An Intro to the Python HTTP Server
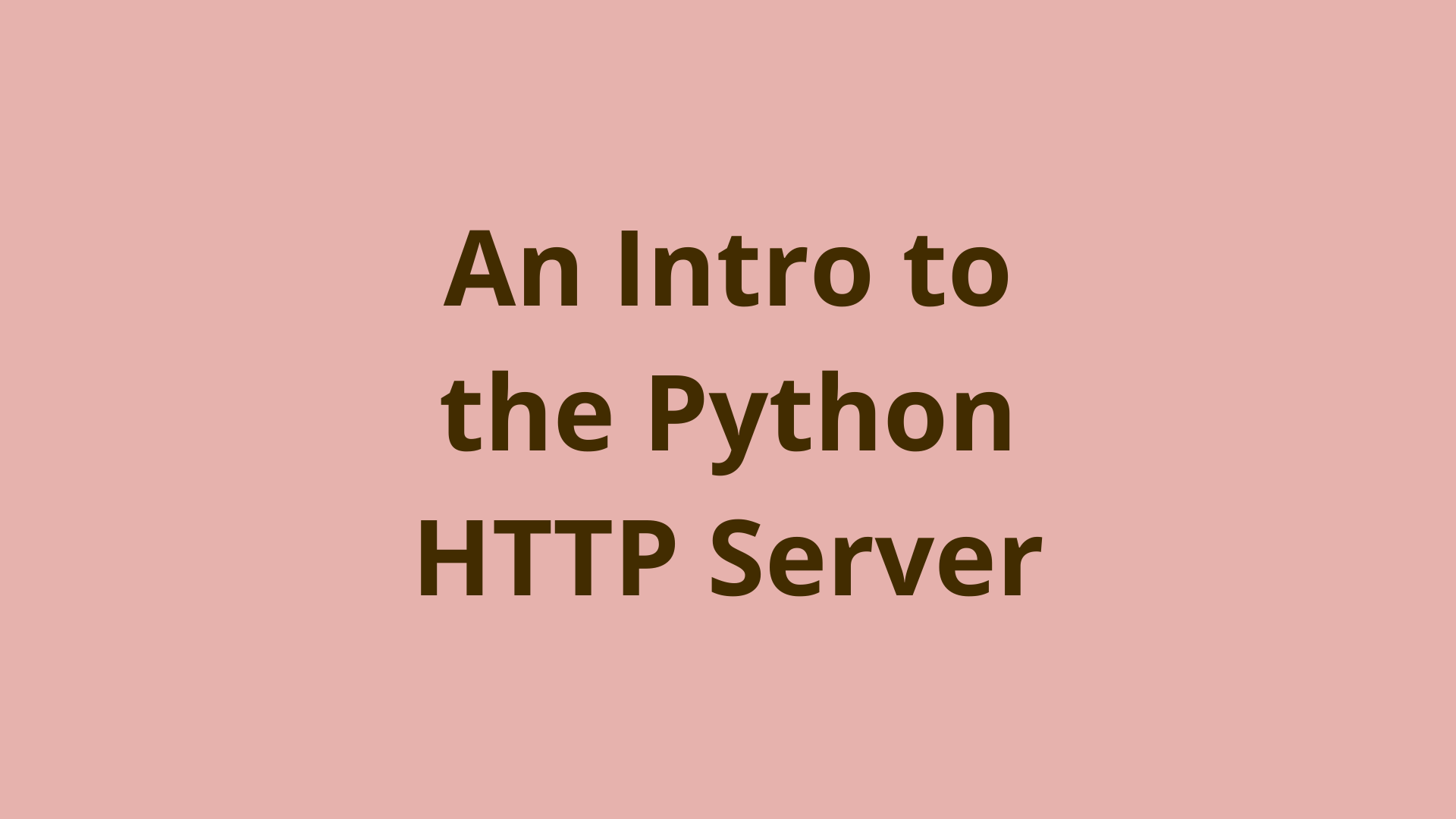
ADVERTISEMENT
Table of Contents
- Introduction
- Creating Python HTTP Server from the Command Line Terminal
- Accessing the Python HTTP Server Locally
- Access Python HTTP Server Over the Network
- Creating a Python HTTP Server via Script
- Customizing the Python HTTP Server with an index.html File
- Summary
- Next Steps
Introduction
Python's standard library includes a simple web server that supports web client-server communication. Although the http.server
module has limited security and should not be use in a production environment, it is useful for developmental purposes and local file sharing.
