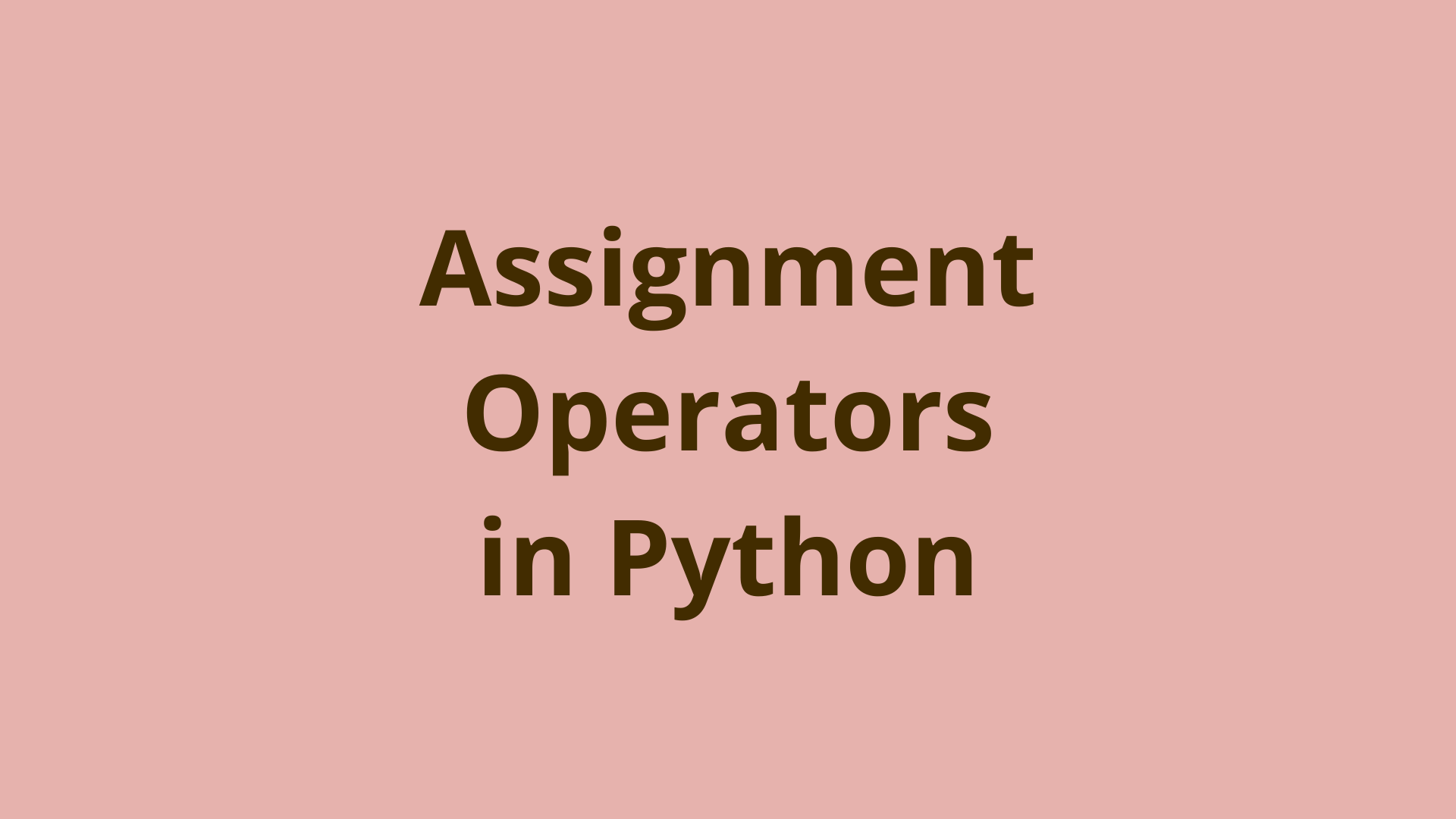
ADVERTISEMENT
Table of Contents
- Introduction
- What are the Assignment Operators and why would you use them in Python?
- How do you use the Assignment Operators in Python and what are the benefits of doing so?
- What are the benefits of using the assignment operators in Python?
- What are the potential drawbacks of using the Assignment Operators in Python?
- When is it best to use the Assignment Operators in Python?
- When is it not recommended to use the Assignments Operators in Python?
- Is there ++ in Python?
- Why isn't there ++ and -- in Python?
- Summary
- Next Steps
Introduction
Whether you are an old hand or you are just learning to program, assignment operators are a useful tool to add to your programming arsenal. Assignment operators are often used when you want to make a variable change by a specific amount in shorthand.
In this blog post, we will discuss what the assignment operators are, how to use them in Python, and the benefits and drawbacks of doing so. We will also explore when it is best to use assignment operators in Python, and when it may not be the best option. Finally, we will answer the question of whether or not the incrementing operator ++
is available in Python.
What are the Assignment Operators and why would you use them in Python?
An assignment operator is a symbol that you use in Python to indicate that you want to perform some action and then assign the value to a . You would use an addition or subtraction operator in Python when you want to calculate the result of adding or subtracting two numbers. There are two types of addition operators: the plus sign +
and the asterisk *
. There are also two types of subtraction operators: the minus sign -
and the forward slash /
.
The plus sign +
is used to indicate that you want to add two numbers together. The asterisk *
is used to indicate that you want to multiply two numbers together. The minus sign -
is used to indicate that you want to subtract two numbers. The forward slash /
is used to indicate that you want to divide two numbers.
How do you use the Assignment Operators in Python and what are the benefits of doing so?
Here are some examples of how to use the addition and subtraction operators in Python:
>>> x = 25
>>> y = 15
>>> x += y # This line adds the value of y (15) to x (25), resulting in a value of 40 for x.
>>> print(x)
40
>>> x = 25
>>> y = 15
>>> x -= y # This line subtracts the value of y (15) to x (25), resulting in a value of 10 for x.
>>> print(x)
10
The +=
operator is used to add two numbers together. The result of the addition will be stored in the variable that is on the left side of the +=
symbol. In this example, the result of adding y
(15) to x
(25) is stored in the variable x
.
The -=
operator is used to subtract one number from another. The result of the subtraction will be stored in the variable that is on the left side of the -=
symbol. In this example, the result of subtracting y
(15) from x
(25) is stored in the variable x.
What are the benefits of using the assignment operators in Python?
These operators are convenient ways to add or subtract values from a variable without having to use an intermediate variable. For example, if you want to add two numbers together and store the result in a new variable, you would write:
first_number = first_number + second_number
However, if you want to use the +=
operator instead, you can simply write:
first_number += second_number
This will save you a few keystrokes and also make your code more readable.
The -=
operator is similarly useful for subtracting values from a variable. For example:
first_number -= second_number
This will subtract the second_number
from the variable first_number
.
What are the potential drawbacks of using the Assignment Operators in Python?
One potential drawback to using the +=
and -=
assignment operators in Python is that they can be confusing for beginners.
Another potential drawback is that they can lead to code that is difficult to read and understand.
However, these drawbacks are outweighed by the benefits of using these operators, which include increased readability and brevity of code. For these reasons, I recommend using the +=
, -=
, *=
, and /=
assignment operators in Python whenever possible.
When is it best to use the Assignment Operators in Python?
The increment operator is best used when you want to increment or decrement a variable by a number and you want to eliminate some keystrokes or create some brevity in your code.
In addition, it can also be used with other operators, such as the multiplication operator *
. The following example shows how to use the increment operator with the multiplication operator:
x = 1
x *= 12
print(x)
This will print out the value of x
as "120".
When is it not recommended to use the Assignments Operators in Python?
You may consider not using the assignment operators when you are dealing with a team of new programmers who may find the syntax confusing.
There are ways to mitigate this though, with training, lunch and learns, and more you can bring up the developers to the place where using some of the intricacies of Python.
Is there ++ in Python?
No, the ++
(and --
) operators available in C and other languages were not brought over to Python.
If you attempt to use ++
and --
the way they are used in C you will not get the results you are expecting. Use the +=
and -=
operators.
Why isn't there ++ and -- in Python?
Python doesn't have a ++
operator because it can be easily replaced with the +=
operator. Having both was probably thought to create more confusion.
There's a certain amount of ambiguity in the operator to the language parser. It could interpret the ++
in Python as two unary operators (+ and +) or it could be one unary operator (++).
Lastly, it can cause confusing side effects and Python likes to eliminate edge cases when interpreting the code. Look up C precedence issues of pre and post incrementation if you want to know more. It's not fun.
Summary
The +=
and -=
assignment operators in Python are convenient ways to add or subtract values from a variable without having to use an intermediate variable. They are also more readable than traditional methods of adding or subtracting values. For these reasons, I recommend using the += and -= assignment operators whenever possible. You can learn more in the Python documentation. Start using these powerful tools today!
Next Steps
If you're interested in learning more about the basics of coding, programming, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers