How to Fix: Positional Argument Follows Keyword Argument in Python
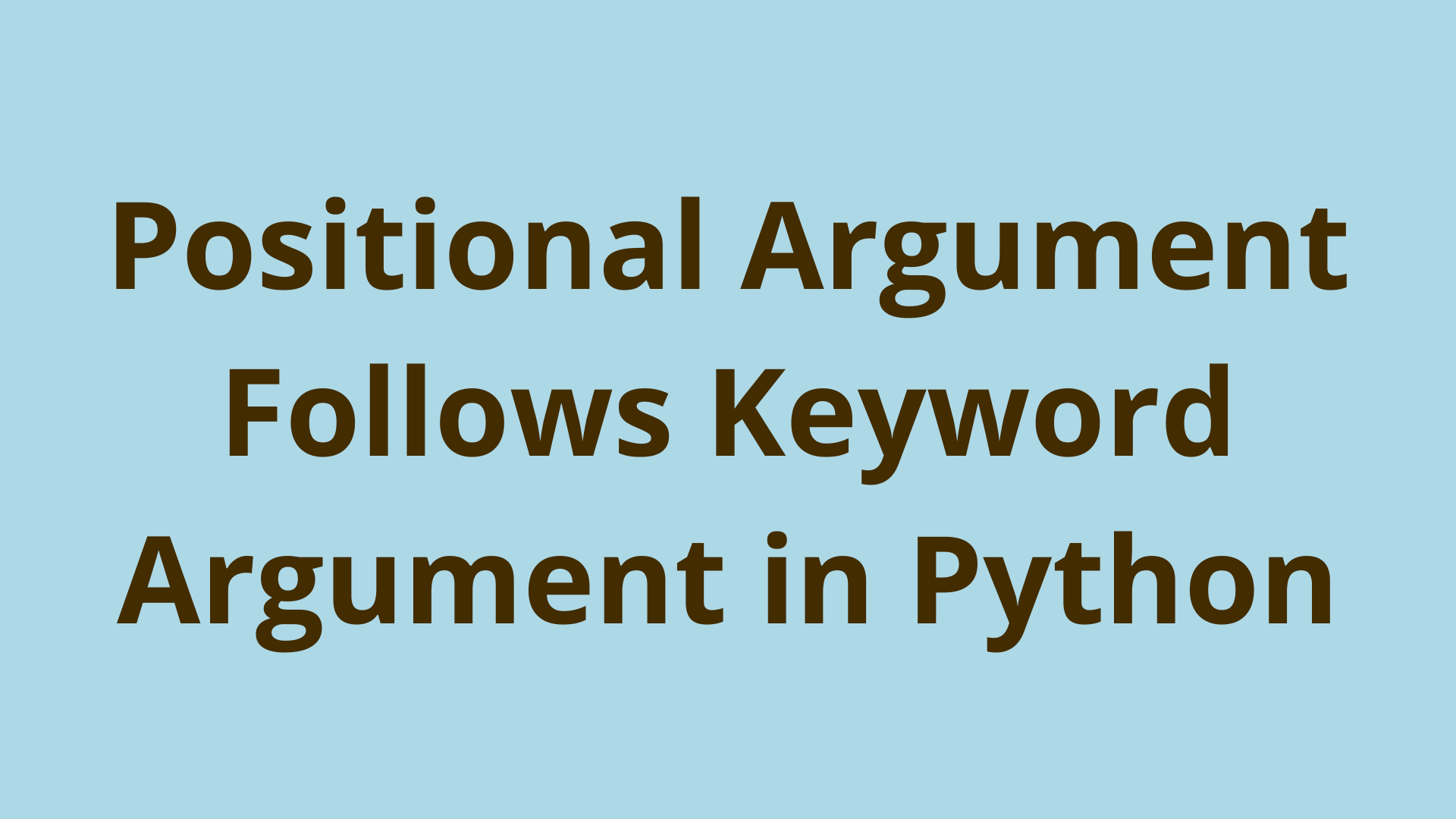
ADVERTISEMENT
Table of Contents
- Introduction
- Positional and Keyword Arguments in Python
- What is SyntaxError: positional argument follows keyword argument?
- How to Fix SyntaxError: positional argument follows keyword argument?
- Using Only Positional Arguments
- *args and **kwargs Parameters in Python
- Summary
- Next Steps
- References
Introduction
Arguments (or parameters) are a fundamental concept in Python programming. They allow you to provide inputs to your programs and functions that result in a varying output return value or varying set of steps performed by your program.
In Python, you might have encountered the error SyntaxError: Positional Argument Follows Keyword Argument
when working with positional and keywords arguments together in one function. To resolve this error you must understand how arguments fundamentally work in Python.
In this article, you will learn how to fix the "SyntaxError: Positional Argument Follows Keyword Argument" in Python by understanding what positional and keyword arguments are and how they work, which will help you prevent this error from occurring in the future.
Positional and Keyword Arguments in Python
Arguments allow developers and users to provide input values to a program or function that returns a varying output based on the arguments supplied. In Python, arguments are most commonly used when calling functions and class methods - a class method is just a function defined as a part of a class. If you are familiar with the Linux command line, you likely have already used arguments with some Linux commands.
The terms "parameter" and "argument" are often used interchangeably. As a refresher, let's quickly mention the difference these two terms:
- A parameter is a scoped variable defined in the function header.
- An argument is the value passed as input via a function call, which is mapped to a parameter.
Python has two important types of arguments, positional arguments and keyword arguments.
You are probably most familiar with positional arguments, since they are the default type in Python.
Positional arguments are simply an ordered list of inputs in a Python function call that correspond to the order of the parameters defined in the function header.
>>> def func(num1, num2):
... print(f"Num 1: {num1}, Num 2: {num2}")
...
>>> func(1, 2)
Num 1: 1, Num 2: 2
>>>
>>> func(2, 1)
Num 1: 2, Num 2: 1
In this example, we have defined a the function func
with parameters num1
, and num2
. When calling the function, the argument order matters. Python uses the position of the arguments to determine the parameter to map each input value to.
On the other hand, a keyword argument is supplied with both a parameter name AND its corresponding value.
>>> def func(num1, num2):
... print(f"Num 1: {num1}, Num 2: {num2}")
...
>>> func(num1=1, num2=2)
Num 1: 1, Num 2: 2
>>>
>>> func(num2=2, num1=1)
Num 1: 1, Num 2: 2
Here, you can see how keyword arguments are used, and how changing the order of the arguments doesn't matter because you specify exactly which parameter refers to which value.
What is SyntaxError: positional argument follows keyword argument?
The Python SyntaxError: positional argument follows keyword argument occurs when you try to specify a positional argument after a keyword argument in a function call.
>>> def func(num1, num2):
... print(f"Num 1: {num1}, Num 2: {num2}")
...
>>> func(num1=1, 2)
File "<stdin>", line 1
func(num1=1, 2)
^
SyntaxError: positional argument follows keyword argument
Here, the SyntaxError occurred because the keyword argument num1=1
is followed by the positional argument 2
.
The logic behind why the SyntaxError occurs is that in order for Python to consistently map all supplied argument values to named parameters, all positional arguments must be specified before any keyword arguments.
How to Fix SyntaxError: positional argument follows keyword argument?
There are multiple ways to fix the Python exception SyntaxError: positional argument follows keyword
and each method has its pros and cons.
Using Positional Arguments Followed by Keyword Arguments
One method is to simply do what the error states and specify all positional arguments before our keyword arguments!
>>> func(1, num2=2)
Num 1: 1, Num 2: 2
Here, we use the positional argument 1
followed by the keyword argument num2=2
which fixes the error. However, you might have noticed that in the previous example we used a keyword argument for the num1 parameter instead of the num2 parameter. If you want to use both positional and keyword arguments, all the positional arguments must come before the keyword arguments.
In Python, optional arguments can be implemented by specifying a default value for a parameter in the function header. This can be done using syntax like "def func(num1, num2=10):". This makes the num2 parameter totally optional. If it's not specified in the function call, num2 will assume the default value of 10.
This method of using arguments is great when working with functions that have some required arguments, which you can use as positional arguments, and any additional optional arguments can be keyword arguments. This makes it clear which of the arguments passed were optional and which were required. Many times there can be a large number of optional arguments, and it would be impractical to refer to them without using keyword arguments.
Using Only Positional Arguments
Another way to resolve this error is to exclusively use positional arguments in your function call:
>>> func(1, 2)
Num 1: 1, Num 2: 2
This can be great when you have a few arguments and you want to keep things simple. However, this could make the code harder to understand as other developers don't know what each argument means right away. They would have to look at the function definition or the function documentation. And it's even worse as the number of arguments increases. It is also impossible to use this method with optional arguments, without passing the default values again in the function call. This is a bit redundant and prone to errors when specifying default values repeatedly in the function calls.
Using Only Keyword Arguments
Finally, you can fix the error by using only keyword arguments:
>>> func(num1=1, num2=2)
Num 1: 1, Num 2: 2
>>>
>>> func(num2=2, num1=1)
Num 1: 1, Num 2: 2
Using this method, you can explicitly state which parameter equals which value, and the order of the arguments doesn't matter since Python knows which parameter name to map each value to. It also makes the code easier for other developers to understand since there is less ambiguity.
This method also easily works with optional arguments. The only downside of this method is that it can be pretty verbose, and you would need to know the name of every parameter when writing a function call.
*args and **kwargs Parameters in Python
Two more ways to define and pass arguments in Python are *args
and **kwargs
. Using the single asterisk *
or double asterisk **
syntax before a parameter name in a function header allows you to pass a varying number of non-keyword or keyword arguments in a function call. Note that *args
stands for "arguments" and **kwargs
stands for "keyword arguments".
*args accepts any number of positional arguments which are ingested as a tuple object, with each argument value being included as an element in the tuple. **kwargs accepts any number of keyword arguments which are ingested as a dictionary object. Each keyword argument name is included as a key in the dictionary, and each corresponding keyword argument value is included as a value in the dictionary.
For example:
>>> def f_args(*args):
... print(args)
...
>>> f_args(1, 2, 3)
(1, 2, 3)
>>>
>>> def f_kwargs(**kwargs):
... print(kwargs)
...
>>> f_kwargs(a=1, b=2, c=3)
{'a': 1, 'b': 2, 'c': 3}
Here, we have two functions f_args
and f_kwargs
, which define *args
and **kwargs
parameters, respectively. You can see that *args
stores a tuple of positional arguments in the variable args
, and **kwargs
stores a dictionary of keyword arguments in the variable kwargs
.
Note that the variable names args
and kwargs
can be replaced with any other variable name, only the single *
or double **
asterisk syntax is important to define how the arguments are ingested.
Here is another example usage of *args
:
>>> def my_sum(*nums):
... total = 0
... for num in nums:
... total += num
... return total
...
>>> my_sum(1, 2, 3)
6
>>>
>>> my_sum(2, 2, 2, 5)
11
>>>
>>> my_sum()
0
The my_sum
function accepts any amount of positional arguments (or no arguments at all), and returns the sum of those arguments.
You can also use both *args
and **kwargs
together:
>>> def f(*args, **kwargs):
... print(args, kwargs)
...
>>> f(1, 2, a=3)
(1, 2) {'a': 3}
Here, all the positional arguments are included in the tuple args
, and all the keyword arguments are included in the dictionary kwargs
. Note that all positional *
parameters must come before keyword **
parameters, or you'll get an invalid syntax error as below:
>>> def f(**kwargs, *args):
File "<stdin>", line 1
def f(**kwargs, *args):
^
SyntaxError: invalid syntax
>>>
Summary
In this article, you learned how to fix the Python error `SyntaxError: Positional Argument Follows Keyword Argument" using various methods and learned about the different types of arguments in Python.
First, you learned what arguments are and some terminology regarding arguments. Then you learned what positional arguments are and what keyword arguments are.
Next, you saw when the "SyntaxError: Positional Argument Follows Keyword Argument" occurs with an example, and why it occurs. Which was followed, with various methods to fix the error and the benefits and drawbacks of each method.
Additionally, you learned what the *args
and **kwargs
arguments are in Python and how to use them.
Next Steps
To learn more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Python args and kwargs: Demystifie - https://realpython.com/python-kwargs-and-args/
- Python Function Arguments - https://www.programiz.com/python-programming/function-argument
Final Notes
Recommended product: Coding Essentials Guidebook for Developers