In this article, we'll discuss how the Python http server module can be used from the terminal, and then we'll cover how to use it within a Python script.
Creating Python HTTP Server from the Command Line Terminal
To launch a Python HTTP server from the command-line, first open the terminal and navigate to the directory that will be hosted to the server. In our example, we'll navigate to a folder we've created that contains hello-world.txt
and lorem-ipsum.txt
:
cd ~/projects/http-server
From this directory, we can run the command python -m http.server
to start a local HTTP server. By default, this will create a server at port 8000. We can also specify a port by running the command python -m http.server PORT_NUMBER
.
Accessing the Python HTTP Server Locally
Now that we've launched the server, we can access it on our local device. To access the server, open a browsing window and enter http://localhost:PORT_NUMBER
into the URL field. If a port number is not specified in the previous step, the server will be found at http://localhost:8000
.
The browser window will then show a list of files in the local directory:
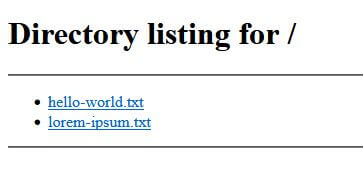
From here, users can open or download any of the hosted files.
Access Python HTTP Server Over the Network
Once the server is launched, users can also access the page from other devices that are connected to the same LAN or WLAN network. To access this server, we first need to get the IP address of the host device.
To do this, we can navigate to the terminal and enter either ipconfig
on a Windows device, or ifconfig
on a Linux, Unix, or macOS device. Once we've obtained the IP address of the host machine, we can then access the server on any device on the same network by simply opening a browser window and entering, http://IP_ADDRESS:8000/
. As on the host device, this page will display the list of files in the directory.
Creating a Python HTTP Server via Script
In addition to launching a server from the terminal, Python's http.server
module can be used to start a server using the following script:
import SimpleHTTPServer
import SocketServer
PORT = 8000
Handler = SimpleHTTPServer.SimpleHTTPRequestHandler
httpd = SocketServer.TCPServer(("", PORT), Handler)
httpd.serve_forever()
By default, this will start a server in the working directory, but a directory location can also be specified. As with the previous example, this server can be accessed on the host device by typing http://localhost:8000/
into the browser window or on other network devices by entering http://IP_ADDRESS:8000/
into a browser window.
Customizing the Python HTTP Server with an index.html File
The http.server
module is not limited to just hosting a list of files. We can also use this module to host a website based on a custom index.html
file. With this approach, the URL will show the contents of the index.html
file instead of the list of files in the host directory.
We'll create a simple index.html
file in the work directory that simply displays 'Hello World':
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello!</title>
</head>
<body>
<h1>Hello World!</h1>
<p>This is a simple paragraph.</p>
</body>
</html>
Then, we can run the following script to launch the server:
import http.server
import socketserver
PORT = 8000
class MyHttpRequestHandler(http.server.SimpleHTTPRequestHandler):
def do_GET(self):
self.path = 'index.html'
return http.server.SimpleHTTPRequestHandler.do_GET(self)
Handler = MyHttpRequestHandler
with socketserver.TCPServer(("", PORT), Handler) as httpd:
print("Http Server Serving at port", PORT)
httpd.serve_forever()
Now, when we navigate to http://localhost:8000/
, we see the custom HTML from our index.html
file instead of the list of files in our working directory:
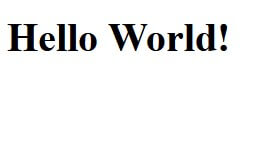
Summary
Python’s HTTP server makes it easy for developers to get started with web client-server communication either from the terminal or from a script. Although http.server
isn't secure for use in a production environment, it provides an easy way for developers to view a local web design or to share files across a private network. For people new to web development, http.server
is a user-friendly way to experiment with website designs.
Next Steps
If you're interested in learning more about the basics of coding, programming, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers